for (int i = 0; i < 12; i++) { string firstNote = notesArray[i]; string secondNote = notesArray[i + 4]; //determine thirdNote using the above information
for (int i = 0; i < 12; i++) { string firstNote = notesArray[i]; string secondNote = notesArray[i + 4]; //determine thirdNote using the above information
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help figuring this out and typing it in c++.
![for (int i = 0; i < 12; i++)
{
string firstNote = notesArray[i];
string secondNote = notesArray[i + 4];
//determine thirdNote using the above information
//concatenate first, second, and third notes (with tabs for readability) and add to triadsArray
triadsArray[i] = firstNote + "\t" + secondNote + "\t" + thirdNote;
}//end for loop
As a less straightforward chord example, suppose that you choose the root note G. In this case, we can "loop around"
the array declared above to find that the middle note of the triad is B. And the final note of the G major chord is D.
Note that this "loop around" operation can be implemented in arrays using the mod (%) operator. If we tried to use the
index in the code above for the second note of the G major chord (i + 4), we would generate an "out of bounds" error.
The index of G is 10, and 10 + 4 = 14, which is greater than the index of the final array element ("G#" is at position 11).
A similar problem would occur for the third note. I am happy to help, but try to resolve this problem out on your own. As
a hint, using %12 in the subscript operator would probably be the most straightforward approach.
The first 6 chords are shown in the image below. Generate these and the remaining 6 chords with your code.
E
F
F#
G
G#
A
A#
B
C
C#
D
C#
D
D#
E
F
F#
(Note for those familiar with music theory: the A# and C# chords are usually referred to as a B-flat and D-flat - ignore
this for simplicity in this program).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff4e702fc-6601-4bea-8fcb-104f0eaa22ba%2Fd69a4041-b7d5-4095-bcb4-892aed26824f%2F6z2sj7_processed.png&w=3840&q=75)
Transcribed Image Text:for (int i = 0; i < 12; i++)
{
string firstNote = notesArray[i];
string secondNote = notesArray[i + 4];
//determine thirdNote using the above information
//concatenate first, second, and third notes (with tabs for readability) and add to triadsArray
triadsArray[i] = firstNote + "\t" + secondNote + "\t" + thirdNote;
}//end for loop
As a less straightforward chord example, suppose that you choose the root note G. In this case, we can "loop around"
the array declared above to find that the middle note of the triad is B. And the final note of the G major chord is D.
Note that this "loop around" operation can be implemented in arrays using the mod (%) operator. If we tried to use the
index in the code above for the second note of the G major chord (i + 4), we would generate an "out of bounds" error.
The index of G is 10, and 10 + 4 = 14, which is greater than the index of the final array element ("G#" is at position 11).
A similar problem would occur for the third note. I am happy to help, but try to resolve this problem out on your own. As
a hint, using %12 in the subscript operator would probably be the most straightforward approach.
The first 6 chords are shown in the image below. Generate these and the remaining 6 chords with your code.
E
F
F#
G
G#
A
A#
B
C
C#
D
C#
D
D#
E
F
F#
(Note for those familiar with music theory: the A# and C# chords are usually referred to as a B-flat and D-flat - ignore
this for simplicity in this program).
![In traditional Western music, we use the following 12 note names to refer to pitches/frequencies:
A, A#, B, C, C#, D, D#, E, F, F#, G, G#
(The # symbol is read as "sharp" - there is an alternative listing of notes that uses "flat" notes, but let's just use sharps
for this assignment).
1)
Declare a 1D array to hold these 12 notes.
2)
Write code that loops through this array (could be named notesArray), prints the "major triads" (see below for definition)
of all 12 notes, and stores their values as strings in a separate array (could be named triadsArray).
A major triad (chord) can be defined as the following three notes:
i) a "root note" - this can be chosen as any of the 12 notes listed above
ii) the note 4 positions above the root note
iii) the note 7 positions above the root note
As an example, suppose that you choose the root note C. In this case, the note 4 positions above C is E. And the third
note of the triad is G.
Therefore the C major chord is given by the notes C, E, and G. (If the song "Kumbaya" is familiar to you, its first three
notes/syllables (Kum-bah-yah) make up a major chord.)
There is more than one approach to writing the code that generates the chords, but you might start with something like
the following:
string notesArray[] = {"A", "A#", ... "G#"};
string triadsArray[12]; //uninitialized - to be filled in with loop below
for (int i = 0; i < 12; i++)
{
string firstNote = notesArray[i];
string secondNote = notesArray[i + 4];
//determine thirdNote using the above information](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff4e702fc-6601-4bea-8fcb-104f0eaa22ba%2Fd69a4041-b7d5-4095-bcb4-892aed26824f%2F1fqhzfp_processed.png&w=3840&q=75)
Transcribed Image Text:In traditional Western music, we use the following 12 note names to refer to pitches/frequencies:
A, A#, B, C, C#, D, D#, E, F, F#, G, G#
(The # symbol is read as "sharp" - there is an alternative listing of notes that uses "flat" notes, but let's just use sharps
for this assignment).
1)
Declare a 1D array to hold these 12 notes.
2)
Write code that loops through this array (could be named notesArray), prints the "major triads" (see below for definition)
of all 12 notes, and stores their values as strings in a separate array (could be named triadsArray).
A major triad (chord) can be defined as the following three notes:
i) a "root note" - this can be chosen as any of the 12 notes listed above
ii) the note 4 positions above the root note
iii) the note 7 positions above the root note
As an example, suppose that you choose the root note C. In this case, the note 4 positions above C is E. And the third
note of the triad is G.
Therefore the C major chord is given by the notes C, E, and G. (If the song "Kumbaya" is familiar to you, its first three
notes/syllables (Kum-bah-yah) make up a major chord.)
There is more than one approach to writing the code that generates the chords, but you might start with something like
the following:
string notesArray[] = {"A", "A#", ... "G#"};
string triadsArray[12]; //uninitialized - to be filled in with loop below
for (int i = 0; i < 12; i++)
{
string firstNote = notesArray[i];
string secondNote = notesArray[i + 4];
//determine thirdNote using the above information
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
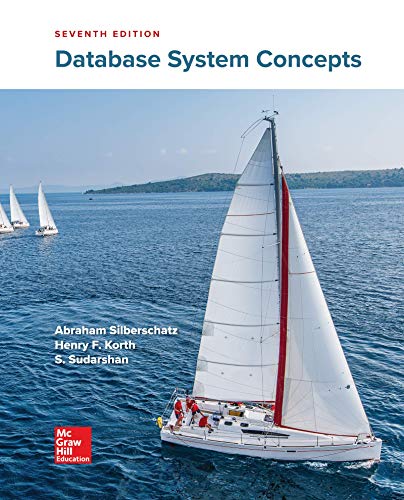
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
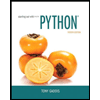
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
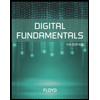
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
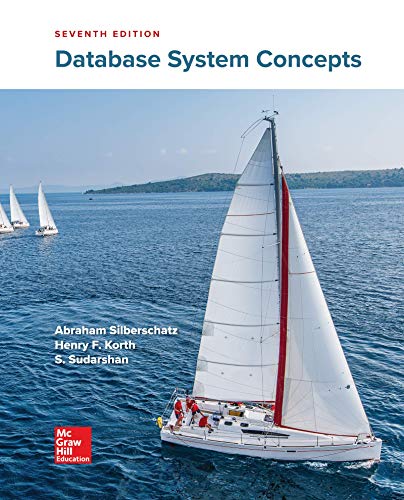
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
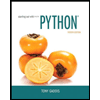
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
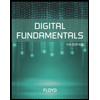
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
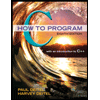
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
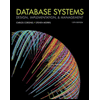
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
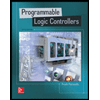
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education