Create a Java application InputAndArithmetic that uses a Scanner object to do the following: 1. Ask the user to enter an integer using prompt "Enter an integer: " 2. Get the integer and store it in an integer variable 3. Print the integer on a new line 4. Ask the user to enter another integer using prompt "Enter a second integer: 5. Get the second integer and store it in another integer variable 6. Print the second integer on a new line 7. Calculate the product of the two integers 8. Print the product on a new line 9. Calculate the double quotient of the first integer divided by the second 10. Print the double quotient on a new line. 11. Calculate the integer quotient of the first integer divided by the second 12. Print the integer quotient on a new line. 13. Calculate the remainder of the first integer divided by the second 14. Print the remainder on a new line. 11 Sample output Enter an integer: 3 The first integer is 3. Enter a second integer: The second integer is 5. The product of the two integers is 15. The double quotient of the first integer divided by the second integer is 0.6. The integer quotient of the first integer divided by the second integer is 0. The remainder of the first integer divided by the second integer is 3. You should create just one Scanner object. 5


import java.util.Scanner;
public class InputAndArithmetic
{
public static void main(String[] args)
{
int num1, num2;
Scanner sc1 = new Scanner(System.in);
Scanner sc2 = new Scanner(System.in);
System.out.print("Enter an integer: ");
num1 = sc1.nextInt();
System.out.print("The first integer is " + num1 + ".");
System.out.print("\nEnter a second integer: ");
num2 = sc2.nextInt();
System.out.print("The second integer is " + num2 + ".");
int product = num1*num2;
System.out.print("\nThe product of the two integers is " + product + ".");
double quotient1 = (double)num1/num2;
System.out.print("\nThe double quotient of the first integer divided by the second integer is " + quotient1 + ".");
int quotient2 = num1/num2;
System.out.print("\nThe integer quotient of the first integer divided by the second integer is " + quotient2 + ".");
int remainder = num1%num2;
System.out.print("\nThe remainder of the first integer divided by the second integer is " + remainder + ".");
}
}
Step by step
Solved in 2 steps with 1 images

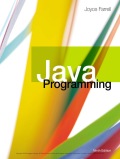
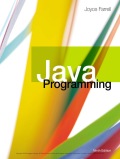