Consider the following game between two players: Both players simultaneously declare "one" or "two". Player 1 wins if the sum of the two declared numbers is odd and Player 2 wins if the sum is even. In either case the loser is obliged to pay the winner (in tokens) the sum of the two declared numbers. So Player 1 may have to pay 2 or 4 tokens or may win 3 tokens. **Part 1:** Write a computer program in Java that allows a user to play this game against a computer. The computer's strategy will be as follows. A computer player will have a threshold variable, `t`. The computer will generate a random number between 0 and 1. If the number is greater than `t` the computer will declare "two" if the random number is less than `t` the computer will declare "one". I have included templates for a `Game` class, a computer `ComputerPlayer` class and a test class, `OddEven` on Codio. Note that there is no class for the human player as this can be handled easily enough in the Game class. **** I have attached the photo of 5 java files in one screenshot. Please add code to ComputerPlayer.java, Game.java and Simulation.java. The files OddEven.java and SimTest.java do not need to modify. ** *** This is part one of the question and I will post part two later. ***
Consider the following game between two players: Both players simultaneously declare "one" or "two". Player 1 wins if the sum of the two declared numbers is odd and Player 2 wins if the sum is even. In either case the loser is obliged to pay the winner (in tokens) the sum of the two declared numbers. So Player 1 may have to pay 2 or 4 tokens or may win 3 tokens.
**Part 1:** Write a computer program in Java that allows a user to play this game against a computer. The computer's strategy will be as follows. A computer player will have a threshold variable, `t`. The computer will generate a random number between 0 and 1. If the number is greater than `t` the computer will declare "two" if the random number is less than `t` the computer will declare "one". I have included templates for a `Game` class, a computer `ComputerPlayer` class and a test class, `OddEven` on Codio. Note that there is no class for the human player as this can be handled easily enough in the Game class.
**** I have attached the photo of 5 java files in one screenshot. Please add code to ComputerPlayer.java, Game.java and Simulation.java. The files OddEven.java and SimTest.java do not need to modify. **
*** This is part one of the question and I will post part two later. ***
*
![O ComputerPlayer.java
OddEven.java
/**
* This class represents a computer
* player in the Odd-Even game
/**
* A tester for the Odd-Even game.
Your code must work with this program. Do not modify this class.
*/
public class OddEven{
public class ComputerPlayer{
private double t;
private int tokenBalance;
public static void main(String ] args){
Game g = new Game ();
g.play();
public ComputerPlayer(double threshold){
t=threshold;
tokenBalance=0;
// your code here
SimTest.java
/***
* This is a sample tester for Part 2 of your program
* We will use something similar to this to test your work
Your program must work with this tester
I Game.java
**
* This class represents the Odd-Even game
*/
public class SimTest{
public static void main(String ] args){
//instantiate a game with two computer players
Game g = new Game(.5,.5);
g.play(1000); // play 1000 games. Please, no print statements.
System.out.println("Player i now has "+ g.getP1Score()+" tokens.");
System.out.printin("Player 2 now has "+ g.getP2Score()+" tokens.");
public class Game{
// your instance variables here:
/* this version of the game constructor is for Part 1
* it takes no parameters */
public Game (){
// your code here
/* this version of the game constructor is for Part 2
* It requires two doubles as parameters. You will use
* these to set the initial thresholds for you computer players */
public Game (double t1, double t2){
// your code here
/* this version of the play method is for Part 1
* It takes no parameters and should play the interactive
* version of the game */
public void play(){
// your code here
Simulation.java
/**
* This class should run simulations to determine
* whether or not the Od-Even game is fair and if
* not who has the advantage and what is a strategy
* that will realize that adavantage.
/** this version of the play method is for Part 2
* It takes a single int as a parameter which is the
* number of computer vs. computer games that should be played */
public void play(int games){
// your code here
*/
/* this method should return the current score (number of tokens)
* that player 1 has */
public int getP1Score(){
// your code here
public class Simulation{
public static void main(String [] args){
// your code here
}
/* this method should return the current score (number of tokens)
that player 2 has */
public int getP2Score() {
// your code here
}
// you may or may not want more methods here:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8bfe1080-e0d5-4baa-b4e3-fde34e28e788%2F0948f203-cfb4-42c1-b8a8-ff6e261af132%2Fe1x2fij_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

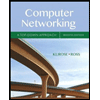
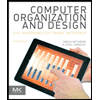
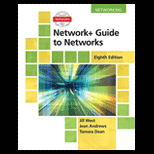
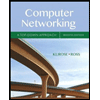
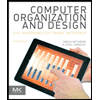
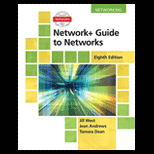
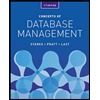
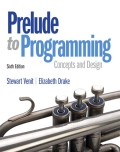
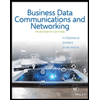