1. Write a program to play rock-paper-scissors. The program should let the user determine whether it is a 2-player game or if they want to play against the computer. If 2-player then each player types in either R, P, or S. If 1-player then only that player would type in either R, P, or S. The computer's choice should be determined randomly. The program announces a winner as well as the basis for determining the winner: Paper covers rock, Rock breaks scissors, Scissors cuts paper, or Nobody wins. Be sure to allow the users to use lowercase as well as uppercase letters. The program should validate that the user(s) did indeed type in a R, P, or S and if not then they should be allowed to re- enter. The program should include a loop that lets the user(s) play again until the users says they are done.
Hello, for this code I am having trouble with the statements to determine if paper beats rock, rock beats scissors, etc. I understand how to prompt the users for input but struggling with the statements.


from random import randint
#create a list of play options
t = ["Rock", "Paper", "Scissors"]
#assign a random play to the computer
computer = t[randint(0,2)]
#set player to False
player = False
while player == False:
#set player to True
player = input("Rock, Paper, Scissors?")
if player == computer:
print("Tie!")
elif player == "Rock":
if computer == "Paper":
print("You lose!", computer, "covers", player)
else:
print("You win!", player, "smashes", computer)
elif player == "Paper":
if computer == "Scissors":
print("You lose!", computer, "cut", player)
else:
print("You win!", player, "covers", computer)
elif player == "Scissors":
if computer == "Rock":
print("You lose...", computer, "smashes", player)
else:
print("You win!", player, "cut", computer)
else:
print("That's not a valid play. Check your spelling!")
#player was set to True, but we want it to be False so the loop continues
player = False
computer = t[randint(0,2)]
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

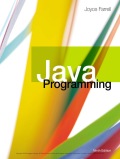
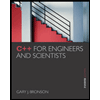
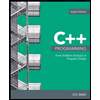
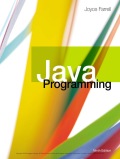
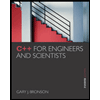
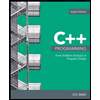