Define the Artist class with a constructor to initialize an artist's information and a print_info() method. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0. print_info() should display Artist Name, born XXXX if the year of death is -1 or Artist Name (XXXX-YYYY) otherwise. Define the Artwork class with a constructor to initialize an artwork's information and a print_info() method. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values. Ex: If the input is: Pablo Picasso 1881 1973 Three Musicians 1921 the output is: Artist: Pablo Picasso (1881-1973) Title: Three Musicians, 1921 If the input is: Brice Marden 1938 -1 Distant Muses 2000 the output is:
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
PYTHON:
Define the Artist class with a constructor to initialize an artist's information and a print_info() method. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0. print_info() should display Artist Name, born XXXX if the year of death is -1 or Artist Name (XXXX-YYYY) otherwise.
Define the Artwork class with a constructor to initialize an artwork's information and a print_info() method. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values.
Ex: If the input is:
Pablo Picasso 1881 1973 Three Musicians 1921
the output is:
Artist: Pablo Picasso (1881-1973) Title: Three Musicians, 1921
If the input is:
Brice Marden 1938 -1 Distant Muses 2000
the output is:
Artist: Brice Marden, born 1938 Title: Distant Muses, 2000
I have the code but I am getting errors that I can't seem to get rid of any suggestions would be appreciated



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

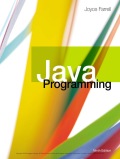
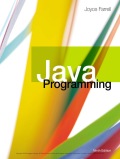