Come up with a better way in which you swap the elements directly. Hint: How do you swap the two elements A and D? A B C D E F D B C A E F D E C A B F D E F A B C Write pseudocode for this algorithm. Add two more letters and run the program again to double-check that it works with any even number of letters.
Come up with a better way in which you swap the elements directly. Hint: How do you swap the two elements A and D? A B C D E F D B C A E F D E C A B F D E F A B C Write pseudocode for this algorithm. Add two more letters and run the program again to double-check that it works with any even number of letters.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
run the main() method of class Lab11D.
Pls do the SWAPIN LAB 11D and below is the tester
For example, A B C D E F should turn into D E F A B C.
You should assume that the array list has an even number of elements (not necessarily 6).
One solution is to keep removing the element at index 0 and adding it to the back.
Each step would look like this:
A B C D E F
B C D E F A
C D E F A B
D E F A B C
When you run the code, you will find that there is a lot of movement in the array list. Each call to remove(0) causes n - 1 elements to move, where n is the length of the array. If n is 100, then you move 99 elements 50 times, (almost 5000 move operations). That's an inefficient way of swapping the first and second halves. (Learn more about time complexity at the end of the lab).
Come up with a better way in which you swap the elements directly. Hint: How do you swap the two elements A and D?
A B C D E F
D B C A E F
D E C A B F
D E F A B C
Write pseudocode for this algorithm .
Add two more letters and run the program again to double-check that it works with any even number of letters. Many more files are provided, and you could add even more letters to run the program
import java.util.*;
/**
* Swappping the first part and the second part of an array list.
*
* @author
* @version
*/
public class Lab11D
{
public static void main(String[] args)
{
VisualArrayList list = new VisualArrayList();
list.add(new Picture("a.jpeg"));
list.add(new Picture("b.jpeg"));
list.add(new Picture("c.jpeg"));
list.add(new Picture("d.jpeg"));
list.add(new Picture("e.jpeg"));
list.add(new Picture("f.jpeg"));
// your work here: swap the first and second parts of the array list.
}
}
TESTER
import java.util.ArrayList;
public class VisualArrayList extends ArrayList
{
private static final int GAP = 10;
private void visualTranslate(final int dx, final int dy, final Picture p)
{
final int x = p.getX();
final int y = p.getY();
Thread t = new Thread()
{
public void run()
{
final int STEPS = 100;
final int DELAY = 10;
for (int i = 1; i = index; i--)
{
Picture p = get(i);
visualTranslate(element.getWidth() + GAP, 0, p);
}
super.add(index, element);
int x = index == 0 ? 0 : get(index - 1).getX() + get(index - 1).getWidth() + GAP;
element.translate(x - element.getX(), element.getHeight() -element.getY());
element.draw();
visualTranslate(0, -element.getHeight(), element);
}
public E remove(int index)
{
E element = super.remove(index);
visualTranslate(0, -element.getHeight(), element);
for (int i = index; i < size(); i++)
{
Picture p = get(i);
visualTranslate(-element.getWidth() - GAP, 0, p);
}
return element;
}
public E set(int index, E element)
{
E oldElement = get(index);
super.set(index, null);
if (!contains(oldElement)) Canvas.getInstance().remove(oldElement);
int dx = element.getWidth() - oldElement.getWidth();
for (int i = index + 1; i < size(); i++)
get(i).translate(dx, 0);
int x = index == 0 ? 0 : get(index - 1).getX() + get(index - 1).getWidth() + GAP;
element.translate(x - element.getX(), element.getHeight() -element.getY());
element.draw();
visualTranslate(0, -element.getHeight(), element);
super.set(index, element);
return oldElement;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
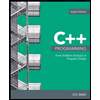
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
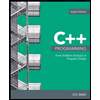
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning