can u make loop portion for the code please. Thank u so much Takes an array of integers and its size as input params and returns a bool such that 'true' ==> all elements of the array are prime, so the array is prime, 'false' ==> at least one element in array is not prime, so array is not prime. Print out a message "Entering " as the first executed statement of each function. Perform the code to test whether every element of the array is a Prime number. Print out a message "Leaving " as the last executed statement before returning from the function. Remember - there will be nested loops for the iterative function and there can be no loops at all in the recursive function. For the recursive function - define one other helper function (IsPrimeRecur) which will recursively check if a number is prime or not - it will take the integer dividend (number to be checked for prime) and the integer divisor to perform the division, return true or false, not contain any loops to check if a number is prime, be the most efficient check possible, and write out the entering and exiting function statement as in #1-2 and #1-4 above. Remember - replace with the actual name of each function. Including your 'main', there will be a total of 4 functions in your program and only the primary helper functions can be invoked from 'main'. No other functions are needed, so do not add unnecessary helper functions. Reminder Note - for all functions except 'main', the 'Entering ' and 'Leaving statements should be printed. Remember: 1 is not a prime number. Hint - try to complete your iterative code first and then convert it piece by piece to the recursive code. sample tests 5 53 5099 1223 567 17 4 1871 8069 3581 6841 #include using namespace std; const int SORT_MAX_SIZE = 8; bool IsPrimeRecur(int dividend, int divisor) { if (divisor == 1) { return true; } if (dividend % divisor == 0) { return false; } return IsPrimeRecur(dividend, divisor-1); } bool IsArrayPrimeRecur(int arr[], int size, int index) { cout << "Entering IsArrayPrimeRecur" << endl; if (index == size) { cout << "Leaving IsArrayPrimeRecur" << endl; return true; } if (!IsPrimeRecur(arr[index], arr[index]-1)) { cout << "Leaving IsArrayPrimeRecur" << endl; return false; } return IsArrayPrimeRecur(arr, size, index+1); } bool IsArrayPrimeIter(int arr[], int size) { cout << "Entering IsArrayPrimeIter" << endl; for (int i = 0; i < size; i++) { for (int j = 2; j < arr[i]; j++) { if (arr[i] % j == 0) { cout << "Leaving IsArrayPrimeIter" << endl; return false; } } } cout << "Leaving IsArrayPrimeIter" << endl; return true; } int main() { cout << "Please enter your input data on one line only." << endl; int size; cin >> size; int arr[SORT_MAX_SIZE]; for (int i = 0; i < size; i++) { cin >> arr[i]; } if (IsArrayPrimeIter(arr, size)) { cout << "Array was found to be prime using iteration" << endl; } else { cout << "Not a Prime Array using iteration" << endl; } if (IsArrayPrimeRecur(arr, size, 0)) { cout << "Array was found to be prime using recursion" << endl; } else { cout << "Not a Prime Array using recursion" << endl; } return 0; }
can u make loop portion for the code please. Thank u so much
- Takes an array of integers and its size as input params and returns a bool such that
- 'true' ==> all elements of the array are prime, so the array is prime,
- 'false' ==> at least one element in array is not prime, so array is not prime.
- Print out a message "Entering <function_name>" as the first executed statement of each function.
- Perform the code to test whether every element of the array is a Prime number.
- Print out a message "Leaving <function_name>" as the last executed statement before returning from the function.
- Remember - there will be nested loops for the iterative function and there can be no loops at all in the recursive function.
- For the recursive function - define one other helper function (IsPrimeRecur) which will recursively check if a number is prime or not - it will
- take the integer dividend (number to be checked for prime) and the integer divisor to perform the division,
- return true or false,
- not contain any loops to check if a number is prime,
- be the most efficient check possible, and
- write out the entering and exiting function statement as in #1-2 and #1-4 above.
- Remember - replace <function_name> with the actual name of each function.
- Including your 'main', there will be a total of 4 functions in your program and only the primary helper functions can be invoked from 'main'. No other functions are needed, so do not add unnecessary helper functions.
- Reminder Note - for all functions except 'main', the 'Entering <function name>' and 'Leaving <function name'> statements should be printed.
- Remember: 1 is not a prime number.
- Hint - try to complete your iterative code first and then convert it piece by piece to the recursive code.
sample tests
- 5 53 5099 1223 567 17
- 4 1871 8069 3581 6841
#include <iostream>
using namespace std;
const int SORT_MAX_SIZE = 8;
bool IsPrimeRecur(int dividend, int divisor) {
if (divisor == 1) {
return true;
}
if (dividend % divisor == 0) {
return false;
}
return IsPrimeRecur(dividend, divisor-1);
}
bool IsArrayPrimeRecur(int arr[], int size, int index) {
cout << "Entering IsArrayPrimeRecur" << endl;
if (index == size) {
cout << "Leaving IsArrayPrimeRecur" << endl;
return true;
}
if (!IsPrimeRecur(arr[index], arr[index]-1)) {
cout << "Leaving IsArrayPrimeRecur" << endl;
return false;
}
return IsArrayPrimeRecur(arr, size, index+1);
}
bool IsArrayPrimeIter(int arr[], int size) {
cout << "Entering IsArrayPrimeIter" << endl;
for (int i = 0; i < size; i++) {
for (int j = 2; j < arr[i]; j++) {
if (arr[i] % j == 0) {
cout << "Leaving IsArrayPrimeIter" << endl;
return false;
}
}
}
cout << "Leaving IsArrayPrimeIter" << endl;
return true;
}
int main() {
cout << "Please enter your input data on one line only." << endl;
int size;
cin >> size;
int arr[SORT_MAX_SIZE];
for (int i = 0; i < size; i++) {
cin >> arr[i];
}
if (IsArrayPrimeIter(arr, size)) {
cout << "Array was found to be prime using iteration" << endl;
} else {
cout << "Not a Prime Array using iteration" << endl;
}
if (IsArrayPrimeRecur(arr, size, 0)) {
cout << "Array was found to be prime using recursion" << endl;
} else {
cout << "Not a Prime Array using recursion" << endl;
}
return 0;
}

Step by step
Solved in 3 steps with 1 images

can you please enble the entering statement?
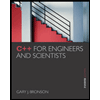
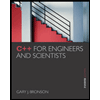