ate class methods (Setters and Getters) that load the data values. The Setter methods will validate the input data based on the criteria below and load the data into the class if valid. For strings, the data must be within the specified data size. (See #3 below) For zipCode, the number must be between 0 and 99999. City and State need to be converted and stored in uppercase. 3. Write a program that will create an instance of this
C++
Note: C-Strings char arrays should be now built as "string". This will help to simplify your work.
2. Create class methods (Setters and Getters) that load the data values. The Setter methods will validate the input data based on the criteria below and load the data into the class if valid.
For strings, the data must be within the specified data size. (See #3 below)
For zipCode, the number must be between 0 and 99999.
City and State need to be converted and stored in uppercase.
3. Write a program that will create an instance of this class as an Object. Allow me to enter data for the Object and create and call a method that displays the data for the Object.
During the input process, pass the input data to the setter method and confirm that the data entered is valid based on the class method return value. If not valid, prompt for me to re-enter the data until it does pass the class method's validation.(A do-while loop works well for this situation) I only need to enter the data for 1 instance of the class. The best way to do this is for the setter methods to return bool instead of void. If the data is valid, return true. Otherwise return false.
There is no need to loop for multiple instances of the object. Do not use cin statements inside the class method definitions.
Below is the original structure to use to create your class definition.
const int NAME_SIZE = 20;
const int STREET_SIZE = 30;
const int CITY_SIZE = 20;
const int STATE_CODE_SIZE = 3;
struct Customer {
long customerNumber;
char name[NAME_SIZE]; // change to string name;
char streetAddress_1[STREET_SIZE]; // change to string
char streetAddress_2[STREET_SIZE]; // change to string
char city[CITY_SIZE]; // change to string
char state[STATE_CODE_SIZE]; // change to string
int zipCode;};
Hints: The const variables need to be defined outside of the class definition.
All access to the data in the class must use public methods that you define. DO NOT DIRECTLY ACCESS the customer data items.
The class level input methods should return a boolean to confirm the data was loaded and passed any size or data ranges. The customerNumber setter does not need to return a value - It can be a void setter.
Set the customerNumber to 1 via the code - not user input. There is no input loop so only 1 set of customer's data is to be entered.
Work on 1 task/data item at a time. Create a getter/setter for customer number, set it, display it, then move to name, etc. If you get stuck, ask for help.
The upper casing of the City and State involve using a loop and treating the string as an array - use toupper to convert each character.
i.e.
FOR loop - start at 0 - while index < string.length()
street_1[idx] = toupper ( street_1[idx]);
Avoid cout statements in the class definition - as in for bad data. A class typically does not contain IO related code unless that is its purpose.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

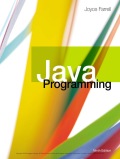
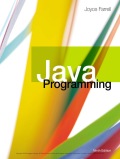