Given a list of random numbers, compute the sum of these numbers. You may not use a for-loop to solve this problem. Starter Code: #include #include #include #include #include #include static int getRandomNumber(); template std::ostream& operator<<(std::ostream& out, std::vector list) { out << "["; } int main() { } for (const auto& val: list) { out << val << ", "; } out << "]"; return out; } std::vector list; std::generate_n(std::back_inserter (list), 20, []() { return getRandomNumber(); }); std::cout << list << "\n"; // TODO: compute the sum of the numbers in the list and print it out // NOTE: no for Loops, use algorithms static int getRandomNumber() { static std::mt19937 rng{ std::random_device{}() }; static std::uniform_int_distribution rand{ 0, 100 }; return rand(rng); Sample Output: [10, 1, 93, 97, 30, 60, 97, 65, 55, 99, 52, 5, 76, 85, 25, 49, 49, 37, 22, 8, 1 1015
Given a list of random numbers, compute the sum of these numbers. You may not use a for-loop to solve this problem. Starter Code: #include #include #include #include #include #include static int getRandomNumber(); template std::ostream& operator<<(std::ostream& out, std::vector list) { out << "["; } int main() { } for (const auto& val: list) { out << val << ", "; } out << "]"; return out; } std::vector list; std::generate_n(std::back_inserter (list), 20, []() { return getRandomNumber(); }); std::cout << list << "\n"; // TODO: compute the sum of the numbers in the list and print it out // NOTE: no for Loops, use algorithms static int getRandomNumber() { static std::mt19937 rng{ std::random_device{}() }; static std::uniform_int_distribution rand{ 0, 100 }; return rand(rng); Sample Output: [10, 1, 93, 97, 30, 60, 97, 65, 55, 99, 52, 5, 76, 85, 25, 49, 49, 37, 22, 8, 1 1015
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
![3 20 points
Given a list of random numbers, compute the sum of these numbers. You may not use a for-loop to solve this problem.
Starter Code:
#include <random>
#include <algorithm>
#include <iostream>
#include <unordered_set>
#include <numeric>
#include <vector>
static int getRandomNumber();
template<typename T> std::ostream& operator<<(std::ostream& out, std::vector<T> list) {
out << "[";
for (const auto& val: list) { out << val << ", "; }
out << "]";
return out;
}
int main() {
}
std::vector<int> list;
std::generate_n(std::back_inserter(list), 20, []() { return getRandomNumber(); });
std::cout << list << "\n";
}
// TODO: compute the sum of the numbers in the list and print it out
// NOTE: no for Loops, use algorithms
static int getRandomNumber() {
static std::mt19937 rng{ std::random_device{}() };
static std::uniform_int_distribution rand{ 0, 100 };
return rand(rng);
Sample Output:
[10, 1, 93, 97, 30, 60, 97, 65, 55, 99, 52, 5, 76, 85, 25, 49, 49, 37, 22, 8, 1
1015](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdad64e64-bab8-4c8e-9af1-b3316be12760%2F12381c35-583b-4487-af0d-75fe0cbbc80a%2Fow94dc9_processed.png&w=3840&q=75)
Transcribed Image Text:3 20 points
Given a list of random numbers, compute the sum of these numbers. You may not use a for-loop to solve this problem.
Starter Code:
#include <random>
#include <algorithm>
#include <iostream>
#include <unordered_set>
#include <numeric>
#include <vector>
static int getRandomNumber();
template<typename T> std::ostream& operator<<(std::ostream& out, std::vector<T> list) {
out << "[";
for (const auto& val: list) { out << val << ", "; }
out << "]";
return out;
}
int main() {
}
std::vector<int> list;
std::generate_n(std::back_inserter(list), 20, []() { return getRandomNumber(); });
std::cout << list << "\n";
}
// TODO: compute the sum of the numbers in the list and print it out
// NOTE: no for Loops, use algorithms
static int getRandomNumber() {
static std::mt19937 rng{ std::random_device{}() };
static std::uniform_int_distribution rand{ 0, 100 };
return rand(rng);
Sample Output:
[10, 1, 93, 97, 30, 60, 97, 65, 55, 99, 52, 5, 76, 85, 25, 49, 49, 37, 22, 8, 1
1015
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
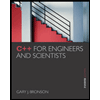
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
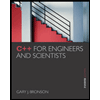
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr