Extract the two words from the input string and remove any spaces. Store the strings in two separate variables and output the strings.
Question is to expand on current code.(
(3) Extract the two words from the input string and remove any spaces. Store the strings in two separate variables and output the strings.
Ex:
CURRENT PROGRAM:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAX_LIMIT 50
//Function definition
int Commacheck(char *input)
{
int flag = 0;
for(int i = 0; i < strlen(input); i++)
{
//if comma is present in the input entered by user, update the fag to 1.
if(input[i] == ',')
{
flag = 1;
break;
}
}
return flag;
}
//Main function
int main(void)
{
//varaiable initialization
char input[MAX_LIMIT];
char *word[2];
char delimiter[] = ", ";
printf("\n");
//get the input string from the user
printf("Enter input string: ");
fgets(input, MAX_LIMIT, stdin);
size_t ln = strlen(input) - 1;
//if no comma in the string entered by user
if(Commacheck(input) == 0)
{
printf("No comma in string.\n\n");
}
//When comma is present in the input string
else
{
char *ptr = strtok(input, delimiter);
int count = 0;
while(ptr != NULL)
{
word[count++] = ptr;
ptr = strtok(NULL, delimiter);
}
//print the first and second word of strings
printf("String 1: %s\n", word[0]);
printf("String 2: %s\n\n", word[1]);
}
return 0;
}

Step by step
Solved in 2 steps with 2 images

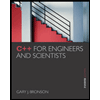
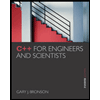