creating tree containers: one that uses a vector to hold your trees (class VectorContainer). Each of these container classes should be able to hold any amount of different expressions each of which can be of any size. see example. Please create vectorContainer.hpp all I need sort.hpp #ifndef __SORT_HPP__ #define __SORT_HPP__ #include "container.hpp" class Container; class Sort { public: /* Constructors */ Sort(); /* Pure Virtual Functions */ virtual void sort(Container* container) = 0; }; #endif //__SORT_HPP__ base.hpp #ifndef __BASE_HPP__ #define __BASE_HPP__
creating tree containers: one that uses a
Please create vectorContainer.hpp all I need
sort.hpp
#ifndef __SORT_HPP__
#define __SORT_HPP__
#include "container.hpp"
class Container;
class Sort {
public:
/* Constructors */
Sort();
/* Pure Virtual Functions */
virtual void sort(Container* container) = 0;
};
#endif //__SORT_HPP__
base.hpp
#ifndef __BASE_HPP__
#define __BASE_HPP__
#include <string>
class Base {
public:
/* Constructors */
Base() { };
/* Pure Virtual Functions */
virtual double evaluate() = 0;
virtual std::string stringify() = 0;
};
#endif //__BASE_HPP__
container.hpp
#ifndef __CONTAINER_HPP__
#define __CONTAINER_HPP__
#include "sort.hpp"
#include "base.hpp"
class Sort;
class Base;
class Container {
protected:
Sort* sort_function;
public:
/* Constructors */
Container() : sort_function(nullptr) { };
Container(Sort* function) : sort_function(function) { };
/* Non Virtual Functions */
void set_sort_function(Sort* sort_function); // set the type of sorting
/* Pure Virtual Functions */
// push the top pointer of the tree into container
virtual void add_element(Base* element) = 0;
// iterate through trees and output the expressions (use stringify())
virtual void print() = 0;
// calls on the previously set sorting-algorithm. Checks if sort_function is not null, throw exception if otherwise
virtual void sort() = 0;
/* Essentially the only functions needed to sort */
//switch tree locations
virtual void swap(int i, int j) = 0;
// get top ptr of tree at index i
virtual Base* at(int i) = 0;
// return container size
virtual int size() = 0;
};
#endif //__CONTAINER_HPP__
Example
#ifndef __LISTCONTAINER_HPP__
#define __LISTCONTAINER_HPP__
#include "container.hpp"
#include <list>
#include <iostream>
#include <stdexcept>
class Sort;
class ListContainer: public Container{
public:
std::list<Base*> baseList;
//Container() : sort_function(nullptr){};
//Container(Sort* function) : sort_Function(function){};
//void set_sort_funtion(Sort* sort_function){
// this -> sort_function = sort_function;
//}
void add_element(Base* element){
baseList.push_back(element);
}
void print(){
for(std::list<Base*>::iterator i = baseList.begin(); i != baseList.end(); ++i){
if(i == baseList.begin()){
std::cout <<(*i) -> stringify();
}
else{
std::cout << ", " << (*i) -> stringify();
}
}
std::cout << std::endl;
}
void sort(){
try{
if(sort_function != nullptr){
sort_function -> sort(this);
}
else{
throw std::logic_error("invalid sort_function");
}
}
catch(std::exception &exp){
std::cout << "ERROR : " << exp.what() << "\n";
}
}
//sorting functions
void swap(int i, int j){
std::list<Base*>::iterator first = baseList.begin();
for(int f = 0; f < i; f++){
first++;
}
Base* temp = *first;
std::list<Base*>::iterator second = baseList.begin();
for(int s = 0; s < j; s++){
second++;
}
*first = *second;
*second = temp;
}
Base* at(int i){
std::list<Base*>::iterator x = baseList.begin();
for(int a = 0; a < i; a++){
x++;
}
return *x;
}
int size(){
return baseList.size();
}
};
#endif //__LISTCONTAINER_HPP__

An iterator is an object (like a pointer) that points to an element inside the container.
We can use iterators to move through the contents of the container.
They can be visualized as something similar to a pointer pointing to some location and we can access the content at that particular location using them
Iterators play a critical role in connecting algorithm with containers along with the manipulation of data stored inside the containers. The most obvious form of an iterator is a pointer.
A pointer can point to elements in an array and can iterate through them using the increment operator (++). But, all iterators do not have similar functionality as that of pointers
Depending upon the functionality of iterators they can be classified into five categories, as shown in the diagram below with the outer one being the most powerful one and consequently the inner one is the least powerful in terms of functionality.
Input Iterators:
They are the weakest of all the iterators and have very limited functionality. They can only be used in a single-pass algorithms, i.e., those algorithms which process the container sequentially, such that no element is accessed more than once.
Output Iterators:
Just like input iterators, they are also very limited in their functionality and can only be used in single-pass algorithm, but not for accessing elements, but for being assigned elements.
Forward Iterator:
They are higher in the hierarachy than input and output iterators, and contain all the features present in these two iterators. But, as the name suggests, they also can only move in a forward direction and that too one step at a time.
Bidirectional Iterators:
They have all the features of forward iterators along with the fact that they overcome the drawback of forward iterators, as they can move in both the directions, that is why their name is bidirectional.
Random-Access Iterators:
They are the most powerful iterators. They are not limited to moving sequentially, as their name suggests, they can randomly access any element inside the container. They are the ones whose functionality are same as pointers.
sort.hpp
#ifndef __SORT_HPP__
#define __SORT_HPP__
#include "container.hpp"
class Container;
class Sort {
public:
/* Constructors */
Sort();
/* Pure Virtual Functions */
virtual void sort(Container* container) = 0;
};
#endif //__SORT_HPP__
c++
class Sort {
public:
/* Constructors */
Sort();
/* Pure Virtual Functions */
virtual void sort(Container* container) = 0;
};
#ifndef __BASE_HPP__
#define __BASE_HPP__
#include <string>
class Base {
public:
/* Constructors */
Base() { };
/* Pure Virtual Functions */
virtual double evaluate() = 0;
virtual std::string stringify() = 0;
};
#endif //__BASE_HPP__
```c++
class Container {
protected:
Sort* sort_function;
public:
/* Constructors */
Container() : sort_function(nullptr) { };
Container(Sort* function) : sort_function(function) { };
/* Non Virtual Functions */
void set_sort_function(Sort* sort_function); // set the type of sorting algorithm
/* Pure Virtual Functions */
// push the top pointer of the tree into container
virtual void add_element(Base* element) = 0;
// iterate through trees and output the expressions (use stringify())
virtual void print() = 0;
// calls on the previously set sorting-algorithm. Checks if sort_function is not null, throw exception if otherwise
virtual void sort() = 0;
/* Functions Needed to Sort */
//switch tree locations
virtual void swap(int i, int j) = 0;
// get top ptr of tree at index i
virtual Base* at(int i) = 0;
// return container size
virtual int size() = 0;
};
```
Trending now
This is a popular solution!
Step by step
Solved in 5 steps

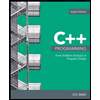
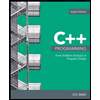