def substitution(plainText, key): Returns encrypted string for the plainText using substitution encryption, which is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute 'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'. Leave all other non-alpha characters as it is in plainText. Your algorithm should be efficient so that it can run fairly quickly for very large strings. 1. use replace() to convert each letter to its encrypted letter 2. append encrypted letter to a list '.join(list_of_letters) to form the final string. Do not use + to do string concatenation to form the encrypted string. return plainText def run(): II II II 1. Prompt user for a key; 2. Check if key is valid. If not, print an error message then return; if valid, prompt for a plain text to be encrypted with the valid key, 3. Send the plain text and valid key to substitution function. 4. Print out the encrypted message. key = input("Enter a 26 letter key: ") if not isValidKey(key): print("Invalid key.") return plainText = input("Plain Text: ") cipherText = substitution(plainText, key) print(f"Cipher Text: {cipherText}") return if "-_main__": %3D _name__ run()
def substitution(plainText, key): Returns encrypted string for the plainText using substitution encryption, which is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute 'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'. Leave all other non-alpha characters as it is in plainText. Your algorithm should be efficient so that it can run fairly quickly for very large strings. 1. use replace() to convert each letter to its encrypted letter 2. append encrypted letter to a list '.join(list_of_letters) to form the final string. Do not use + to do string concatenation to form the encrypted string. return plainText def run(): II II II 1. Prompt user for a key; 2. Check if key is valid. If not, print an error message then return; if valid, prompt for a plain text to be encrypted with the valid key, 3. Send the plain text and valid key to substitution function. 4. Print out the encrypted message. key = input("Enter a 26 letter key: ") if not isValidKey(key): print("Invalid key.") return plainText = input("Plain Text: ") cipherText = substitution(plainText, key) print(f"Cipher Text: {cipherText}") return if "-_main__": %3D _name__ run()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
python program. Instructions are written within the quotation marks of how to define a function.
![def substitution(plainText, key):
II II ||
Returns encrypted string for the plainText using substitution encryption, which
is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute
'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'.
Leave all other non-alpha characters as it is in plainText.
Your algorithm should be efficient so that it can run fairly quickly for very
large strings.
1. use replace() to convert each letter to its encrypted letter
2. append encrypted letter to a list
3. use ''.join(list_of_letters) to form the final string. Do not use + to do
string concatenation to form the encrypted string.
II II ||
return plainText
def run():
1. Prompt user for a key;
2. Check if key is valid. If not, print an error message then return;
if valid, prompt for a plain text to be encrypted with the valid key,
3. Send the plain text and valid key to substitution function.
4. Print out the encrypted message.
II II||
input("Enter a 26 letter key: ")
key
if not isValidKey(key):
print("Invalid key.")
return
plainText
cipherText = substitution(plainText, key)
print(f"Cipher Text: {cipherText}")
input("Plain Text: ")
return
if
-_name_.
__main__":
run()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe489eec6-06b4-4f41-8536-03174f230880%2F1f72c020-4c4e-44b9-add6-d571da9eb8fa%2F6aymkfa_processed.png&w=3840&q=75)
Transcribed Image Text:def substitution(plainText, key):
II II ||
Returns encrypted string for the plainText using substitution encryption, which
is to substitute 'a'/'A' with the lower/upper case of key[0], and substitute
'b'/'B' with the lower/upper case of key[1], and so on all the way to 'z'/'Z'.
Leave all other non-alpha characters as it is in plainText.
Your algorithm should be efficient so that it can run fairly quickly for very
large strings.
1. use replace() to convert each letter to its encrypted letter
2. append encrypted letter to a list
3. use ''.join(list_of_letters) to form the final string. Do not use + to do
string concatenation to form the encrypted string.
II II ||
return plainText
def run():
1. Prompt user for a key;
2. Check if key is valid. If not, print an error message then return;
if valid, prompt for a plain text to be encrypted with the valid key,
3. Send the plain text and valid key to substitution function.
4. Print out the encrypted message.
II II||
input("Enter a 26 letter key: ")
key
if not isValidKey(key):
print("Invalid key.")
return
plainText
cipherText = substitution(plainText, key)
print(f"Cipher Text: {cipherText}")
input("Plain Text: ")
return
if
-_name_.
__main__":
run()

Transcribed Image Text:• The run() function is implemented for you already that will get user to enter a key, call a function you
implemented to check the validity of the key and proceed to get plainText, encrypt with the key and print out the
cipherText.
• The isValidKey() function will check If the key is invalid (as by not containing 26 characters, containing any
character that is not an alphabetic character, or not containing each letter exactly once) and return False if it is
not valid and return True if it is valid.
• Your substitution() function will generate a cipherText from plainText using a valid key. The cipherText is obtained
by replacing each alphabetical character in the plainText substituted for the corresponding character in the
ciphertext; non-alphabetical characters should be left unchanged. You should first implement the replace()
function that do the substitution for one letter, and then map the replace() function to every letter in the
plainText.
• Your program must preserve case: capitalized letters must remain capitalized letters; lowercase letters must
remain lowercase letters.
Here is your starter code in hw6.py:
# Author: Yanling Wang yuw17@psu.edu
def isValidKey(key):
II II ||
Returns True if key is a string that has 26 characters and each of the letter
'a'/'A'-'z'/'Z' appeared once and only once in either lower case or upper case.
Returns False otherwise.
II II ||
return True
def replace(letter, key):
II II ||
Assume letter is a single characer string.
Replace a single letter with its corresponding key, returns letter if it is
not in the alphabet 'a'-'z' or 'A'-'Z'
II II ||
return letter
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
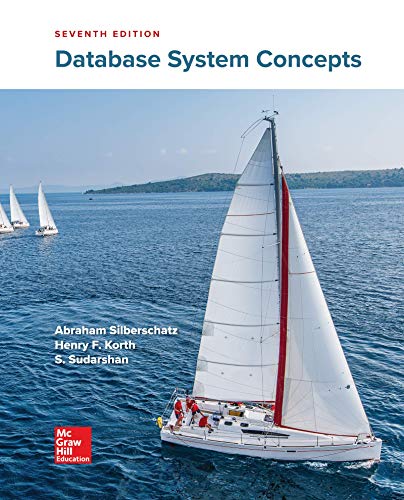
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
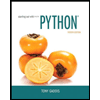
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
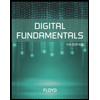
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
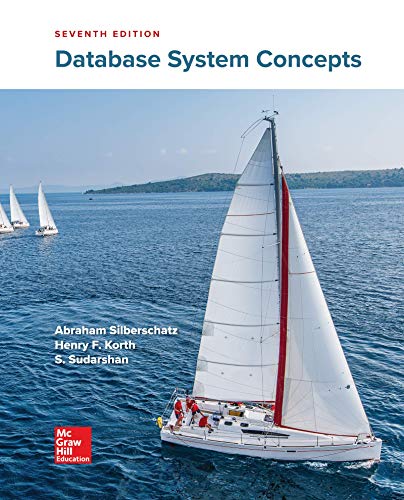
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
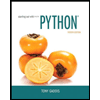
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
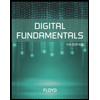
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
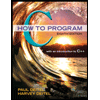
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
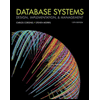
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
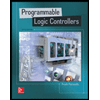
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education