1. Your program must implement all the functions (load_contact_list and the helper functions) listed above. Your implemented functions must have the same name as the descriptions above, or you will lose points. 2. Each function you implement must follow the descriptions provided above.
1. Your program must implement all the functions (load_contact_list and the helper functions) listed above. Your implemented functions must have the same name as the descriptions above, or you will lose points. 2. Each function you implement must follow the descriptions provided above.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Implement the following functions:
- code should be in python
![The load_contact_list Function
You will first implement the load_contact_list function, which should take in one argument, the name of the file to load.
This function should read the contact list file that is passed in, store the contents into a dictionary of dictionaries, and
return that dictionary.
• The higher-level dictionary should contain the names as its keys.
• Each entry in the higher-level dictionary should contain 3 entries with the keys email, phone, and address. The values
for each of these dictionaries should be filled according the content loaded from the lists.
For example, consider the sample file containing the following few lines:
Sandy Cheeks, cheeks@ucsc.edu, 831-507-8282, 157 Dome Drive
Perry Platypus, notaplatypus@ucsc.edu, 510-339-2102, 7211 Tristate Alley
Ino Yamanaka, telekineticflower@ucsc.edu, 415-988-2341, 98 Konoha Lane
The following code should produce the following results (displayed output is italicized).
contact_list_dict = load_contact_list('sample_contact_list.txt')
print(contact_list_dict['Sandy Cheeks'])
{'email': 'cheeks@ucsc. edu', 'phone': '831-507-8282',
print(contact_list_dict['Ino Yamanaka']['email']
telekineticflower@ucsc. edu
'address': '157 Dome Drive'}
Keep in mind that content values must be trimmed of surrounding whitespaces.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F30f15bed-e8ae-4686-a633-a9ef864b3bed%2Fb9019017-e8d3-44d4-91b4-101e0603d30f%2Fux1vccej_processed.png&w=3840&q=75)
Transcribed Image Text:The load_contact_list Function
You will first implement the load_contact_list function, which should take in one argument, the name of the file to load.
This function should read the contact list file that is passed in, store the contents into a dictionary of dictionaries, and
return that dictionary.
• The higher-level dictionary should contain the names as its keys.
• Each entry in the higher-level dictionary should contain 3 entries with the keys email, phone, and address. The values
for each of these dictionaries should be filled according the content loaded from the lists.
For example, consider the sample file containing the following few lines:
Sandy Cheeks, cheeks@ucsc.edu, 831-507-8282, 157 Dome Drive
Perry Platypus, notaplatypus@ucsc.edu, 510-339-2102, 7211 Tristate Alley
Ino Yamanaka, telekineticflower@ucsc.edu, 415-988-2341, 98 Konoha Lane
The following code should produce the following results (displayed output is italicized).
contact_list_dict = load_contact_list('sample_contact_list.txt')
print(contact_list_dict['Sandy Cheeks'])
{'email': 'cheeks@ucsc. edu', 'phone': '831-507-8282',
print(contact_list_dict['Ino Yamanaka']['email']
telekineticflower@ucsc. edu
'address': '157 Dome Drive'}
Keep in mind that content values must be trimmed of surrounding whitespaces.

Transcribed Image Text:Helper Functions
You will also implement the following helper functions that use the dictionary returned from the load_contact_list
function you implement. The first argument of each of these functions will be the contact list dictionary. This is
necessary so these helper functions have the dictionary in their scope.
• get_email: The second argument is a name. This function returns the email of the specified contact. If contact is not
found, this function must print an error and return None.
• get_phone: The second argument is a name. This function returns the phone number for the specified contact. If
contact is not found, this function must print an error and return None.
get_address: The second argument is a name. This function returns the address for the specified contact. If contact
is not found, this function must print an error and return None.
get_email_list: The second argument is a list of names. This function returns a list of emails for all specified contacts.
If one of the contacts is not found, this function must print an error message (that includes the name of the contact
not found) and not include a value for that missing contact in the list that is returned.
• get_phone_list: The second argument is a list of names. This function returns a list of phone numbers for all
specified contacts. If one of the contacts is not found, this function must print an error message (that includes the
name of the contact not found) and not include a value for that missing contact in the list that is returned.
get_address_list: The second argument is a list of names. This function returns a list of addresses for all specified
contacts. If one of the contacts is not found, this function must print an error message (that includes the name of
the contact not found) and not include a value for that missing contact in the list that is returned.
Requirements
1. Your program must implement all the functions (load_contact_list and the helper functions) listed above. Your
implemented functions must have the same name as the descriptions above, or you will lose points.
2. Each function you implement must follow the descriptions provided above.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
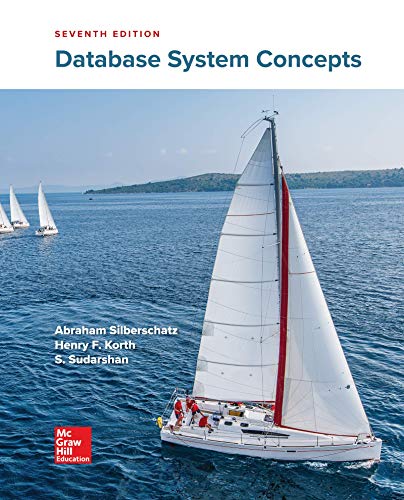
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
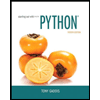
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
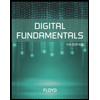
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
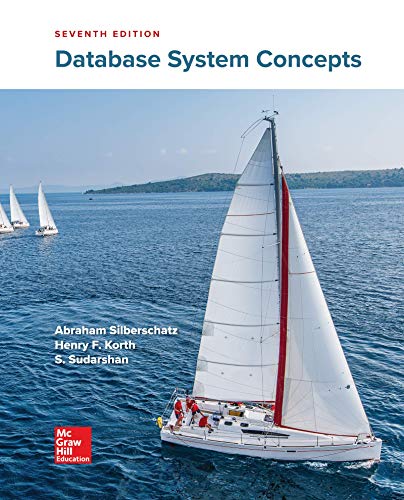
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
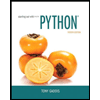
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
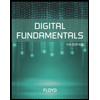
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
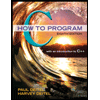
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
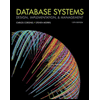
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
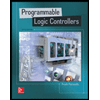
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education