Can you please fix the error in houses.cpp. It’s 3 files. lab2 main.cpp, house.h and house.cpp. this is Lab2mian.cpp: #include #include #include "House.h" using namespace std; // Already implemented for you do not modify int main() { House house1("Lionel Messi", "34 Cedarwood Road", 5, 2.5, 2500000.00); House house2("Neymar da Silva Santos Junior", "12 Broadway Street", 7, 4, 5600000.00); House house3(house1);//Copy constructor House house4; house4 = house2;//Copy assignment // convert house to string and print string house1String = house1; cout << house1String << endl; string house2String = house2; cout << house2String << endl; string house3String = house3; cout << house3String << endl; string house4String = house4; cout << house4String << endl; cout << "----------------------------" << endl << endl; House house5(std::move(house1));//Move constructor House house6("Luke Shaw", "33 Picadilly Avenue", 6, 4.5, 4400000); house6 = std::move(house2);//Move assignment // convert house to string and print house1String = house1; cout << house1String << endl; house2String = house2; cout << house2String << endl; house3String = house3; cout << house3String << endl; house4String = house4; cout << house4String << endl; string house5String = house5; cout << house5String << endl; string house6String = house6; cout << house6String << endl; cout << "----------------------------" << endl << endl; if (house3 == house4) cout << "Houses 3 and 4 are the same" << endl; if (house3 == house5) cout << "Houses 3 and 5 are the same" << endl; if (house3 == house6) cout << "Houses 3 and 6 are the same" << endl; if (house4 == house5) cout << "Houses 4 and 5 are the same" << endl; if (house4 == house6) cout << "Houses 4 and 6 are the same" << endl; if (house5 == house6) cout << "Houses 5 and 6 are the same" << endl; cout << endl << "----------------------------" << endl << endl; house3 += 1000000; house4 += 1000000; house3String = house3; cout << house3String << endl; house4String = house4; cout << house4String << endl; house5String = house5; cout << house5String << endl; house6String = house6; cout << house6String << endl; cout << "----------------------------" << endl << endl; house3 >> house5; house4 >> house6; house3String = house3; cout << house3String << endl; house4String = house4; cout << house4String << endl; house5String = house5; cout << house5String << endl; house6String = house6; cout << house6String << endl; return 0; } #include "House.h" #include #include #include char* deepCopy(const char* src) { if (src == nullptr) return nullptr; char* dest = new char[strlen(src) + 1]; strcpy(dest, src); return dest; } House::House() : owner(nullptr), address(nullptr), rooms(0), bathrooms(0), price(0.0) {} House::House(const char* _owner, const char* _address, const double _rooms, const double _bathrooms, const double _price) : rooms(_rooms), bathrooms(_bathrooms), price(_price) { owner = deepCopy(_owner); address = deepCopy(_address); } House::House(const House& house) : rooms(house.rooms), bathrooms(house.bathrooms), price(house.price) { owner = deepCopy(house.owner); address = deepCopy(house.address); } House& House::operator=(const House& house) { if (this != &house) { delete[] owner; delete[] address; owner = deepCopy(house.owner); address = deepCopy(house.address); rooms = house.rooms; bathrooms = house.bathrooms; price = house.price; } return *this; } House::House(House&& house) noexcept : owner(house.owner), address(house.address), rooms(house.rooms), bathrooms(house.bathrooms), price(house.price) { house.owner = nullptr; house.address = nullptr; } House& House::operator=(House&& house) noexcept { if (this != &house) { delete[] owner; delete[] address; owner = house.owner; address = house.address; rooms = house.rooms; bathrooms = house.bathrooms; price = house.price; house.owner = nullptr; house.address = nullptr; } return *this; } House::~House() { delete[] owner; delete[] address; } bool House::changeOwner(const char* newOwner) { delete[] owner; owner = deepCopy(newOwner); return true; } bool House::changePrice(double newPrice) { price = newPrice; return true; }
Can you please fix the error in houses.cpp. It’s 3 files. lab2 main.cpp, house.h and house.cpp. this is Lab2mian.cpp: #include #include #include "House.h" using namespace std; // Already implemented for you do not modify int main() { House house1("Lionel Messi", "34 Cedarwood Road", 5, 2.5, 2500000.00); House house2("Neymar da Silva Santos Junior", "12 Broadway Street", 7, 4, 5600000.00); House house3(house1);//Copy constructor House house4; house4 = house2;//Copy assignment // convert house to string and print string house1String = house1; cout << house1String << endl; string house2String = house2; cout << house2String << endl; string house3String = house3; cout << house3String << endl; string house4String = house4; cout << house4String << endl; cout << "----------------------------" << endl << endl; House house5(std::move(house1));//Move constructor House house6("Luke Shaw", "33 Picadilly Avenue", 6, 4.5, 4400000); house6 = std::move(house2);//Move assignment // convert house to string and print house1String = house1; cout << house1String << endl; house2String = house2; cout << house2String << endl; house3String = house3; cout << house3String << endl; house4String = house4; cout << house4String << endl; string house5String = house5; cout << house5String << endl; string house6String = house6; cout << house6String << endl; cout << "----------------------------" << endl << endl; if (house3 == house4) cout << "Houses 3 and 4 are the same" << endl; if (house3 == house5) cout << "Houses 3 and 5 are the same" << endl; if (house3 == house6) cout << "Houses 3 and 6 are the same" << endl; if (house4 == house5) cout << "Houses 4 and 5 are the same" << endl; if (house4 == house6) cout << "Houses 4 and 6 are the same" << endl; if (house5 == house6) cout << "Houses 5 and 6 are the same" << endl; cout << endl << "----------------------------" << endl << endl; house3 += 1000000; house4 += 1000000; house3String = house3; cout << house3String << endl; house4String = house4; cout << house4String << endl; house5String = house5; cout << house5String << endl; house6String = house6; cout << house6String << endl; cout << "----------------------------" << endl << endl; house3 >> house5; house4 >> house6; house3String = house3; cout << house3String << endl; house4String = house4; cout << house4String << endl; house5String = house5; cout << house5String << endl; house6String = house6; cout << house6String << endl; return 0; } #include "House.h" #include #include #include char* deepCopy(const char* src) { if (src == nullptr) return nullptr; char* dest = new char[strlen(src) + 1]; strcpy(dest, src); return dest; } House::House() : owner(nullptr), address(nullptr), rooms(0), bathrooms(0), price(0.0) {} House::House(const char* _owner, const char* _address, const double _rooms, const double _bathrooms, const double _price) : rooms(_rooms), bathrooms(_bathrooms), price(_price) { owner = deepCopy(_owner); address = deepCopy(_address); } House::House(const House& house) : rooms(house.rooms), bathrooms(house.bathrooms), price(house.price) { owner = deepCopy(house.owner); address = deepCopy(house.address); } House& House::operator=(const House& house) { if (this != &house) { delete[] owner; delete[] address; owner = deepCopy(house.owner); address = deepCopy(house.address); rooms = house.rooms; bathrooms = house.bathrooms; price = house.price; } return *this; } House::House(House&& house) noexcept : owner(house.owner), address(house.address), rooms(house.rooms), bathrooms(house.bathrooms), price(house.price) { house.owner = nullptr; house.address = nullptr; } House& House::operator=(House&& house) noexcept { if (this != &house) { delete[] owner; delete[] address; owner = house.owner; address = house.address; rooms = house.rooms; bathrooms = house.bathrooms; price = house.price; house.owner = nullptr; house.address = nullptr; } return *this; } House::~House() { delete[] owner; delete[] address; } bool House::changeOwner(const char* newOwner) { delete[] owner; owner = deepCopy(newOwner); return true; } bool House::changePrice(double newPrice) { price = newPrice; return true; }
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 5RQ
Related questions
Question
Can you please fix the error in houses.cpp.
It’s 3 files. lab2 main.cpp, house.h and house.cpp.
this is Lab2mian.cpp: #include
#include
#include "House.h"
using namespace std;
// Already implemented for you do not modify
int main() {
House house1("Lionel Messi", "34 Cedarwood Road", 5, 2.5, 2500000.00);
House house2("Neymar da Silva Santos Junior", "12 Broadway Street", 7, 4, 5600000.00);
House house3(house1);//Copy constructor
House house4;
house4 = house2;//Copy assignment
// convert house to string and print
string house1String = house1;
cout << house1String << endl;
string house2String = house2;
cout << house2String << endl;
string house3String = house3;
cout << house3String << endl;
string house4String = house4;
cout << house4String << endl;
cout << "----------------------------" << endl << endl;
House house5(std::move(house1));//Move constructor
House house6("Luke Shaw", "33 Picadilly Avenue", 6, 4.5, 4400000);
house6 = std::move(house2);//Move assignment
// convert house to string and print
house1String = house1;
cout << house1String << endl;
house2String = house2;
cout << house2String << endl;
house3String = house3;
cout << house3String << endl;
house4String = house4;
cout << house4String << endl;
string house5String = house5;
cout << house5String << endl;
string house6String = house6;
cout << house6String << endl;
cout << "----------------------------" << endl << endl;
if (house3 == house4) cout << "Houses 3 and 4 are the same" << endl;
if (house3 == house5) cout << "Houses 3 and 5 are the same" << endl;
if (house3 == house6) cout << "Houses 3 and 6 are the same" << endl;
if (house4 == house5) cout << "Houses 4 and 5 are the same" << endl;
if (house4 == house6) cout << "Houses 4 and 6 are the same" << endl;
if (house5 == house6) cout << "Houses 5 and 6 are the same" << endl;
cout << endl << "----------------------------" << endl << endl;
house3 += 1000000;
house4 += 1000000;
house3String = house3;
cout << house3String << endl;
house4String = house4;
cout << house4String << endl;
house5String = house5;
cout << house5String << endl;
house6String = house6;
cout << house6String << endl;
cout << "----------------------------" << endl << endl;
house3 >> house5;
house4 >> house6;
house3String = house3;
cout << house3String << endl;
house4String = house4;
cout << house4String << endl;
house5String = house5;
cout << house5String << endl;
house6String = house6;
cout << house6String << endl;
return 0;
}
#include "House.h"
#include
#include
#include
char* deepCopy(const char* src) {
if (src == nullptr) return nullptr;
char* dest = new char[strlen(src) + 1];
strcpy(dest, src);
return dest;
}
House::House() : owner(nullptr), address(nullptr), rooms(0), bathrooms(0), price(0.0) {}
House::House(const char* _owner, const char* _address, const double _rooms, const double _bathrooms, const double _price)
: rooms(_rooms), bathrooms(_bathrooms), price(_price) {
owner = deepCopy(_owner);
address = deepCopy(_address);
}
House::House(const House& house)
: rooms(house.rooms), bathrooms(house.bathrooms), price(house.price) {
owner = deepCopy(house.owner);
address = deepCopy(house.address);
}
House& House::operator=(const House& house) {
if (this != &house) {
delete[] owner;
delete[] address;
owner = deepCopy(house.owner);
address = deepCopy(house.address);
rooms = house.rooms;
bathrooms = house.bathrooms;
price = house.price;
}
return *this;
}
House::House(House&& house) noexcept
: owner(house.owner), address(house.address), rooms(house.rooms), bathrooms(house.bathrooms), price(house.price) {
house.owner = nullptr;
house.address = nullptr;
}
House& House::operator=(House&& house) noexcept {
if (this != &house) {
delete[] owner;
delete[] address;
owner = house.owner;
address = house.address;
rooms = house.rooms;
bathrooms = house.bathrooms;
price = house.price;
house.owner = nullptr;
house.address = nullptr;
}
return *this;
}
House::~House() {
delete[] owner;
delete[] address;
}
bool House::changeOwner(const char* newOwner) {
delete[] owner;
owner = deepCopy(newOwner);
return true;
}
bool House::changePrice(double newPrice) {
price = newPrice;
return true;
}

Transcribed Image Text:Lab2main.cpp
hourse
1
3
u
5
6
7
8
9
10
11
12
13
14
15
16
17
19
20
24
37
38
39
40
41
42
43
house.h Xhouse.cpp*
class declaration for a house
// Already implemented for you do not modify
#ifndef _HOUSE_H_
#define _HOUSE_H_
class House {
char* owner;
char* address;
double rooms;
double bathrooms;
double price;
public:
House();//Default constructor
House(const char* _owner, const char* _address, const double rooms, const double bathrooms, const double price);//Data for the house
House(const House& house); //Copy constructor
House& operator (const House& house); //Copy assignment
House (House&& house) noexcept; //Move constructor
House& operator (House&& house) noexcept;//Move assignment
// setters
bool changeOwner(const char* newOwner);//Change the owner
bool changePrice (double newPrice); //Change the price
// operators
operator std::string() const;// convert house object to a string (check the sample output)
const House& operator+=(double amount);//increment/decrement the price of the house
friend bool operator==(const House& housel, const House& house2);//Do the two houses have the same owner?
// getters
const char* getOwner() const; //Return the owner
const char* getAddress() const;//Return the address
const double getRooms() const;//Return the number of rooms
→g House
const double getBathrooms () const;//Return the number of bathrooms
const double getPrice() const;//Return the price
~House();
//Destructor
This is house.h
};
bool operator==(const House& housel, const House& house2);//Do the two houses have the same owner?
void operator>>(House& housel, House& house2);//Move contents from the housel object to the house2 object
#endif// _HOUSE_H__
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
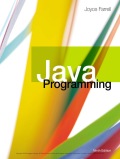
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
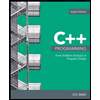
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
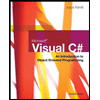
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
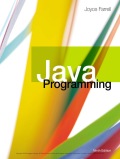
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
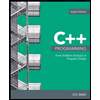
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
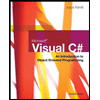
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
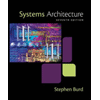
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning