Creating a JAVA program which should implement a method that verifies whether a given 4 x 4 square (containing 16 values) is a Magic Square with a given magicValue. Also, adding code to generate values for a Magic Square based on a magicValue. The code here is good to solve for verifying if it is a magic square but not n generating a new Magic Square, it is not as in the picture. The sum in row and columns are not equal. PLEASE, PLEASE, PLEASE, don't post code from anywhere else. Please help me by working on this code here. import java.util.*; import java.awt.*; public class MagicSquare{ static int checkMag(int mat[][],int M) { // Function for checking Magic square int i, j,n=4; int sum=0; // filling matrix with its count value // starting from 1; for ( i = 0; i < n; i++) { for ( j = 0; j < n; j++) sum=sum+mat[i][j]; if(sum!=M) { return 0; } sum=0; } for ( i = 0; i < n; i++) { for ( j = 0; j < n; j++) sum=sum+mat[j][i]; if(sum!=M) { return 0; } sum=0; } int sumR=0, sumL=0; for ( i = 0; i < n; i++) { for ( j = 0; j < n; j++) { if(i==j) sumL=sumL+mat[i][j]; if((i+j)==(n-1)) sumR=sumR+mat[i][j]; } } if(sumR!=M ) { return 0; } if(sumL!=M) { return 0; } return 1; } static int[][] builtMagic(int mat[][],int M,int Mnew) { int i,j,n=4; Mnew=Mnew-M; int rem=Mnew%4; int quo =Mnew/4; if(rem==0){ for ( i = 0; i < n; i++) { for ( j = 0; j < n; j++) mat[i][j] =mat[i][j]+quo; }} else{ for ( i = 0; i < n; i++) { for ( j = 0; j < n; j++) { mat[i][j] =mat[i][j]+quo; if(mat[i][j]==13||mat[i][j]==14||mat[i][j]==15||mat[i][j]==16) { mat[i][j] =mat[i][j]+rem; } } } } return mat; } public static void main (String[] args) { //Scanner object Scanner sc = new Scanner(System.in); DrawingPanel panel = new DrawingPanel(500, 500); Graphics g = panel.getGraphics(); // creating a 2d array of magic square numbers int numbers[][] = { { 8, 11, 14, 1 }, { 13, 2, 7, 12 }, { 3, 16, 9, 6 }, { 10, 5, 4, 15 } }; // drawing grid of cell width = 80 centered at panel center drawGrid(g, panel.getWidth() / 2, panel.getHeight() / 2,numbers.length, 80); //drawNumbers(g, panel.getWidth() / 2, panel.getHeight() / 2, 80, numbers); // drawing title string centered at y=50 drawString(g, "CSC 142 Magic Square", panel.getWidth() / 2, 50); int n = 4; int magMat[][]= new int[n][n]; int M; int baseMat[][]={{12,6,15,1},{13,3,10,8},{2,16,5,11},{7,9,4,14}}; for (int k=0;k<10;k++){ System.out.println("Enter magic value"); M=sc.nextInt(); System.out.println( "Enter " + ( n*n ) + " values" ); for(int i=0;i
QUESTION: Creating a JAVA program which should implement a method that verifies whether a given 4 x 4 square (containing 16 values) is a Magic Square with a given magicValue. Also, adding code to generate values for a Magic Square based on a magicValue.
The code here is good to solve for verifying if it is a magic square but not n generating a new Magic Square, it is not as in the picture. The sum in row and columns are not equal.
PLEASE, PLEASE, PLEASE, don't post code from anywhere else. Please help me by working on this code here.
import java.util.*;
import java.awt.*;
public class MagicSquare{
static int checkMag(int mat[][],int M)
{
// Function for checking Magic square
int i, j,n=4; int sum=0; // filling matrix with its count value // starting from 1;
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++)
sum=sum+mat[i][j];
if(sum!=M)
{
return 0;
}
sum=0;
}
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++)
sum=sum+mat[j][i];
if(sum!=M)
{
return 0;
}
sum=0;
}
int sumR=0,
sumL=0;
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++) {
if(i==j) sumL=sumL+mat[i][j];
if((i+j)==(n-1))
sumR=sumR+mat[i][j]; } }
if(sumR!=M )
{
return 0;
}
if(sumL!=M)
{
return 0;
}
return 1;
}
static int[][] builtMagic(int mat[][],int M,int Mnew) {
int i,j,n=4;
Mnew=Mnew-M;
int rem=Mnew%4;
int quo =Mnew/4;
if(rem==0){ for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++)
mat[i][j] =mat[i][j]+quo; }}
else{
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++) {
