15. Dice Game Write a program that uses the Die class that was presented in this chapter to play a simple dice game between the computer and the user. The program should create two instances of the Die class (each a 6-sided die). One Die object is the computer's die, and the other Die object is the user's die. The program should have a loop that iterates 10 times. Each time the loop iterates, it should roll both dice. The die with the highest value wins. (In case of a tie, there is no winner for that particular roll of the dice.) As the loop iterates, the program should keep count of the number of times the computer wins, and the number of times that the user wins. After the loop performs all of its iterations, the program should display who was the grand winner, the computer or the user.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:


//you haven't mentioned the language, I have done in java, if u need in other languages please //comment
public class Die {
//method return values from 1to6
int roll()
{
return (int)(Math.random()*6+1);
}
public static void main(String args[])
{
//part 1
Die comp=new Die();
Die user=new Die();
 Â
int comp_win=0,user_win=0,tie=0;
int comp_roll=0,user_roll=0;
//part 2
for(int i=0;i<10;i++)
{
comp_roll=comp.roll();
user_roll=user.roll();
if(comp_roll>user_roll)
comp_win++;
else if(comp_roll<user_roll)
user_win++;
else
tie++;
System.out.println("***At iteration"+(i+1));
System.out.println("Computer rolled:"+comp_roll);
System.out.println("User rolled:"+user_roll);
System.out.println("currently Computer score:"+comp_win+" User score"+user_win);
}
if(comp_win>user_win)
{ System.out.println("The Grand winner is Computer.");
System.out.println("Total Computer score:"+comp_win+" User score"+user_win+" no.of ties:"+tie);
}
else if(comp_win<user_win)
{ System.out.println("The Grand winner is User.");
System.out.println("Total Computer score:"+comp_win+" User score"+user_win+" no.of ties:"+tie);
}
else
{ System.out.println("Its overall Tie");
System.out.println("Total Computer score:"+comp_win+" User score"+user_win+" no.of ties:"+tie);
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

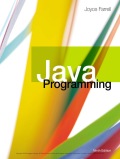
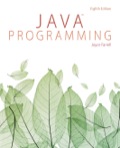
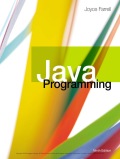
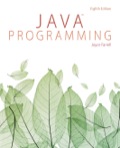