Add a public method called add() to the Java program below. This method adds another fraction to the ‘calling object’. Thus, the method will take a Fraction class object as a parameter, add this parameter fraction to the calling object (fraction), and return a Fraction object as a result. NOTE: it is possible to use cross multiplication to determine the numerator of the resultant Fraction. The denominator of the resultant Fraction is simply the multiplication of the denominators of the two other Fractions.
Add a public method called add() to the Java program below. This method
adds another fraction to the ‘calling object’. Thus, the method will take a
Fraction class object as a parameter, add this parameter fraction to the calling
object (fraction), and return a Fraction object as a result.
NOTE: it is possible to use cross multiplication to determine the numerator of the resultant Fraction. The denominator of the resultant Fraction is simply the multiplication of the denominators of the two other Fractions.
JAVA PROGRAM:
import java.util.ArrayList;
import java.util.Scanner;
//Define the class Fraction
class Fraction
{
private int n, d;
public Fraction()
{
this.n = this.d = 0; //Initialize the values
}
public Fraction(int n, int d)
{
this.n = n; //Initialize the variables
this.d = d;
}
//Define the getter function getNum() that returns the numerator
public int getNum()
{
//Returns numerator
return n;
}
//Define the getter function getDen() that returns the denominator
public int getDen()
{
//Returns denominator
return d;
}
//Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero
public boolean isZero()
{
return(getNum() == 0 && getDen() != 0);
}
public boolean isequals(Object c)
{
Fraction f = (Fraction) c;
return(Integer.compare(n,f.n))==0
&& (Integer.compare(d,f.d)==0);
}
//Define the function getSimplifiedFraction() that returns the simplified fraction
public String getSimplifiedFraction()
{
//Decalre the string variable result to store the result
String result = "";
//if the numerator and denominator both are zero
if(getNum() == 0 && getDen() == 0)
//result is zero
result = "0";
//if the numerator is zero and denominator is non-zero
else if(isZero())
//result is zero
result = "0";
//if the numerator is non-zero and denominator is zero
else if(getNum() != 0 && getDen() == 0)
//result is Undefined
result = "Undefined";
//for a defined and non-zero fraction
else
{
//if the remainder is zero
if(getNum() % getDen() == 0)
result = (getNum() / getDen()) + "";
//if the numerator and denominator both are greater than zero
else if(getNum() < 0 && getDen() < 0)
result = (getNum() * -1) + "/" + (getDen() * -1);
//if any of them is zero
else if(getNum() < 0 || getDen() < 0)
{
//if the numerator is greater than zero
if(getNum() < 0)
result = "-" + (getNum() * -1) + "/" + getDen(); // appending a sign.
//if the denominator is zero
else
result = "-" + getNum() + "/" + (getDen() * -1); // appending a sign.
}
//both are non-zero
else
result = (getNum() + "/" + getDen());
}
//return the result
return result;
}
//Define the method display() that displays the resultant fraction
public void display()
{
//Call and display the fraction
System.out.println(getSimplifiedFraction());
}
}
//Define the class TestFraction
class Main
{
//Define the main method
public static void main(String[] args)
{
//Declare the variables to store the numerator and denominator
String res;
int num, den, num1, den1;
//Declare an ArrayList to store the results
ArrayList<Fraction> fractions = new ArrayList<>();
//Create the object for scanner class
Scanner sc = new Scanner(System.in);
//use the do-while loop to iterate test the conditions
do
{
//Prompts the user to enter the numerator
System.out.print("Enter the num: ");
//Store the Integer part only
num = Integer.parseInt(sc.nextLine().trim());
//If the numerator is less than zero
if(num < 0)
//Break from loop
break;
//Prompts the user to enter the denominator
System.out.print("Enter the den: ");
//store the integer part only
den = Integer.parseInt(sc.nextLine().trim());
//Create the object of Fraction class
Fraction fraction = new Fraction(num, den);
System.out.print("Enter the num to compare: ");
num1 = Integer.parseInt(sc.nextLine().trim());
if(num1 < 0)
break;
System.out.print("Enter the den to compare: ");
den1 = Integer.parseInt(sc.nextLine().trim());
Fraction fraction1 = new Fraction(num1, den1);
if(fraction1.isequals(fraction))
{
System.out.println("\nTrue-> Second fraction is equal to First Fraction");
}
else
System.out.println("\nFalse-> Second fraction is not equal to First Fraction"); // if isequal() returns zero.
//Add the resultant fractions into the ArrayList
fractions.add(fraction);
//Display the resultant fraction
res = fraction.getSimplifiedFraction();
System.out.println("Fraction added to list as: " + res + "\n"); //result is stored in ‘res’ variable
}while(res != "0");
//Display all fractions that are stored in the ArrayList
System.out.println("\nDISPLAYING ALL FRACTIONS:\n"
+ "-------------------------");
//Use the loop to display the values stored in the ArrayList
for(Fraction fr : fractions)
fr.display();
//to print the new line
System.out.println();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

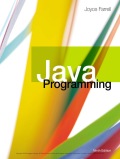
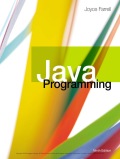