Can you please explain this code to me step-by-step? I especially did not get how s.append(x) is iteratively adding the elements back to the stack without any loop. Note: If you can spot any mistake in the function call, please point that out as well. CODE To Delete Middle Element of a Stack: def deleteMid_util(s, sizeOfStack, current): #if current pointer is half of the size of stack then we #are accessing the middle element of stack. if(current==sizeOfStack//2): s.pop() return #storing the top element in a variable and popping it. x = s.pop() current+=1 #calling the function recursively. deleteMid_util(s,sizeOfStack,current) #pushing the elements (except middle element) back #into stack after recursion calls. s.append(x) def deleteMid(s, sizeOfStack): deleteMid_util(s, sizeOfStack, 0) res = deleteMid([1,2,3,4,5], 5) print(res)
Can you please explain this code to me step-by-step? I especially did not get how s.append(x) is iteratively adding the elements back to the stack without any loop.
Note: If you can spot any mistake in the function call, please point that out as well.
CODE To Delete Middle Element of a Stack:
def deleteMid_util(s, sizeOfStack, current):
#if current pointer is half of the size of stack then we
#are accessing the middle element of stack.
if(current==sizeOfStack//2):
s.pop()
return
#storing the top element in a variable and popping it.
x = s.pop()
current+=1
#calling the function recursively.
deleteMid_util(s,sizeOfStack,current)
#pushing the elements (except middle element) back
#into stack after recursion calls.
s.append(x)
def deleteMid(s, sizeOfStack):
deleteMid_util(s, sizeOfStack, 0)
res = deleteMid([1,2,3,4,5], 5)
print(res)

Step by step
Solved in 3 steps with 1 images

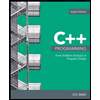
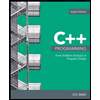