Given a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList), that uses the printinfo() methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with-1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printinfo() method.
Given a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList), that uses the printinfo() methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with-1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printinfo() method.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java (ArrayList) - Plant Information
![Given a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be
able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList(), that uses the printinfo()
methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input
(ending with -1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printinfo() method.
Ex. If the input is:
plant Spirea 10
flower Hydrangea 30 false lilac.
flower Rose 6 false white
plant Mint 4
|-1
the output is:
Plant Information:
Plant Information:
Plant Information:
Plant name: Rose
Cost: 6
7
8
Plant Information:
Plant name: Mint
Cost: 4
9
18
11
1 import java.util.Scanner;
2 import java.util.ArrayList;
3 import java.util.StringTokenizer;
13
14
15
16
17
18
19
20
4
5 public class PlantArrayList Example {
// TODO: Define a printArrayList method that prints an ArrayList of plant (or flower) objects
public static void main(String[] args) {
Scanner scnr
new Scanner(System.in);
String input;
// TODO: Declare an ArrayList called myGarden that can hold object of type plant
// TODO: Declare variables - plantName, plant Cost, colorOfFlowers, isAnnual
21
22
23
27
29
Plant name: Spirea
Cost: 10
4
5
6
7
Plant name: Hydrangea
Cost: 30
9
10
11
Annual: false
Color of flowers: lilac
12
13.
14
15
16
Annual: false
Color of flowers: white
17
18
19
20
21
22
23
26
File is marked as read only
1 public class Plant
2 protected String plantName;
protected String plantCost;
input scnr.next();
while (!input.equals("-1")){
}
// TODO: Check if input is a plant or flower
//
11
Store as a plant object or flower object
Add to the ArrayList myGarden
input scnr.next();
}
Current PlantArrayListExample.java
file:
// TODO: COLL the method printArrayList to print myGarden
}
public void setPlantName(String userPlantName) {
plantName userPlantName;
public String getPlantName() {
return plantName;
public void setPlant Cost(String userPlantCost) {
plantCost userPlantCost;
public String getPlantCost() {
return plantCost;
Current file: Plant.java →
Load default template..
public void printInfo() {
System.out.println("Plant Information: ");
System.out.println(" Plant name:" + plantName);
System.out.println(" Cost: +plantCost);
File is marked as read only
1 public class Flower extends Plant
private boolean isAnnual;
private String colorOfFlowers;
3
7
8
9
10
11
12
13
14
15
16
17
19
20
21
22
24
25
26
27
28
29
30
public void setPlantType(boolean userIsAnnual) {
isAnnual userIsAnnual;
}
public boolean getPlantType(){
return isAnnual;
}
public void setColorOfFlowers (String userColorOfFlowers) {
colorOfFlowers = userColorOfFlowers;
}
Current file: Flower.java →
public String getColorOfFlowers() {
return colorOfFlowers;
}
@Override
public void printInfo(){
}
System.out.println("Plant Information: ");
System.out.println(" Plant name:" + plantName);
System.out.println("
Cost: + plantCost);
System.out.println(" Annual: " + isAnnual);
System.out.println(" Color of flowers: +colorOfFlowers);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd863c081-8226-42e9-a15c-9cd471d16ef3%2F40410bd2-789c-45b9-9736-85a840f5188b%2F0g5fzk_processed.png&w=3840&q=75)
Transcribed Image Text:Given a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be
able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList(), that uses the printinfo()
methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input
(ending with -1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printinfo() method.
Ex. If the input is:
plant Spirea 10
flower Hydrangea 30 false lilac.
flower Rose 6 false white
plant Mint 4
|-1
the output is:
Plant Information:
Plant Information:
Plant Information:
Plant name: Rose
Cost: 6
7
8
Plant Information:
Plant name: Mint
Cost: 4
9
18
11
1 import java.util.Scanner;
2 import java.util.ArrayList;
3 import java.util.StringTokenizer;
13
14
15
16
17
18
19
20
4
5 public class PlantArrayList Example {
// TODO: Define a printArrayList method that prints an ArrayList of plant (or flower) objects
public static void main(String[] args) {
Scanner scnr
new Scanner(System.in);
String input;
// TODO: Declare an ArrayList called myGarden that can hold object of type plant
// TODO: Declare variables - plantName, plant Cost, colorOfFlowers, isAnnual
21
22
23
27
29
Plant name: Spirea
Cost: 10
4
5
6
7
Plant name: Hydrangea
Cost: 30
9
10
11
Annual: false
Color of flowers: lilac
12
13.
