Assignment 7 B: Battle! Language is in C++ (pictures include the sample output) Recreate a basic battle system from a video game. You will create a character and then use it to battle a computer generated opponent. There are three possible classes, with the following attributes: Sword Fighter Dance Battler HP: 120 Attack: 40 Defense: 0.20 ClassID: 1 Unicorn Sorcerer HP: 80 Attack: 35 Defense: 0.60
Assignment 7 B: Battle! Language is in C++ (pictures include the sample output)
Recreate a basic battle system from a video game. You will create a character and then use it to
battle a computer generated opponent. There are three possible classes, with the
following attributes:
Sword Fighter Dance Battler
HP: 120
Attack: 40
Defense: 0.20
ClassID: 1
Unicorn Sorcerer
HP: 80
Attack: 35
Defense: 0.60
ClassID: 2
Dance Battler
HP: 100
Attack: 20
Defense: 0.42
ClassID: 3
You will create a NPC class that has these three attribute. It should also have an
attribute for Name.
You will then create the following methods in the NPC class:
A constructor that takes in a Name string and a ClassID int. It should use the
value of the ClassID to assign values to the other attributes (using the chart
above)
Getter methods for all five attributes, and a Setter method for HP that subtracts
the object’s HP by the value passed in.
calculateAttack
◦ This method returns a float value. It takes in a float that represents the
opponent’s defense percentage. It should multiply the object’s attack by the
inverse of the opponent’s defense percentage, and return that value. For
example:
100 * (1 – 0.60) = 40
calculateDefense
◦ This method returns nothing. It takes in a float that represents the opponent’s
attack power. It should multiply the opponent’s attack by the inverse of the
object’s defense percentage, then subtract 6 additional points from the result.
This value should then be subtracted from the object’s HP.
isStillAlive
◦ This method returns a boolean value. It should check if the object’s HP is less
than or equal to 0. If it is, then return FALSE. Otherwise, return TRUE.
You may add other “helper” methods to reduce redundant code, although this is
not required.
You should then create a driver class, Assignment7B. This program should do the
following:
Prompt the user for a custom name and what class they want their character to
be. You should use this information to create an NPC object.
Randomly generate another NPC object to represent the opponent. You can do
this by randomly generating the two primitive values, then using them to
construct the opponent NPC.
Create a loop that runs until one of the opponents runs out of HP. You will first
prompt the player to either attack or defend. Then, you will have the opponent
randomly choose to either attack or defend.
◦ If both the human and computer player choose to defend, do nothing.
◦ If both of them attack, then call the calculateAttack function on both objects.
◦ If one attacks and one defends, call the calculateDefense function on the
defending object.
After both choices are made, display messages indicating the actions take and
the remaining HP for both sides.
When one or both players are defeated, stop the loop and print the results
Sample Output:
[Generic RPG Battle System]
Enter your name: Morgan
Enter your battle class: Unicorn Sorcerer
Morgan the Unicorn Sorcerer, your opponent is Brad the Battle Dancer!
-Round 1-
Do you (a)ttack or (d)efend? a
Morgan the Unicorn Sorcerer attacked Brad the Battle Dancer!
Brad now has 79.7 HP.
Brad the Battle Dancer attacked Morgan the Unicorn Sorcerer!
Morgan now has 72 HP.
-Round 2-
Do you (a)ttack or (d)efend? d
Morgan the Unicorn Sorcerer is on guard.
Brad the Battle Dancer is on guard.
-Round 3-
Do you (a)ttack or (d)efend? d
Morgan the Unicorn Sorcerer is on guard.
Brad the Battle Dancer attacked Morgan the Unicorn Sorcerer!
Morgan now has 70 HP.
//Skipping ahead to the end of this epic battle (THIS IS NOT PART OF
//THE OUTPUT
Brad the Battle Dancer was defeated...
Morgan the Unicorn Sorcerer wins!

Step by step
Solved in 2 steps with 5 images

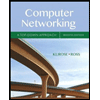
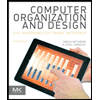
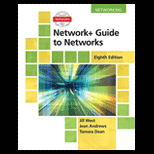
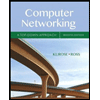
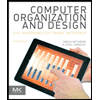
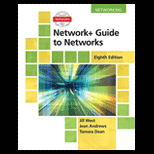
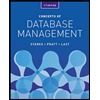
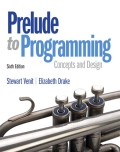
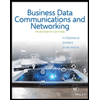