(5) def add_chars (some_list, some_str): Special Restrictions: You are not allowed to use loops. You must use recursion for this function. aracters of some_str to some_list in the following order and return the list: [1st element of some_list, 1st character of some_str, 2nd element of some list, 2nd character of some str, 3rd element of some_list, 3rd character of some_str, ........ .....] For example, if some_list-[1, 2, 3, 4, 5] and some_str = "hello", the returned list is [1, 'h', 2, 'e', 3, '1', 4, '1', 5, '0']. Note that, some_list and some_str can have unequal length. If length of some_str is less than length of some_list, after adding all the characters of the some_str, we leave the rest of the some_list as-is.
(5) def add_chars (some_list, some_str): Special Restrictions: You are not allowed to use loops. You must use recursion for this function. aracters of some_str to some_list in the following order and return the list: [1st element of some_list, 1st character of some_str, 2nd element of some list, 2nd character of some str, 3rd element of some_list, 3rd character of some_str, ........ .....] For example, if some_list-[1, 2, 3, 4, 5] and some_str = "hello", the returned list is [1, 'h', 2, 'e', 3, '1', 4, '1', 5, '0']. Note that, some_list and some_str can have unequal length. If length of some_str is less than length of some_list, after adding all the characters of the some_str, we leave the rest of the some_list as-is.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 12PE
Related questions
Question
5A in Python language please:
![(5) def add_chars (some_list, some_str):
Special Restrictions: You are not allowed to use loops. You must use recursion for this function.
aracters of some_str to some_list in the following order and return the list:
[1st element of some_list, 1st character of some_str, 2nd element of
some list, 2nd character of some_str, 3rd element of some_list, 3rd
character of some_str, .......
......]
For example, if some_list = [1, 2, 3, 4, 5] and some_str = "hello", the returned
list is [1, 'h', 2, 'e', 3, '1', 4, '1', 5, 'o'].
Note that, some_list and some_str can have unequal length.
• If length of some_str is less than length of some_list, after adding all the characters
of the some_str, we leave the rest of the some_list as-is.
For example, if some_list = [1, 2, 3, 4, 5] and some_str = "hel", the
returned list is [1, 'h', 2, 'e', 3, '1', 4, 5].
• If length of some_list is less than length of some_str, we do not add the extra
characters of some_str to the some_list.
For example, if some_list = [1, 2, 3] and some_str = "hello", the returned
list is [1, 'h', 2, 'e', 3, '1'].
Parameters: some_list (a list), some_str (a string)
Return value: A list as described above
Examples:
add_chars ([1, 2, 3, 4, 5], "hello")
→ [1, 'h', 2, 'e', 3, '1', 4, '1', 5, 'o']
add_chars ([1, 2, 3], "hello")
→ [1, 'h', 2, 'e', 3, '1']
add_chars ([1, 2, 3, 4, 5], "hel")
→ [1, 'h', 2, 'e', 3, '1', 4, 5]
add_chars ([], "hello")
→ []](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa2fae0d2-f8de-4d86-b278-3d843b434350%2F1f0909fe-6d88-44cd-871a-e226d02c59ad%2F30rdbwb_processed.jpeg&w=3840&q=75)
Transcribed Image Text:(5) def add_chars (some_list, some_str):
Special Restrictions: You are not allowed to use loops. You must use recursion for this function.
aracters of some_str to some_list in the following order and return the list:
[1st element of some_list, 1st character of some_str, 2nd element of
some list, 2nd character of some_str, 3rd element of some_list, 3rd
character of some_str, .......
......]
For example, if some_list = [1, 2, 3, 4, 5] and some_str = "hello", the returned
list is [1, 'h', 2, 'e', 3, '1', 4, '1', 5, 'o'].
Note that, some_list and some_str can have unequal length.
• If length of some_str is less than length of some_list, after adding all the characters
of the some_str, we leave the rest of the some_list as-is.
For example, if some_list = [1, 2, 3, 4, 5] and some_str = "hel", the
returned list is [1, 'h', 2, 'e', 3, '1', 4, 5].
• If length of some_list is less than length of some_str, we do not add the extra
characters of some_str to the some_list.
For example, if some_list = [1, 2, 3] and some_str = "hello", the returned
list is [1, 'h', 2, 'e', 3, '1'].
Parameters: some_list (a list), some_str (a string)
Return value: A list as described above
Examples:
add_chars ([1, 2, 3, 4, 5], "hello")
→ [1, 'h', 2, 'e', 3, '1', 4, '1', 5, 'o']
add_chars ([1, 2, 3], "hello")
→ [1, 'h', 2, 'e', 3, '1']
add_chars ([1, 2, 3, 4, 5], "hel")
→ [1, 'h', 2, 'e', 3, '1', 4, 5]
add_chars ([], "hello")
→ []

Transcribed Image Text:User Defined Functions and Recursion
Background
The purpose of this Programming is to practice writing function definitions, using functions inside of
other functions, and using recursion to solve complex problems.
Restrictions
Any function that violates the following:
• You are not allowed to import anything
•
You are not allowed to use the global keyword, nor should you have any global variables. (In
other words, do not create any variables outside of a function definition.
You are not allowed to use slicing (except for the 5th function add_chars)
•
No built-in function except round(), range(), and len() is allowed
• From list methods, you are allowed to use .append(), .insert(),.remove(), or del
•
From dictionary methods, you are allowed to use .keys(), .values(),.items()
Testing
In addition to the examples in the tester file, you should also devise your own test cases for your code.
Develop additional test cases, on your own, to make sure that you haven't missed anything (empty lists,
different sized lists, etc) and your code is correct. The goal is to keep your focus on writing correct code.
Functions
In this Programming, you will be writing and calling a handful of functions (five). From the description,
you will generate the signature, and function body for each function. (The examples can give you hints
about the signatures!)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
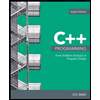
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
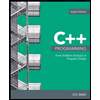
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning