Hi i need an implementation on the LinkListDriver and the LinkListOrdered for this output : please help me i need this to run Enter your choice: 1 Enter element: Mary List Menu Selections 1. add element 2_remove element 3.head element 4. display 5.Exit Enter your choice: 3 Element @ head: Mary List Menu Selections 1-add element 2-remove element 3_head element 4.display 5-Exit Enter your choice: 4 1. Mary List Menu Selections 1. add element 2. remove element 3.head element 4. display 5. Exit Enter your choice: LinkedListDriver package jsjf; import java.util.LinkedList; import java.util.Scanner; public class LinkedListDriver { public static void main(String [] args) { Scanner input = new Scanner(System.in); LinkedList list = new LinkedList(); int menu = 0; do { System.out.println("\nList menu selection\n1.Add element\n2.Remove element\n3.Head\n4.Display\n5.Exit"); System.out.println(); System.out.print("Enter your choice: "); menu = Integer.parseInt(input.next()); switch (menu) { case 1: System.out.print("Enter Element: "); String Element = input.next(); break; case 2: System.out.print("Enter Element: "); Element = input.next(); break; case 3: System.out.print("Enter Element: "); Element = input.next(); break; case 4: System.out.print("Enter Element: "); Element = input.next(); break; } } while (menu != 5); // iterates until 5 (Exit) is entered input.close(); } } Linear Node package jsjf; public class LinearNode { private LinearNode next; private E element; /** * Creates an empty node. */ public LinearNode() { next = null; element = null; } public LinearNode(E elem) { next = null; element = elem; } public LinearNode getNext() { return next; } /** * Sets the node that follows this one. public void setNext(LinearNode node) { next = node; } /** * Returns the element stored in this node. * * @return the element stored in this node */ public E getElement() { return element; } /** * Sets the element stored in this node. * * @param elem the element to be stored in this node */ public void setElement(E elem) { element = elem; } }
Hi i need an implementation on the LinkListDriver and the LinkListOrdered for this output :
please help me i need this to run
Enter your choice: 1
Enter element: Mary
List Menu Selections
1. add element
2_remove element
3.head element
4. display
5.Exit
Enter your choice: 3
Element @ head: Mary
List Menu Selections
1-add element
2-remove element
3_head element
4.display
5-Exit
Enter your choice:
4
1. Mary
List Menu Selections
1. add element
2. remove element
3.head element
4. display
5. Exit
Enter your choice:
LinkedListDriver
package jsjf;
import java.util.LinkedList;
import java.util.Scanner;
public class LinkedListDriver {
public static void main(String [] args)
{
Scanner input = new Scanner(System.in);
LinkedList<String> list = new LinkedList<String>();
int menu = 0;
do {
System.out.println("\nList menu selection\n1.Add element\n2.Remove element\n3.Head\n4.Display\n5.Exit");
System.out.println();
System.out.print("Enter your choice: ");
menu = Integer.parseInt(input.next());
switch (menu) {
case 1:
System.out.print("Enter Element: ");
String Element = input.next();
break;
case 2:
System.out.print("Enter Element: ");
Element = input.next();
break;
case 3:
System.out.print("Enter Element: ");
Element = input.next();
break;
case 4:
System.out.print("Enter Element: ");
Element = input.next();
break;
}
} while (menu != 5); // iterates until 5 (Exit) is entered
input.close();
}
}
Linear Node
package jsjf;
public class LinearNode<E>
{
private LinearNode<E> next;
private E element;
/**
* Creates an empty node.
*/
public LinearNode()
{
next = null;
element = null;
}
public LinearNode(E elem)
{
next = null;
element = elem;
}
public LinearNode<E> getNext()
{
return next;
}
/**
* Sets the node that follows this one.
public void setNext(LinearNode<E> node)
{
next = node;
}
/**
* Returns the element stored in this node.
*
* @return the element stored in this node
*/
public E getElement()
{
return element;
}
/**
* Sets the element stored in this node.
*
* @param elem the element to be stored in this node
*/
public void setElement(E elem)
{
element = elem;
}
}
LinkedOrderedList
package jsjf;
import java.util.Iterator;
import java.util.Spliterator;
import java.util.function.Consumer;
import jsjf.exceptions.*;
public class LinkedOrderedList<T> extends LinkedList<T>
implements OrderedListADT<T>
{
public LinkedOrderedList()
{
super();
}
public void add(T element, LinearNode<T> tail)
{
if (!(element instanceof Comparable))
{
throw new NonComparableElementException("LinkedOrderedList");
}
else
{
Comparable<T> comparableElement = (Comparable<T>)element;
LinearNode<T> head = null;
LinearNode<T> current = head;
LinearNode<T> previous = null;
LinearNode<T> newNode = new LinearNode<T>(element);
boolean found = false;
if (isEmpty()) // list is empty
{
head = newNode;
tail = newNode;
}
else if (comparableElement.compareTo(head.getElement()) <= 0)// element goes in front
{
newNode.setNext(head);
head = newNode;
}
else if (comparableElement.compareTo(tail.getElement()) >= 0)// element goes at tail
{
tail.setNext(newNode);
tail = newNode;
}
else // element goes in the middle
{
while ((comparableElement.compareTo(current.getElement()) > 0))
{
previous = current;
current = current.getNext();
}
newNode.setNext(current);
previous.setNext(newNode);
}
int count = 0;
count++;
int modCount = 0;
modCount++;
}
}
@Override
public T removeFirst() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public T removeLast() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public T remove(T element) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public T first() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public T last() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public boolean contains(T target) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public boolean isEmpty() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public int size() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public Iterator<T> iterator() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public void forEach(Consumer<? super T> action) {
OrderedListADT.super.forEach(action); //To change body of generated methods, choose Tools | Templates.
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

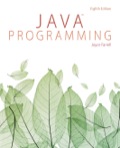
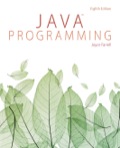