CollegeApplicant Class Write a class encapsulating the concept of College Applicant. Use the class diagram above as well as the specific requirements below to develop your class: Class Members name - The name of the college applicant. college - The name of the college. CollegeApplicant() - Initializes an instance of the CollegeApplicant class where the name and college are set to "unknown". CollegeApplicant(String, String - Initializes an instance of the CollegeApplicant class where the name and college are set to the specified values. getName() : String - Returns the college applicant's name. setName(String) : void - Sets the college applicant's name. getCollege() : String - Returns the name of the college. setCollege(String) : void - Sets the name of the college. registerForProgram(String) : String - Returns a String representing the registration of a program. toString() : String - Returns a String representation of the class. toString() Format: ======================= College Applicant ======================= Name: {name} College: {college} Sample: ======================= College Applicant ======================= Name: Andrew Jones College: BIT College GraduateApplicant Class Write a class encapsulating the concept of a Graduate Applicant. Use the class diagram above as well as the specific requirements below to develop your class: Class Members collegeOfOrigin - The name of the college where the graduate applicant received their degree. GraduateApplicant(String, String, String) - Initializes an instance of the GraduateApplicant class with a specified name, college name, and college of origin. getCollegeOfOrigin() : String - Returns the college of origin. setCollegeOfOrigin(String) : void - Sets the college of origin. isInside() : boolean - Returns true when the college name and the college of origin are the same (case should not be a factor); otherwise false. registerForProgram(String) : String - Returns the String "{college_of_origin} > college_name - program_name". Example: "Red River College > Red River College - Business Administration". toString() : String - Returns a String representation of the class. Format: ======================= College Applicant ======================= Name: {name} College: {college} Origin: {collegeOfOrigin} Sample: ======================= College Applicant ======================= Name: Andrew Jones College: BIT College Origin: Yale UndergraduateApplicant Class Write a class encapsulating the concept of a Undergraduate Applicant. Use the class diagram above as well as the specific requirements below to develop your class: Class Members standardAptitudeTestScore - The score achieved on the standard aptitude test. Allowable values: 0.0 - 500.0 inclusive. If this field is set outside the allowable range, set the state to 0 (zero). gradePointAverage - The applicants grade point average. Allowable values: 0.00 - 4.50 inclusive. If this field is set outside the allowable range, set the state to 0 (zero). UndergraduateApplicant(String, String, double, double) - Initializes an instance of the UndergraduateApplicant class with the specified applicant name, college name, standard aptitude test score and grade point average. getStandardAptitudeTestScore() : double - Returns the standard aptitude test score. setStandardAptitudeTestScore(double) : void - Sets the standard aptitude test score. getGradePointAverage() : double - Returns the grade point average. setGradePointAverage(double) : void - Sets the grade point average. registerForProgram(String) : String - Returns the String "college_name - program_name [aptitude_test_score]". Example: "Red River College - Business Information Technology [465.3]". toString() : String - Returns a String representation of the class. toString() Format: ======================= College Applicant ======================= Name: {name} College: {college} SAT Score: {standardAptitudeTest} GPA: {gradePointAverage} Formatting Requirements The standard aptitude test score is formatted to one decimal place. The grade point average is formatted to two decimal places. Sample: ======================= College Applicant ======================= Name: Andrew Jones College: BIT College SAT Score: 465.3 GPA: 4.22
Folder Structure
Your project will contain two sub-directories:
- library
- test
In the library directory, create the classes in the class diagram below. In the test directory, create the test applications.
Class Diagram
CollegeApplicant Class
Write a class encapsulating the concept of College Applicant. Use the class diagram above as well as the specific requirements below to develop your class:
Class Members
- name - The name of the college applicant.
- college - The name of the college.
- CollegeApplicant() - Initializes an instance of the CollegeApplicant class where the name and college are set to "unknown".
- CollegeApplicant(String, String - Initializes an instance of the CollegeApplicant class where the name and college are set to the specified values.
- getName() : String - Returns the college applicant's name.
- setName(String) : void - Sets the college applicant's name.
- getCollege() : String - Returns the name of the college.
- setCollege(String) : void - Sets the name of the college.
- registerForProgram(String) : String - Returns a String representing the registration of a program.
- toString() : String - Returns a String representation of the class.
toString() Format:
Sample:
GraduateApplicant Class
Write a class encapsulating the concept of a Graduate Applicant. Use the class diagram above as well as the specific requirements below to develop your class:
Class Members
- collegeOfOrigin - The name of the college where the graduate applicant received their degree.
- GraduateApplicant(String, String, String) - Initializes an instance of the GraduateApplicant class with a specified name, college name, and college of origin.
- getCollegeOfOrigin() : String - Returns the college of origin.
- setCollegeOfOrigin(String) : void - Sets the college of origin.
- isInside() : boolean - Returns true when the college name and the college of origin are the same (case should not be a factor); otherwise false.
- registerForProgram(String) : String - Returns the String "{college_of_origin} > college_name - program_name". Example: "Red River College > Red River College - Business Administration".
- toString() : String - Returns a String representation of the class.
Format:
Sample:
UndergraduateApplicant Class
Write a class encapsulating the concept of a Undergraduate Applicant. Use the class diagram above as well as the specific requirements below to develop your class:
Class Members
- standardAptitudeTestScore - The score achieved on the standard aptitude test. Allowable values: 0.0 - 500.0 inclusive. If this field is set outside the allowable range, set the state to 0 (zero).
- gradePointAverage - The applicants grade point average. Allowable values: 0.00 - 4.50 inclusive. If this field is set outside the allowable range, set the state to 0 (zero).
- UndergraduateApplicant(String, String, double, double) - Initializes an instance of the UndergraduateApplicant class with the specified applicant name, college name, standard aptitude test score and grade point average.
- getStandardAptitudeTestScore() : double - Returns the standard aptitude test score.
- setStandardAptitudeTestScore(double) : void - Sets the standard aptitude test score.
- getGradePointAverage() : double - Returns the grade point average.
- setGradePointAverage(double) : void - Sets the grade point average.
- registerForProgram(String) : String - Returns the String "college_name - program_name [aptitude_test_score]". Example: "Red River College - Business Information Technology [465.3]".
- toString() : String - Returns a String representation of the class.
toString() Format:
Formatting Requirements
- The standard aptitude test score is formatted to one decimal place.
- The grade point average is formatted to two decimal places.
Sample:
Documentation
Using the javadoc program, publish the documentation for all library classes.
Directory Structure
1008_firstname_lastname_assignment_7.zip
├── library
│ └── docs
│ └── CollegeApplicant.java
│ └── GraduateApplicant.java
│ └── UndergraduateApplicant.java
└


Step by step
Solved in 3 steps with 2 images

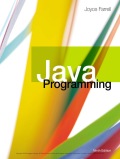
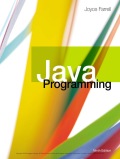