mat[i][j] =mat[i][j]+quo;
if(mat[i][j]==13||mat[i][j]==14||mat[i][j]==15||mat[i][j]==16) {
mat[i][j] =mat[i][j]+rem;
}
}
}
}
return mat;
}
public static void main (String[] args) {
//Scanner object
Scanner sc = new Scanner(System.in);
DrawingPanel panel = new DrawingPanel(500, 500);
Graphics g = panel.getGraphics();
// creating a 2d array of magic square numbers
int numbers[][] = { { 8, 11, 14, 1 }, { 13, 2, 7, 12 }, { 3, 16, 9, 6 }, { 10, 5, 4, 15 } };
// drawing grid of cell width = 80 centered at panel center
drawGrid(g, panel.getWidth() / 2, panel.getHeight() / 2,numbers.length, 80);
//drawNumbers(g, panel.getWidth() / 2, panel.getHeight() / 2, 80, numbers);
// drawing title string centered at y=50
drawString(g, "CSC 142 Magic Square", panel.getWidth() / 2, 50);
int n = 4;
int magMat[][]= new int[n][n];
int M;
int baseMat[][]={{12,6,15,1},{13,3,10,8},{2,16,5,11},{7,9,4,14}};
for (int k=0;k<10;k++){
System.out.println("Enter magic value");
M=sc.nextInt();
System.out.println( "Enter " + ( n*n ) + " values" );
for(int i=0;i<n;i++) {
for(int j=0;j<n;j++)
{
magMat[i][j]= sc.nextInt();
}
}
if(checkMag(magMat,M)==1) {
System.out.println("It is a magic square");
panel.clear();
makeMagicSquareGrid(panel,magMat,k+1); // insert magic m=numbers on grid
}
else {
System.out.println("It is not a magic square"); }
System.out.println("Enter another magic value greater than 34");
int Mnew=sc.nextInt();
magMat=builtMagic(magMat,34,Mnew);
panel.clear();
makeMagicSquareGrid(panel,magMat,k+1); // insert magic m=numbers on grid
}
}
static void makeMagicSquareGrid(DrawingPanel dp,int[][] magMat, int k){
Graphics g = dp.getGraphics();
drawGrid(g, dp.getWidth() / 2, dp.getHeight() / 2,magMat.length, 80);
// drawing numbers
drawNumbers(g, dp.getWidth() / 2, dp.getHeight() / 2, 80, magMat);
// drawing title string centered at y=50
drawString(g, "Magic Square "+k, dp.getWidth() / 2, 50);
String filename="MagicMatrix"+k+".jpeg";
try{
dp.save(filename);
}
catch(Exception e)
{
System.out.println("File coudn't be saved");
}
}
public static void drawGrid(Graphics g, int centerX, int centerY, int row, int cell) { // cell width
int x = centerX - (row / 2) * cell;
int y = centerY - (row / 2) * cell;
// setting black color
g.setColor(Color.BLACK);
// looping for each row and column
for (int i = 0; i < row; i++) {
for (int j = 0; j < row; j++) {
// drawing a square with equal sides
g.drawRect(x + (cell * j), y + (cell * i),cell, cell);
}
}
}
// method to draw the numbers (given in a 2d array) inside a grid (assuming
// grid is previously drawn)
public static void drawNumbers(Graphics g, int centerX, int centerY,int cell, int numbers[][]) {
int x = centerX - (numbers.length / 2) * cell;
int y = centerY - (numbers.length / 2) * cell; //Setting red color
g.setColor(Color.RED); // Customizing font
g.setFont(new Font("Georgia", Font.BOLD, 25));
for (int i = 0; i < numbers.length; i++) {
for (int j = 0; j < numbers.length; j++) {
// Setting center cell
int xx = x + (cell * j) + cell / 2;
int yy = y + (cell * i) + cell / 2;
// Setting center text
drawString(g, String.valueOf(numbers[i][j]), xx, yy);
}
}
} public static void drawString(Graphics g, String text, int centerX, int centerY) {
// getting FontMetrics of current font
FontMetrics metrics = g.getFontMetrics(g.getFont());
// finding width of text in pixels using metrics
int width = metrics.stringWidth(text);
// drawing string center aligned
g.drawString(text, centerX - width / 2, centerY + metrics.getHeight()/ 2);
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

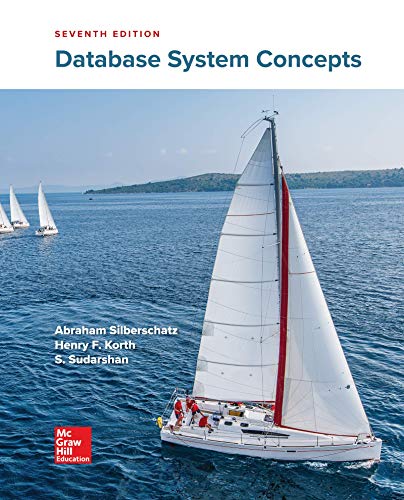
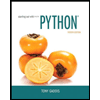
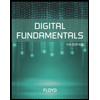
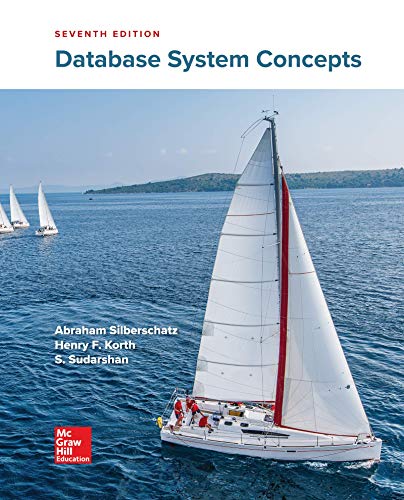
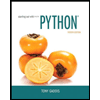
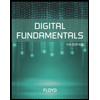
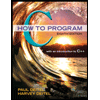
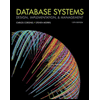
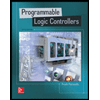