Convert this script from using a list to using a system arry instead. using UnityEngine; using System.Collections; using System.Collections.Generic; using UdonSharp; using UnityEngine; using VRC.SDKBase; using VRC.Udon; using System.Collections; using System.Collections.Generic; public class Randomsoundeffect : UdonSharpBehaviour { public List audioClips; public AudioClip currentClip; public AudioSource source; public float minWaitBetweenPlays = 1f; public float maxWaitBetweenPlays = 5f; public float waitTimeCountdown = -1f; public class RandomSoundsScript : MonoBehaviour { public AudioSource randomSound; public AudioClip[] audioSources; // Use this for initialization void Start () { CallAudio (); } void CallAudio() { Invoke ("RandomSoundness", 10); } void RandomSoundness() { randomSound.clip = audioSources[Random.Range(0, audioSources.Length)]; randomSound.Play (); CallAudio (); } } void Start() { source = GetComponent(); } void Update() { if (!source.isPlaying) { if (waitTimeCountdown < 0f) { currentClip = audioClips[Random.Range(0, audioClips.Count)]; source.clip = currentClip; source.Play(); waitTimeCountdown = Random.Range(minWaitBetweenPlays, maxWaitBetweenPlays); } else { waitTimeCountdown -= Time.deltaTime; } } } }
Convert this script from using a list to using a system arry instead.
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
using UdonSharp;
using UnityEngine;
using VRC.SDKBase;
using VRC.Udon;
using System.Collections;
using System.Collections.Generic;
public class Randomsoundeffect : UdonSharpBehaviour
{
public List<AudioClip> audioClips;
public AudioClip currentClip;
public AudioSource source;
public float minWaitBetweenPlays = 1f;
public float maxWaitBetweenPlays = 5f;
public float waitTimeCountdown = -1f;
public class RandomSoundsScript : MonoBehaviour {
public AudioSource randomSound;
public AudioClip[] audioSources;
// Use this for initialization
void Start () {
CallAudio ();
}
void CallAudio()
{
Invoke ("RandomSoundness", 10);
}
void RandomSoundness()
{
randomSound.clip = audioSources[Random.Range(0, audioSources.Length)];
randomSound.Play ();
CallAudio ();
}
}
void Start()
{
source = GetComponent<AudioSource>();
}
void Update()
{
if (!source.isPlaying)
{
if (waitTimeCountdown < 0f)
{
currentClip = audioClips[Random.Range(0, audioClips.Count)];
source.clip = currentClip;
source.Play();
waitTimeCountdown = Random.Range(minWaitBetweenPlays, maxWaitBetweenPlays);
}
else
{
waitTimeCountdown -= Time.deltaTime;
}
}
}
}

Step by step
Solved in 2 steps

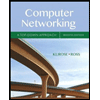
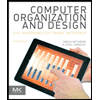
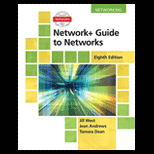
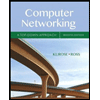
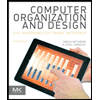
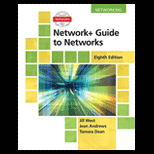
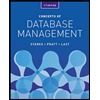
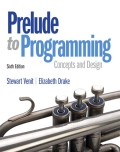
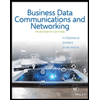