Below is my code and an image of the prompt. The code runs but according to my professor I cant use built in functions such as in Line 4[clientList.append(fullName)], Line 17[clientList.insert(index, fullName)], & Line 46[combined_data.sort(reverse=True)]. I also cannot use these other functions such as Sort,Max,Min,Append,Insert & Remove.
Below is my code and an image of the prompt. The code runs but according to my professor I cant use built in functions such as in Line 4[clientList.append(fullName)], Line 17[clientList.insert(index, fullName)], & Line 46[combined_data.sort(reverse=True)]. I also cannot use these other functions such as Sort,Max,Min,Append,Insert & Remove.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Below is my code and an image of the prompt.
The code runs but according to my professor I cant use built in functions such as in
Line 4[clientList.append(fullName)],
Line 17[clientList.insert(index, fullName)],
& Line 46[combined_data.sort(reverse=True)].
I also cannot use these other functions such as Sort,Max,Min,Append,Insert & Remove.
Im suppose to replace the built in function and create my own functions but im not sure how and hopefully someone can solve it for me.
My Code(Python)
import sys
def addClient(clientList, balanceList, fullName, balance):
clientList.append(fullName)
balanceList.append(balance)
def removeClient(clientList, balanceList, fullName):
if fullName in clientList:
index = clientList.index(fullName)
clientList.pop(index)
balanceList.pop(index)
else:
print("Client not found.")
def insertClient(clientList, balanceList, fullName, index, balance):
if index >= 0 and index <= len(clientList):
clientList.insert(index, fullName)
balanceList.insert(index, balance)
else:
print("Invalid index. Index should be between 0 and", len(clientList))
def deposit(balanceList, index, depositAmount):
if index >= 0 and index < len(balanceList):
balanceList[index] += depositAmount
else:
print("Invalid index. Index should be between 0 and", len(balanceList) - 1)
def withdraw(balanceList, index, withdrawAmount):
if index >= 0 and index < len(balanceList):
if balanceList[index] >= withdrawAmount:
balanceList[index] -= withdrawAmount
else:
print("Insufficient balance.")
else:
print("Invalid index. Index should be between 0 and", len(balanceList) - 1)
def searchFirstName(clientList, firstName):
matching_indices = [i for i, name in enumerate(clientList) if firstName in name]
if matching_indices:
return matching_indices
else:
return None
def sortingDescending(clientList, balanceList):
combined_data = list(zip(clientList, balanceList))
combined_data.sort(reverse=True)
clientList, balanceList = zip(*combined_data)
def displayList(clientList, balanceList):
for i in range(len(clientList)):
print(f"{clientList[i]} - Balance: ${balanceList[i]:.2f}")
def exit_program():
print("Exiting the program.")
sys.exit()
clientList = ["Mike Navarro", "Miguel Saba", "Maria Rami"]
balanceList = [900.00, 400.00, 450.00]
while True:
print("\nMenu:")
print("1- Add a new client")
print("2- Remove a client")
print("3- Insert an item to the list")
print("4- Deposit")
print("5- Withdraw")
print("6- Search for a client based on firstName")
print("7- Sort the list in descending order")
print("8- Display the list")
print("9- Quit the program")
choice = input("Enter your choice: ")
if choice == '1':
fullName = input("Enter full name: ")
balance = float(input("Enter initial balance: "))
addClient(clientList, balanceList, fullName, balance)
elif choice == '2':
fullName = input("Enter the full name of the client to remove: ")
removeClient(clientList, balanceList, fullName)
elif choice == '3':
fullName = input("Enter full name: ")
index = int(input("Enter index: "))
balance = float(input("Enter balance: "))
insertClient(clientList, balanceList, fullName, index, balance)
elif choice == '4':
index = int(input("Enter index: "))
depositAmount = float(input("Enter deposit amount: "))
deposit(balanceList, index, depositAmount)
elif choice == '5':
index = int(input("Enter index: "))
withdrawAmount = float(input("Enter withdrawal amount: "))
withdraw(balanceList, index, withdrawAmount)
elif choice == '6':
firstName = input("Enter first name to search: ")
matching_indices = searchFirstName(clientList, firstName)
if matching_indices:
for index in matching_indices:
print(f"Found at index {index}: {clientList[index]} - Balance: ${balanceList[index]:.2f}")
else:
print("Client not found.")
elif choice == '7':
sortingDescending(clientList, balanceList)
elif choice == '8':
displayList(clientList, balanceList)
elif choice == '9':
exit_program()
else:
print("Invalid choice. Please select a valid option.")
