Can this code be modified so there are three sorted files merged instead of two. ( To compile: javac xxxxxH2.java ) ( To execute: java xxxxxH2 f1.txt f2.txt f3.txt f4.txt ) import java.util.*; import java.io.*; public class xxxxxH1{ // print k integers per line int k = 20; //use a short word for System.out to save typing PrintStream prt = System.out; // print file contents private void prtfile(String fn){ //declare variables int i=0, x; prt.printf("\n%s:", fn); try{ Scanner inf = new Scanner(new File(fn)); while (inf.hasNext()) { //read an input from inf x = inf.nextInt(); prt.printf("%3d ", x); i++; if(i % k == 0) prt.printf("\n%s:", fn); } // endwhile prt.printf("(count=%d)", i); // close file inf.close(); } catch (Exception e){ prt.printf("\nOoops! Read Error:");} } // end prtfile // complete following method private void merge(String fn1, String fn2, String fn3){ int x, y; try{ // merge two sorted integer files fn1 and fn1 into fn3 prtfile(fn1); // print first sorted file prtfile(fn2); // print second sorted file prt.printf("\n\tS T A R T * M E R G I N G\n"); // open input files F1 & F2 Scanner F1 = new Scanner(new File(fn1)); Scanner F2 = new Scanner(new File(fn2)); // open output file F3 PrintWriter F3 = new PrintWriter(new File(fn3)); // read an input from file F1 x = F1.nextInt(); // read an input from file F2 y = F2.nextInt(); // ………… complete the rest ……………… while (F1.hasNext() && F2.hasNext()) { if (x<=y) { F3.printf("%4d", x); x = F1.nextInt(); } else { F3.printf("%4d", y); y = F2.nextInt(); } } if (!F1.hasNext()) { while (F2.hasNext()) { F3.printf("%4d", y); y = F2.nextInt(); } } else { while (F1.hasNext()) { F3.printf("%4d", x); x = F1.nextInt(); } } if (x <= y) { F3.printf("%4d", x); F3.printf("%4d", y); } else{ F3.printf("%4d", y); F3.printf("%4d", x); } // ………… complete the rest ……………… // close all files F1.close(); F2.close(); F3.close(); } catch (Exception e){prt.printf("\nOoops! I/O Error:");} prtfile(fn3); // print final sorted file prt.printf("\n\tE N D * M E R G I N G"); } // end merge public static void main(String[] args) throws Exception{ int cnt = args.length; // get no. of arguments if (cnt == 3){ String f1 = args[0]; // get first file name String f2 = args[1]; // get second file name String f3 = args[2]; // get third file name //System.out.printf("\n f1=%s, f2=%s, f3=%s, cnt=%d", f1, f2, f3, cnt); // create an instance of xxxxxH1 class xxxxxH1 srt = new xxxxxH1(); srt.merge(f1, f2, f3); //MAKE SURE TO WRITE YOUR NAME IN NEXT LINE System.out.printf("\n\tAuthor: xxxxx xxxxx Date: " + java.time.LocalDate.now()); } else System.out.printf("\n\tOOOPS Invalid No. of aguments!" + "\n\tTO Execute: java xxxxxH1 f1.txt f2.txt f3.txt"); } // end main } // end xxxxxH1
Can this code be modified so there are three sorted files merged instead of two.
