Draw a UML class diagram for the following code: class Node { E data; Node next; public Node(E data) { this.data = data; this.next = null; } } class Queue { Node front; Node rear; public Queue() { front = null; rear = null; } public boolean isEmpty() { return front == null; } public void enqueue(E item) { Node newNode = new Node(item); if (isEmpty()) { front = newNode; rear = newNode; } else { rear.next = newNode; rear = newNode; } } public E dequeue() { if (isEmpty()) { System.out.println("Queue is empty."); return null; } else { E item = front.data; front = front.next; if (front == null) { rear = null; } return item; } } public void dequeueAll() { front = null; rear = null; } public E peek() { if (isEmpty()) { System.out.println("Queue is empty."); return null; } else { return front.data; } } } public class Main { public static void printQueue(Queue queue) { Node currentNode = queue.front; System.out.print("Queue: "); while (currentNode != null) { System.out.print(currentNode.data + " "); currentNode = currentNode.next; } System.out.println(); } public static void main(String[] args) { Queue queue = new Queue(); System.out.println("Is the queue empty? " + queue.isEmpty()); printQueue(queue); queue.enqueue(1); System.out.println("Enqueued: 1"); printQueue(queue); queue.enqueue(2); System.out.println("Enqueued: 2"); printQueue(queue); queue.enqueue(3); System.out.println("Enqueued: 3"); printQueue(queue); System.out.println("Is the queue empty? " + queue.isEmpty()); printQueue(queue); System.out.println("Front of the queue: " + queue.peek()); printQueue(queue); System.out.println("Dequeue: " + queue.dequeue()); printQueue(queue); System.out.println("Front of the queue: " + queue.peek()); printQueue(queue); queue.dequeueAll(); System.out.println("Dequeued all items"); printQueue(queue); System.out.println("Is the queue empty? " + queue.isEmpty()); } }
Draw a UML class diagram for the following code:
class Node<E> {
E data;
Node<E> next;
public Node(E data) {
this.data = data;
this.next = null;
}
}
class Queue<E> {
Node<E> front;
Node<E> rear;
public Queue() {
front = null;
rear = null;
}
public boolean isEmpty() {
return front == null;
}
public void enqueue(E item) {
Node<E> newNode = new Node<E>(item);
if (isEmpty()) {
front = newNode;
rear = newNode;
} else {
rear.next = newNode;
rear = newNode;
}
}
public E dequeue() {
if (isEmpty()) {
System.out.println("Queue is empty.");
return null;
} else {
E item = front.data;
front = front.next;
if (front == null) {
rear = null;
}
return item;
}
}
public void dequeueAll() {
front = null;
rear = null;
}
public E peek() {
if (isEmpty()) {
System.out.println("Queue is empty.");
return null;
} else {
return front.data;
}
}
}
public class Main {
public static void printQueue(Queue<Integer> queue) {
Node<Integer> currentNode = queue.front;
System.out.print("Queue: ");
while (currentNode != null) {
System.out.print(currentNode.data + " ");
currentNode = currentNode.next;
}
System.out.println();
}
public static void main(String[] args) {
Queue<Integer> queue = new Queue<Integer>();
System.out.println("Is the queue empty? " + queue.isEmpty());
printQueue(queue);
queue.enqueue(1);
System.out.println("Enqueued: 1");
printQueue(queue);
queue.enqueue(2);
System.out.println("Enqueued: 2");
printQueue(queue);
queue.enqueue(3);
System.out.println("Enqueued: 3");
printQueue(queue);
System.out.println("Is the queue empty? " + queue.isEmpty());
printQueue(queue);
System.out.println("Front of the queue: " + queue.peek());
printQueue(queue);
System.out.println("Dequeue: " + queue.dequeue());
printQueue(queue);
System.out.println("Front of the queue: " + queue.peek());
printQueue(queue);
queue.dequeueAll();
System.out.println("Dequeued all items");
printQueue(queue);
System.out.println("Is the queue empty? " + queue.isEmpty());
}
}

Step by step
Solved in 3 steps with 1 images

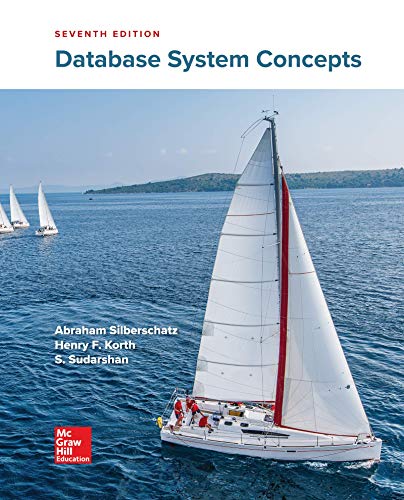
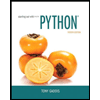
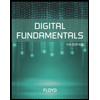
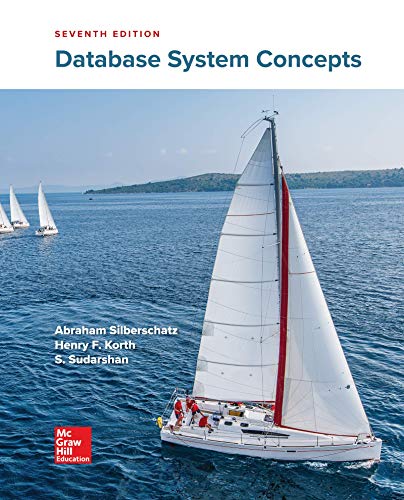
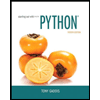
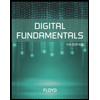
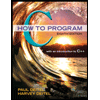
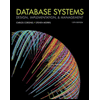
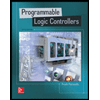