![For this program, you have to write your own functions without using any of the build-in functions. You could only use len(), and index() for this program as built in functions for list.
You have to write your own functions for append(), insert(), remove(), max(), min(), sort().
Create a menu to allow for the user to add a client, insert the client into a special location in the list, closing the account(remove), Deposit, withdrawal, Search for a client using the
firstName, and Sorting in descending order. Declare and initialize your list as
clientList=["Mike navarro","Miguel saba","Maria Rami"] balanceList= [900.00, 400.00, 450.00]
You may only use these build in functions len(), index(). Here is an sample output:
1- Add a new client ->addClient(fullName, balance)
2- Remove a client -->removeClient(fullName)
3- Insert an item to the list-->insertClient(fullName, index,balance)
4- Deposit -->deposit(depositAmount, index)
5- Withdraw--> withdraw(withdrawAmount, index)
6-Search for the client based on firstName-->searchFirstName(firstName)
7- Sort the list in descending order based on firstName--> sortingDescending( clientList, balanceList)
8- Display the list.... displayList(clientList, balanceList)
9-Quit the program.....exit()
Once the user enters an option, your program should execute the code for that option and displays the list before and after the operations. Make sure to use a while loop so the program
runs until user enters the quit option.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb2f5b0ad-df1d-48c6-a9a3-5338b8167143%2F477f4532-3221-4b45-aa20-9d96a6172180%2Ff83mz7c_processed.png&w=3840&q=75)
Transcribed Image Text:For this program, you have to write your own functions without using any of the build-in functions. You could only use len(), and index() for this program as built in functions for list.
You have to write your own functions for append(), insert(), remove(), max(), min(), sort().
Create a menu to allow for the user to add a client, insert the client into a special location in the list, closing the account(remove), Deposit, withdrawal, Search for a client using the
firstName, and Sorting in descending order. Declare and initialize your list as
clientList=["Mike navarro","Miguel saba","Maria Rami"] balanceList= [900.00, 400.00, 450.00]
You may only use these build in functions len(), index(). Here is an sample output:
1- Add a new client ->addClient(fullName, balance)
2- Remove a client -->removeClient(fullName)
3- Insert an item to the list-->insertClient(fullName, index,balance)
4- Deposit -->deposit(depositAmount, index)
5- Withdraw--> withdraw(withdrawAmount, index)
6-Search for the client based on firstName-->searchFirstName(firstName)
7- Sort the list in descending order based on firstName--> sortingDescending( clientList, balanceList)
8- Display the list.... displayList(clientList, balanceList)
9-Quit the program.....exit()
Once the user enters an option, your program should execute the code for that option and displays the list before and after the operations. Make sure to use a while loop so the program
runs until user enters the quit option.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
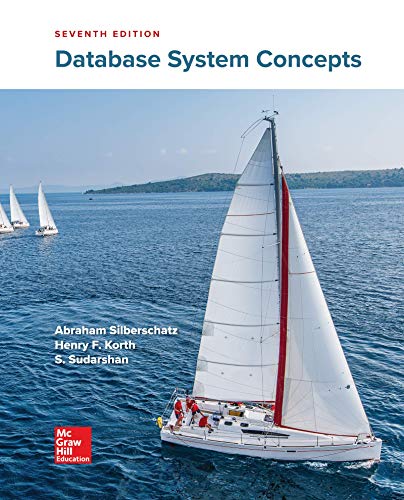
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
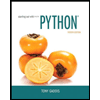
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
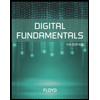
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
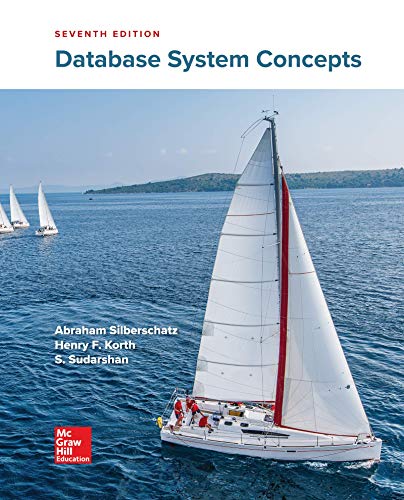
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
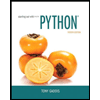
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
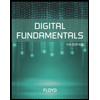
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
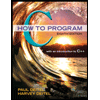
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
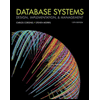
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
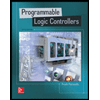
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education