can you provide assumptions and demonstration for this code?? only C programming #include #include #include // Function Declarations void create_inventory(); void update_vacc_qty(); int search_vaccine(); void display_vaccine(); // Main Function starts here int main() { create_inventory(); display_vaccine(); search_vaccine(); //update_vacc_qty(); return 0; } //Function to Create Vaccine.txt as per the given table void create_inventory() { int option = 1; // variables to collect data as per table given char vaccinename[15]; char vaccinecode[2]; char country[15]; int dosage; float populaion; //File definition FILE* infile; infile = fopen("Vaccine.txt","w"); // file opening for writing if (infile == NULL) // Checking for the file creation { printf("Vaccine.txt file unfamiliar\n"); } //Accepting data from user from keyboard till user enters 0 to close while (option != 0) { printf("Enter Vaccine Name : "); scanf("%s", vaccinename); printf("Enter Vaccine Code : "); scanf("%s", vaccinecode); printf("Enter Counry Name : "); scanf("%s", country); printf("Enter Dosage Required : "); scanf("%d", &dosage); printf("Enter Population Covered : "); scanf("%f", &populaion); //writing to the file using fprintf command fprintf(infile, "%s %s %s %d %3.2f\n",vaccinename, vaccinecode, country, dosage, populaion); printf("\n press 1 to pursue and 0 to exit : "); scanf("%d", &option); if (option == 0) fclose(infile); // closing the file when user wants to exit } } //Function to display the file contents in a formatted way void display_vaccine() { // variables to collect data as per table given char vaccinename[15]; char vaccinecode[2]; char country[15]; int dosage; float populaion; FILE* infile; infile = fopen("Vaccine.txt", "r"); // file opening for reading if (infile == NULL) //checking for file exists or not { printf("Vaccine.txt file unfamiliar\n"); } //printing the header line printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", "Vaccine Code", "Country", "Dosage", "Population"); // Reading the file while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF) { //printing the read data in a formatted way printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion); } fclose(infile); // closing the file } int search_vaccine() { // variables to collect data as per table given char vaccinename[15]; char vaccinecode[2]; char country[15]; int dosage; float populaion; FILE* infile; char vcode[2]; char temp[2]; int value; infile = fopen("Vaccine.txt", "r"); // file opening for reading //getting the vaccine code from user through keyboard to search printf("Enter Vaccine Code to explore : "); scanf("%s", vcode); if (infile == NULL) // checking for file existence { printf("Vaccine.txt file unfamiliar\n"); } strcpy(temp, vcode); //Reading the file while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF) { //checking user entered vaccine code and available in the file is same if (vaccinecode[0] == temp[0] && vaccinecode[1] == temp[1]) { // Printing the matched record printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", " Vaccine Code", "Country", "Dosage", "Population"); printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion); } } fclose(infile); // closing the file }//end
can you provide assumptions and demonstration for this code?? only C programming
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Function Declarations
void create_inventory();
void update_vacc_qty();
int search_vaccine();
void display_vaccine();
// Main Function starts here
int main()
{
create_inventory();
display_vaccine();
search_vaccine();
//update_vacc_qty();
return 0;
}
//Function to Create Vaccine.txt as per the given table
void create_inventory()
{
int option = 1;
// variables to collect data as per table given
char vaccinename[15];
char vaccinecode[2];
char country[15];
int dosage;
float populaion;
//File definition
FILE* infile;
infile = fopen("Vaccine.txt","w"); // file opening for writing
if (infile == NULL) // Checking for the file creation
{
printf("Vaccine.txt file unfamiliar\n");
}
//Accepting data from user from keyboard till user enters 0 to close
while (option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s", vaccinename);
printf("Enter Vaccine Code : ");
scanf("%s", vaccinecode);
printf("Enter Counry Name : ");
scanf("%s", country);
printf("Enter Dosage Required : ");
scanf("%d", &dosage);
printf("Enter Population Covered : ");
scanf("%f", &populaion);
//writing to the file using fprintf command
fprintf(infile, "%s %s %s %d %3.2f\n",vaccinename, vaccinecode, country, dosage, populaion);
printf("\n press 1 to pursue and 0 to exit : ");
scanf("%d", &option);
if (option == 0)
fclose(infile); // closing the file when user wants to exit
}
}
//Function to display the file contents in a formatted way
void display_vaccine()
{
// variables to collect data as per table given
char vaccinename[15];
char vaccinecode[2];
char country[15];
int dosage;
float populaion;
FILE* infile;
infile = fopen("Vaccine.txt", "r"); // file opening for reading
if (infile == NULL) //checking for file exists or not
{
printf("Vaccine.txt file unfamiliar\n");
}
//printing the header line
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", "Vaccine Code", "Country", "Dosage", "Population");
// Reading the file
while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF)
{
//printing the read data in a formatted way
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion);
}
fclose(infile); // closing the file
}
int search_vaccine()
{
// variables to collect data as per table given
char vaccinename[15];
char vaccinecode[2];
char country[15];
int dosage;
float populaion;
FILE* infile;
char vcode[2];
char temp[2];
int value;
infile = fopen("Vaccine.txt", "r"); // file opening for reading
//getting the vaccine code from user through keyboard to search
printf("Enter Vaccine Code to explore : ");
scanf("%s", vcode);
if (infile == NULL) // checking for file existence
{
printf("Vaccine.txt file unfamiliar\n");
}
strcpy(temp, vcode);
//Reading the file
while (fscanf(infile, "%s %s %s %d %f\n", vaccinename, vaccinecode, country, &dosage, &populaion) != EOF)
{
//checking user entered vaccine code and available in the file is same
if (vaccinecode[0] == temp[0] && vaccinecode[1] == temp[1])
{
// Printing the matched record
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", " Vaccine Code", "Country", "Dosage", "Population");
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", vaccinename, vaccinecode, country, dosage, populaion);
}
}
fclose(infile); // closing the file
}//end

Step by step
Solved in 3 steps with 3 images

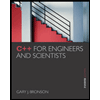
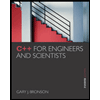