Can someone please help me with the main code? The code for the business class is down below. Part I - Design a class named Business that contains: A String attribute named name for the Business name A String attribute named symbol for the Business’s stock symbol A double attribute named lastPrice that stores the stock price one month ago A double attribute named currentPrice that stores the stock price for the current time A default constructor An overloaded constructor that creates a Business with a specified Business stock symbol and name An overloaded constructor with all attributes Accessors and mutators for each attribute A copy Constructor An Equals Method A toString() method to display current state of the object, formatted! Part 2- Create a BusinessDriver application. You will have at least 4 methods in this application main() – setBusinessData() - this reads the data from the file (call the file businessData.txt) displayBusinessData() - this displays the data to the screen, calling the toString() method of all objects sortData() - This will sort the data by choice either name of stock or current price. Pick one. Create an arraylist of the 10 Business objects Create a menu: Options 1 = List the objects in the arraylist 2 = List just the Business names of the array list 3 = List just the Business Name and current stock price 4 = Sort by name or by price Your choice 5 = Exit Read the data for the array from a file (see below) Call each method as described above then list the menu and loop until the user exits. BusinessData.txt (the data is set up like this: Business symbol, Business name, lastprice, current price - copy and paste this into a .txt file) GPRO GoPro, Inc. 10.71 13.69 SBUX Starbucks 59.53 58.46 JCP JC Penney 7.73 10.78 AMZN Amazon 531.07 576.32 AE Adams Resources and Energy 34.96 33.77 KO Coca-Cola 42.72 43.60 MCD McDonald's 121.47 115.99 TSLA Tesla Motors 173.48 187.90 AAPL Apple Inc 96.35 100.67 FB Facebook 112.69 108.93 ------------------------------------CLASS CODE------------------------- public class Business { private String businessSymbol; private String businessName; private double lastPrice; private double currentPrice; public Business() { businessSymbol = " "; businessName = " "; lastPrice = 0; currentPrice = 0; } public Business(String businessName, String businessSymbol) { this.businessSymbol = businessSymbol; this.businessName = businessName; } public Business(String businessName, String businessSymbol, double lastPrice, double currentPrice) { this.businessSymbol = businessSymbol; this.businessName = businessName; this.lastPrice = lastPrice; this.currentPrice = currentPrice; } // The copy constructor public Business(Business business) { this.businessSymbol = business.businessSymbol; this.businessName = business.businessName; this.lastPrice = business.lastPrice; this.currentPrice = business.currentPrice; } public void setBussinessName(String businessName) { this.businessName = businessName; } public String getBussinessName() { return businessName; } public void setLastPrice(double lastPrice) { this.lastPrice = lastPrice; } public double getLastPrice() { return lastPrice; } public void setCurrentPrice(double currentPrice) { this.currentPrice = currentPrice; } public double getCurrentPrice() { return currentPrice; } public boolean equals(Business someBusiness) { boolean same = false; if(businessSymbol.equalsIgnoreCase(someBusiness.businessSymbol) && businessName.equalsIgnoreCase(someBusiness.businessName) && lastPrice == (someBusiness.lastPrice) && currentPrice ==(someBusiness.currentPrice)) same = true; return same; } public String toString() { String str = " "; return "\nBusiness symbol: " + businessSymbol + "\nBusiness name: " + businessName + "\nLast price: " + lastPrice + "\nCurrent price: " + currentPrice; } }//end class
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Can someone please help me with the main code? The code for the business class is down below.
Part I - Design a class named Business that contains:
- A String attribute named name for the Business name
- A String attribute named symbol for the Business’s stock symbol
- A double attribute named lastPrice that stores the stock price one month ago
- A double attribute named currentPrice that stores the stock price for the current time
- A default constructor
- An overloaded constructor that creates a Business with a specified Business stock symbol and name
- An overloaded constructor with all attributes
- Accessors and mutators for each attribute
- A copy Constructor
- An Equals Method
- A toString() method to display current state of the object, formatted!
Part 2- Create a BusinessDriver application.
- You will have at least 4 methods in this application
- main() –
- setBusinessData() - this reads the data from the file (call the file businessData.txt)
- displayBusinessData() - this displays the data to the screen, calling the toString() method of all objects
- sortData() - This will sort the data by choice either name of stock or current price. Pick one.
- Create an arraylist of the 10 Business objects
- Create a menu:
- Options
- 1 = List the objects in the arraylist
- 2 = List just the Business names of the array list
- 3 = List just the Business Name and current stock price
- 4 = Sort by name or by price Your choice
- 5 = Exit
- Read the data for the array from a file (see below)
- Call each method as described above then list the menu and loop until the user exits.
BusinessData.txt (the data is set up like this: Business symbol, Business name, lastprice, current price - copy and paste this into a .txt file)
GPRO
GoPro, Inc.
10.71
13.69
SBUX
Starbucks
59.53
58.46
JCP
JC Penney
7.73
10.78
AMZN
Amazon
531.07
576.32
AE
Adams Resources and Energy
34.96
33.77
KO
Coca-Cola
42.72
43.60
MCD
McDonald's
121.47
115.99
TSLA
Tesla Motors
173.48
187.90
AAPL
Apple Inc
96.35
100.67
FB
Facebook
112.69
108.93
------------------------------------CLASS CODE-------------------------
public class Business
{
private String businessSymbol;
private String businessName;
private double lastPrice;
private double currentPrice;
public Business()
{
businessSymbol = " ";
businessName = " ";
lastPrice = 0;
currentPrice = 0;
}
public Business(String businessName, String businessSymbol)
{
this.businessSymbol = businessSymbol;
this.businessName = businessName;
}
public Business(String businessName, String businessSymbol, double lastPrice, double currentPrice)
{
this.businessSymbol = businessSymbol;
this.businessName = businessName;
this.lastPrice = lastPrice;
this.currentPrice = currentPrice;
}
// The copy constructor
public Business(Business business)
{
this.businessSymbol = business.businessSymbol;
this.businessName = business.businessName;
this.lastPrice = business.lastPrice;
this.currentPrice = business.currentPrice;
}
public void setBussinessName(String businessName)
{
this.businessName = businessName;
}
public String getBussinessName()
{
return businessName;
}
public void setLastPrice(double lastPrice)
{
this.lastPrice = lastPrice;
}
public double getLastPrice()
{
return lastPrice;
}
public void setCurrentPrice(double currentPrice)
{
this.currentPrice = currentPrice;
}
public double getCurrentPrice()
{
return currentPrice;
}
public boolean equals(Business someBusiness)
{
boolean same = false;
if(businessSymbol.equalsIgnoreCase(someBusiness.businessSymbol) &&
businessName.equalsIgnoreCase(someBusiness.businessName) &&
lastPrice == (someBusiness.lastPrice) &&
currentPrice ==(someBusiness.currentPrice))
same = true;
return same;
}
public String toString()
{
String str = " ";
return "\nBusiness symbol: " + businessSymbol + "\nBusiness name: " + businessName + "\nLast price: " + lastPrice + "\nCurrent price: " + currentPrice;
}
}//end class

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

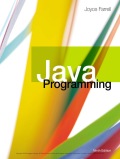
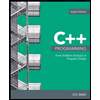
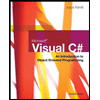
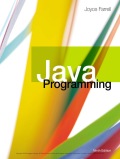
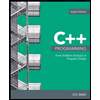
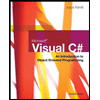