14
15
16
Annual: false
Color of flowers: white
17
18
19
20
21
22
23
26
File is marked as read only
1 public class Plant
2 protected String plantName;
protected String plantCost;
input scnr.next();
while (!input.equals("-1")){
}
// TODO: Check if input is a plant or flower
//
11
Store as a plant object or flower object
Add to the ArrayList myGarden
input scnr.next();
}
Current PlantArrayListExample.java
file:
// TODO: COLL the method printArrayList to print myGarden
}
public void setPlantName(String userPlantName) {
plantName userPlantName;
public String getPlantName() {
return plantName;
public void setPlant Cost(String userPlantCost) {
plantCost userPlantCost;
public String getPlantCost() {
return plantCost;
Current file: Plant.java →
Load default template..
public void printInfo() {
System.out.println("Plant Information: ");
System.out.println(" Plant name:" + plantName);
System.out.println(" Cost: +plantCost);
File is marked as read only
1 public class Flower extends Plant
private boolean isAnnual;
private String colorOfFlowers;
3
7
8
9
10
11
12
13
14
15
16
17
19
20
21
22
24
25
26
27
28
29
30
public void setPlantType(boolean userIsAnnual) {
isAnnual userIsAnnual;
}
public boolean getPlantType(){
return isAnnual;
}
public void setColorOfFlowers (String userColorOfFlowers) {
colorOfFlowers = userColorOfFlowers;
}
Current file: Flower.java →
public String getColorOfFlowers() {
return colorOfFlowers;
}
@Override
public void printInfo(){
}
System.out.println("Plant Information: ");
System.out.println(" Plant name:" + plantName);
System.out.println("
Cost: + plantCost);
System.out.println(" Annual: " + isAnnual);
System.out.println(" Color of flowers: +colorOfFlowers);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
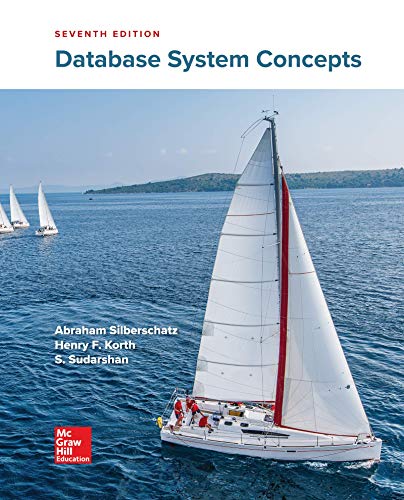
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
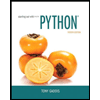
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
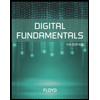
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
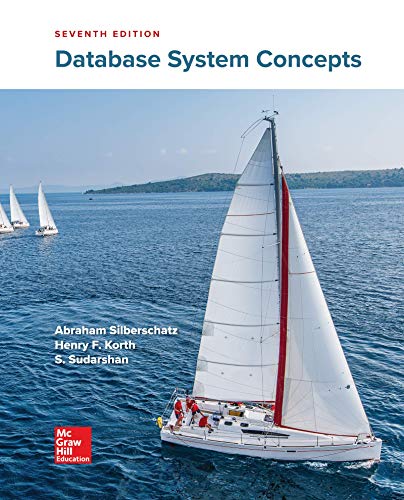
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
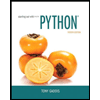
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
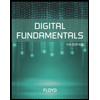
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
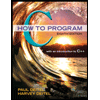
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
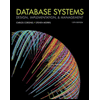
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
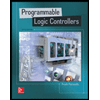
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education