a) Create a new Jupyter Notebook and start your program by asking the user for a text to encode and the shift value. Then begin the structure of your program by entering in this loop (we'll build on it more in a bit): encoded Text = *** for letter in text: encoded Text = encoded Text + letter What does this loop do? Make sure you understand what the code does before moving on!
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Q1: Secret Messages
Implement a Caesar cipher to encrypt messages.


Step by step
Solved in 4 steps with 9 images

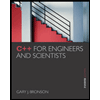
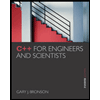