Create a program that exhibits inheritance and
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Objective:
At the end of the activity, the students should be able to:
- Create a
program that exhibits inheritance and
Software Requirements:
- Latest version of NetBeans IDE
- Java Development Kit (JDK) 8
Procedure:
- Create four (4) Java classes. Name them RunEmployee, Employee, FullTimeEmployee, PartTimeEmployee. The RunEmployee class shall contain the main method and will be used to execute the
- Write a simple payroll program that will display employee’s information. Refer to the UML Class Diagram for the names of the variable and method. This should be the sequence of the program upon execution:
- Ask the user to input the name of the
- Prompt the user to select between full time and part time by pressing either F (full time) or P (part time).
- If F is pressed, ask the user to type his monthly salary. Then, display his name and monthly salary.
If P is pressed, ask the user to type his rate (pay) per hour and the number of hours he worked for the entire month separated by a space. Then, display his name and wage.
Note: You can add variables and methods if needed. Just make sure that all the variables and methods in the diagram are properly used.



Step by step
Solved in 2 steps with 1 images

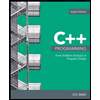
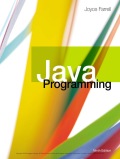
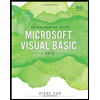
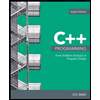
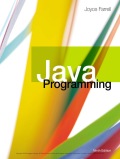
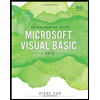
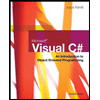