Add a method called multiplesOfFive to the Exercise class. The method must have a void return type and take a single int parameter called limit. In the body of the method, write a while loop that prints out all multiples of 5 between 10 and limit (inclusive) on a single line. For instance, if the value of limit were 15 then the method will
Add a method called multiplesOfFive to the Exercise class. The method must have a void return type and take a single int parameter called limit. In the body of the method, write a while loop that prints out all multiples of 5 between 10 and limit (inclusive) on a single line. For instance, if the value of limit were 15 then the method will
print: 10 15
You can use the printEvenNumbers method that is already in the Exercise class as an example to help you work out how to complete this method. In the main method of the Main class, add a call on the Exercise object to your multiplesOfFive method that prints the multiples of 5 between 10 and 25. Add a method called sum to the Exercise class. The method must have a void return type and take no parameters. In the body of the method, write a while loop to sum the values 1 to 10 and print the sum once the loop has finished. In the main method of the Main class, add a call on the Exercise object to your sum method. Check that it
prints: 55
Add a method called sum to the Exercise class. The method must have an int return type and take two int parameters called low and high, in that order. In the body of the method, write a while loop to add up all numbers between its two int parameters, inclusive. The method must return the total as its result. For instance: sum(1, 5) would return the total 15 (i.e., 1 + 2 + 3 + 4 + 5).
In the main method of the Main class, add calls on the Exercise object to your sum method with the following pairs of values for the low and high parameters:
- 1 for low and 5 for high
- 1 for low and 1 for high
- 2 for low and 3 for high
Print the value that is returned from each call. In the main method of the Main class, add a call on the Exercise object to your sum method with a value of 3 for the low parameter and 2 for the high parameter. Print the value that is returned. Have your method return 0 for cases like this where the value of low is greater than the value of high.
Add a new class to your IntelliJ project for this week's exercises and call it RandomTester. Import java.util.Random at the top of the class and define a field of type Random called rand. Initialize the field in the constructor by creating a Random object. This Random object will be the one to be used in the following exercises. Add the following statement to the main method. It won't produce any output, but it will be the starting point for the following exercises. RandomTester tester = new RandomTester();
In RandomTester, implement a method with the following header:
/**
* Print a single random integer using System.out.println.
*/
public void printOneRandom()
The method must use the Random object that was created in the constructor to generate a random integer, which it then prints using System.out.println. Add a statement to the main method to call printOneRandom on the tester object created in the previous exercise. Add a second method to RandomTester, with the following header:
/**
* Print multiple random integer values using System.out.println.
* @par howMany How many random values to print.
*/
public void printMultiRandom(int howMany)
This method must print the given number of random integers, one per line. This method must not create a new Random object. It must use the Random object that was created in the constructor and stored in the rand field.
Add a statement to the main method to call printMultiRandom on the tester object. Have the method print 5 random numbers.
Use the Java API documentation and find the version of the nextInt method in class Random that allows the target range of random numbers to be specified. What are all the possible random numbers that are generated when you call this method with 100 as its parameter?
Add a method to the RandomTester class with the following header:
/**
* Return a random integer in the range 0 to (limit-1) (inclusive).
* @par limit The limit of the range (exclusive).
* @return a random integer.
*/
public int getRandom(int limit)
Add two calls to the main method to your getRandom method. Pass in the value 10 to both calls and print out the two values that are returned.
Write a method in RandomTester with the following header:
/**
* Return a random one of the strings in the given list.
* The list will have at least one item in it.
* @par choices A list of strings.
* @return One of the strings in the given list.
*/
public String chooseOne(ArrayList<String> choices)

Step by step
Solved in 6 steps with 5 images

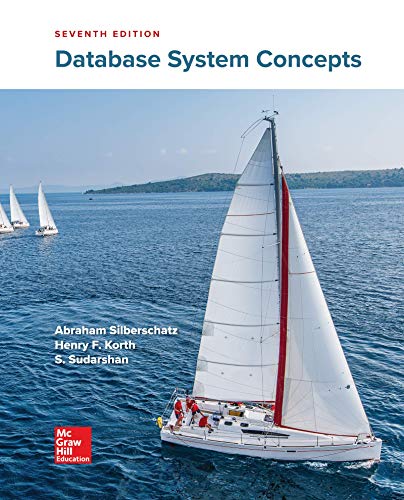
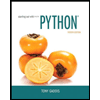
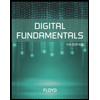
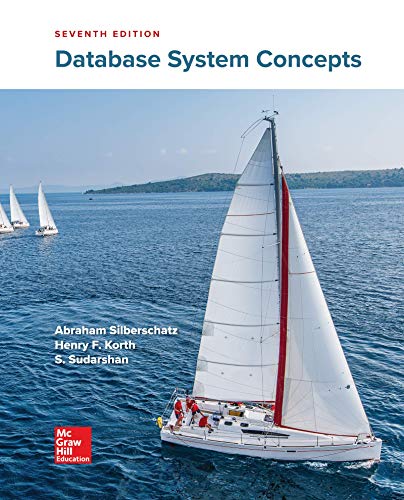
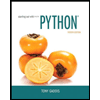
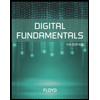
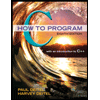
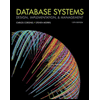
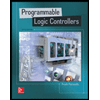