Develop flowchart for this algorithm, The algorithm takes as input a search key and an array, output "key in arr: YES" or "key in arr: NO" Hint: use loop and two variables, one to store the starting index of the current search subarray and another to store the end of the current search subarray. These variables are often called start, end, low, high, etc. Let's call it L and H. In each iteration we search array from index L to H. Initially L is0 and H is the last index of the original array. In each iteration of the loop, we get middle index M and middle value. If the search key is smaller than the middle values, search 1st half of the array by setting
Develop flowchart for this algorithm, The algorithm takes as input a search key and an array, output "key in arr: YES" or "key in arr: NO" Hint: use loop and two variables, one to store the starting index of the current search subarray and another to store the end of the current search subarray. These variables are often called start, end, low, high, etc. Let's call it L and H. In each iteration we search array from index L to H. Initially L is0 and H is the last index of the original array. In each iteration of the loop, we get middle index M and middle value. If the search key is smaller than the middle values, search 1st half of the array by setting
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Problem 2
In this problem, develop an algorithm to search a key in a sorted array.
As shown in class, one general way to search a key in an array is to scan the array, comparing each element against
the search key, until one element matches the key, or no match is found till the end of the array. In the latter case the
algorithm concludes that the key is not in the array.
when the array is sorted, we can search in a more efficient way. Assume the array is sorted in ascending order. The
idea is, we retrieve the middle value from the array and compare it against the search key. If the middle value and the
search key match, we’ve found it and the algorithm stops. If not so and the search key is smaller than the middle
value, we search the 1st (left) half of the array as the key could not be in the 2nd (right) half (convince yourself!),
otherwise -- the search key is larger than the middle value -- we search the 2nd half of the array as the key could not
be in the 1st half of the
array.
In the half sub-array, we repeat the above steps, retrieving the middle key of the sub-array, comparing it against the
search key. If they match, we've found it and stop. If they are not same, then go to either first half or second half of
the subarray based on the comparison result.
The search stops either when a match is found, or there is no subarray to search. In the latter case the algorithm
concludes that the key is not in the array. Following figure shows the steps of searching 38 in a sorted array.
0 1 2 3
4 5
7 8 9
10
Search 38
02
05
08
12
16
23
38
56
72
91
97
|L= 0 1 2
3
M= 5
7 8
H = 10
38 > 23
take 2nd Half
02
05
08
12.
16
23
38
56
72
91
97
1st Half
2nd Half
5 6
1
2
3
4
M= 8
H = 10
%3D
38 < 72
take 1st half
02
05
08
12 16
23
38
56
72
91
97
Discarded
1st Half
2nd Half
L=6
M = 6
23
0 1 2
3
4 5
H = 7
8 9
10
Found 38,
Return 6
05
02
08
12
16
38
56
72
91
97
4.
![Develop flowchart for this algorithm,
The algorithm takes as input a search key and an array, output “key in ar: YES" or "key in arr: NO"
Hint: use loop and two variables, one to store the starting index of the current search subarray and
another to store the end of the current search subarray. These variables are often called start, end, low,
high, etc. Let's call it L and H. In each iteration we search array from index L to H. Initially L is O
and H is the last index of the original array. In each iteration of the loop, we get middle index M and
middle value. If the search key is smaller than the middle values, search 1st half of the array by setting
H to be M-1 (L does not change). If need to search 2nd half, set L to be M+1 (H does not change).
If the search (sub)array is of even size, the middle index can be either the end of the 1st half or the
beginning of the 2nd half (so to be precise, the "middle" element should be called "middle-most"
element).
The loop should stop immediately when the key is found.
What is the terminating condition of the search loop?
Must use loops. You should not use recursions. (We will learn recursion later).
Start your flowchart as shown in the following figure. The algorithm takes as input an array arr and a
search key key
Save the flowchart as img_02.jpg
start
arr[]
key
Implement the algorithm using JavaScript
Complete the function problem20, and attach the onclick event to the corresponding button in html
Your implementation should match your flowchart.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdac761a6-ba1c-4b50-93cd-f82ea0746880%2F6cb61855-8e1c-4759-977f-724f78057e50%2Fvm5c4of_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Develop flowchart for this algorithm,
The algorithm takes as input a search key and an array, output “key in ar: YES" or "key in arr: NO"
Hint: use loop and two variables, one to store the starting index of the current search subarray and
another to store the end of the current search subarray. These variables are often called start, end, low,
high, etc. Let's call it L and H. In each iteration we search array from index L to H. Initially L is O
and H is the last index of the original array. In each iteration of the loop, we get middle index M and
middle value. If the search key is smaller than the middle values, search 1st half of the array by setting
H to be M-1 (L does not change). If need to search 2nd half, set L to be M+1 (H does not change).
If the search (sub)array is of even size, the middle index can be either the end of the 1st half or the
beginning of the 2nd half (so to be precise, the "middle" element should be called "middle-most"
element).
The loop should stop immediately when the key is found.
What is the terminating condition of the search loop?
Must use loops. You should not use recursions. (We will learn recursion later).
Start your flowchart as shown in the following figure. The algorithm takes as input an array arr and a
search key key
Save the flowchart as img_02.jpg
start
arr[]
key
Implement the algorithm using JavaScript
Complete the function problem20, and attach the onclick event to the corresponding button in html
Your implementation should match your flowchart.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
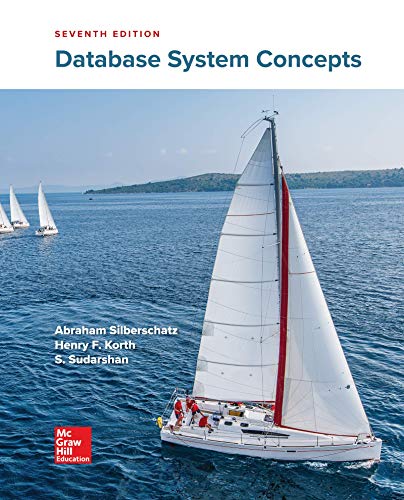
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
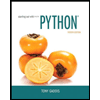
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
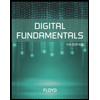
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
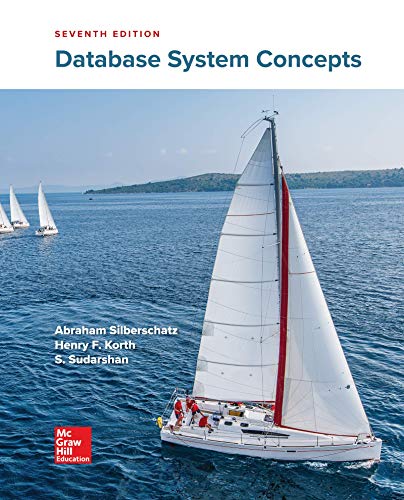
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
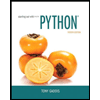
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
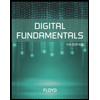
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
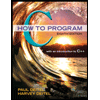
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
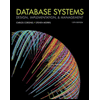
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
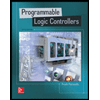
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education