( To compile: javac xxxxxH2.java )
( To execute: java xxxxxH2 f1.txt f2.txt f3.txt f4.txt )
import java.util.*;
import java.io.*;
public class xxxxxH1{
// print k integers per line
int k = 20;
//use a short word for System.out to save typing
PrintStream prt = System.out;
// print file contents
private void prtfile(String fn){
//declare variables
int i=0, x;
prt.printf("\n%s:", fn);
try{
Scanner inf = new Scanner(new File(fn));
while (inf.hasNext()) {
//read an input from inf
x = inf.nextInt();
prt.printf("%3d ", x);
i++;
if(i % k == 0)
prt.printf("\n%s:", fn);
} // endwhile
prt.printf("(count=%d)", i);
// close file
inf.close();
} catch (Exception e){
prt.printf("\nOoops! Read Error:");}
} // end prtfile
// complete following method
private void merge(String fn1, String fn2, String fn3){
int x, y;
try{
// merge two sorted integer files fn1 and fn1 into fn3
prtfile(fn1); // print first sorted file
prtfile(fn2); // print second sorted file
prt.printf("\n\tS T A R T * M E R G I N G\n");
// open input files F1 & F2
Scanner F1 = new Scanner(new File(fn1));
Scanner F2 = new Scanner(new File(fn2));
// open output file F3
PrintWriter F3 = new PrintWriter(new File(fn3));
// read an input from file F1
x = F1.nextInt();
// read an input from file F2
y = F2.nextInt();
// ………… complete the rest ………………
while (F1.hasNext() && F2.hasNext()) {
if (x<=y) {
F3.printf("%4d", x);
x = F1.nextInt();
}
else {
F3.printf("%4d", y);
y = F2.nextInt();
}
}
if (!F1.hasNext()) {
while (F2.hasNext()) {
F3.printf("%4d", y);
y = F2.nextInt();
}
}
else {
while (F1.hasNext()) {
F3.printf("%4d", x);
x = F1.nextInt();
}
}
if (x <= y) {
F3.printf("%4d", x);
F3.printf("%4d", y);
}
else{
F3.printf("%4d", y);
F3.printf("%4d", x);
}
// ………… complete the rest ………………
// close all files
F1.close();
F2.close();
F3.close();
} catch (Exception e){prt.printf("\nOoops! I/O Error:");}
prtfile(fn3); // print final sorted file
prt.printf("\n\tE N D * M E R G I N G");
} // end merge
public static void main(String[] args) throws Exception{
int cnt = args.length; // get no. of arguments
if (cnt == 3){
String f1 = args[0]; // get first file name
String f2 = args[1]; // get second file name
String f3 = args[2]; // get third file name
//System.out.printf("\n f1=%s, f2=%s, f3=%s, cnt=%d", f1, f2, f3, cnt);
// create an instance of xxxxxH1 class
xxxxxH1 srt = new xxxxxH1();
srt.merge(f1, f2, f3);
//MAKE SURE TO WRITE YOUR NAME IN NEXT LINE
System.out.printf("\n\tAuthor: xxxxx xxxxx Date: " +
java.time.LocalDate.now());
}
else
System.out.printf("\n\tOOOPS Invalid No. of aguments!" +
"\n\tTO Execute: java xxxxxH1 f1.txt f2.txt f3.txt");
} // end main
} // end xxxxxH1

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

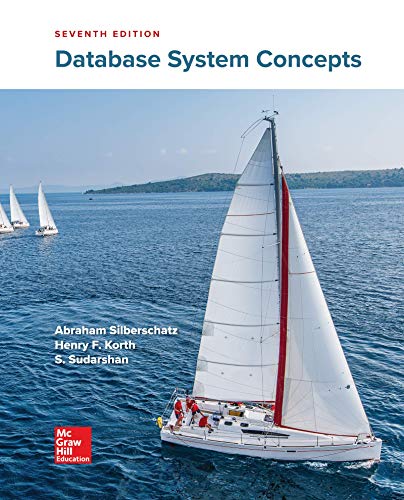
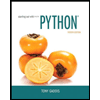
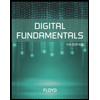
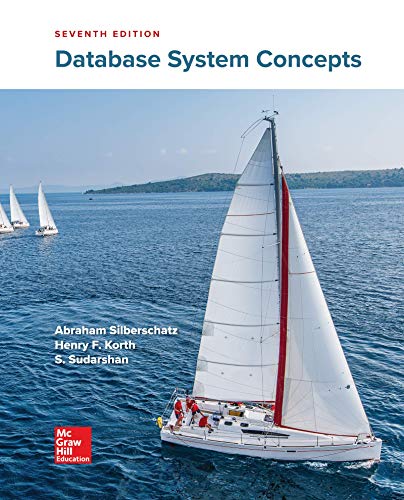
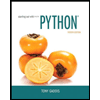
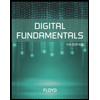
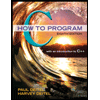
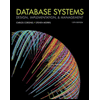
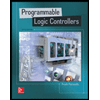