his is a project I am doing on Java but I can't get it to work somehow on Eclipse. Can you fix it and tell me the output? Thank you in advance! import java.util.Scanner; class RunningLog { static class Runner { String name; int numRuns; double[] distances; double[] times; Runner(String name, int numRuns) { this.name = name; this.numRuns = numRuns; this.distances = new double[numRuns]; this.times = new double[numRuns]; } } static void quickSort(Runner[] arr, int low, int high) { if (low < high) { int pi = partition(arr, low, high); quickSort(arr, low, pi - 1); quickSort(arr, pi + 1, high); } } static int partition(Runner[] arr, int low, int high) { String pivot = arr[high].name; int i = (low - 1); for (int j = low; j < high; j++) { if (arr[j].name.compareToIgnoreCase(pivot) < 0) { i++; Runner temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } Runner temp = arr[i + 1]; arr[i + 1] = arr[high]; arr[high] = temp; return i + 1; } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the number of runners: "); int numRunners = scanner.nextInt(); scanner.nextLine(); Runner[] runners = new Runner[numRunners]; for (int i = 0; i < numRunners; i++) { System.out.println("\nRunner " + (i + 1)); System.out.print("Enter the name of the runner: "); String name = scanner.nextLine(); System.out.print("Enter the number of runs: "); int numRuns = scanner.nextInt(); scanner.nextLine(); runners[i] = new Runner(name, numRuns); for (int j = 0; j < numRuns; j++) { System.out.println("\nRun " + (j + 1)); System.out.print("Enter the distance in miles (e.g., 3.1): "); runners[i].distances[j] = scanner.nextDouble(); System.out.print("Enter the time in minutes (e.g., 23.9): "); runners[i].times[j] = scanner.nextDouble(); } } quickSort(runners, 0, numRunners - 1); System.out.println("\nRunner Details:"); for (Runner runner : runners) { System.out.println(runner.name + " - Average Pace: " + calculateAveragePace(runner) + " minutes/mile"); } double totalPace = 0; int totalRuns = 0; for (Runner runner : runners) { for (int j = 0; j < runner.numRuns; j++) { totalPace += calculatePace(runner.distances[j], runner.times[j]); totalRuns++; } } double averagePaceAllRuns = totalPace / totalRuns; System.out.println("\nAverage Pace of All Runs: " + averagePaceAllRuns + " minutes/mile"); System.out.println("\nTotal Number of Runners: " + numRunners); System.out.println("Total Number of Runs: " + totalRuns); scanner.close(); } private static double calculatePace(double distance, double time) { return time / distance; } private static double calculateAveragePace(Runner runner) { double totalPace = 0; for (int i = 0; i < runner.numRuns; i++) { totalPace += calculatePace(runner.distances[i], runner.times[i]); } return totalPace / runner.numRuns;
his is a project I am doing on Java but I can't get it to work somehow on Eclipse. Can you fix it and tell me the output? Thank you in advance! import java.util.Scanner; class RunningLog { static class Runner { String name; int numRuns; double[] distances; double[] times; Runner(String name, int numRuns) { this.name = name; this.numRuns = numRuns; this.distances = new double[numRuns]; this.times = new double[numRuns]; } } static void quickSort(Runner[] arr, int low, int high) { if (low < high) { int pi = partition(arr, low, high); quickSort(arr, low, pi - 1); quickSort(arr, pi + 1, high); } } static int partition(Runner[] arr, int low, int high) { String pivot = arr[high].name; int i = (low - 1); for (int j = low; j < high; j++) { if (arr[j].name.compareToIgnoreCase(pivot) < 0) { i++; Runner temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } Runner temp = arr[i + 1]; arr[i + 1] = arr[high]; arr[high] = temp; return i + 1; } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the number of runners: "); int numRunners = scanner.nextInt(); scanner.nextLine(); Runner[] runners = new Runner[numRunners]; for (int i = 0; i < numRunners; i++) { System.out.println("\nRunner " + (i + 1)); System.out.print("Enter the name of the runner: "); String name = scanner.nextLine(); System.out.print("Enter the number of runs: "); int numRuns = scanner.nextInt(); scanner.nextLine(); runners[i] = new Runner(name, numRuns); for (int j = 0; j < numRuns; j++) { System.out.println("\nRun " + (j + 1)); System.out.print("Enter the distance in miles (e.g., 3.1): "); runners[i].distances[j] = scanner.nextDouble(); System.out.print("Enter the time in minutes (e.g., 23.9): "); runners[i].times[j] = scanner.nextDouble(); } } quickSort(runners, 0, numRunners - 1); System.out.println("\nRunner Details:"); for (Runner runner : runners) { System.out.println(runner.name + " - Average Pace: " + calculateAveragePace(runner) + " minutes/mile"); } double totalPace = 0; int totalRuns = 0; for (Runner runner : runners) { for (int j = 0; j < runner.numRuns; j++) { totalPace += calculatePace(runner.distances[j], runner.times[j]); totalRuns++; } } double averagePaceAllRuns = totalPace / totalRuns; System.out.println("\nAverage Pace of All Runs: " + averagePaceAllRuns + " minutes/mile"); System.out.println("\nTotal Number of Runners: " + numRunners); System.out.println("Total Number of Runs: " + totalRuns); scanner.close(); } private static double calculatePace(double distance, double time) { return time / distance; } private static double calculateAveragePace(Runner runner) { double totalPace = 0; for (int i = 0; i < runner.numRuns; i++) { totalPace += calculatePace(runner.distances[i], runner.times[i]); } return totalPace / runner.numRuns;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
This is a project I am doing on Java but I can't get it to work somehow on Eclipse. Can you fix it and tell me the output? Thank you in advance!
import java.util.Scanner;
class RunningLog
{
static class Runner
{
String name;
int numRuns;
double[] distances;
double[] times;
Runner(String name, int numRuns)
{
this.name = name;
this.numRuns = numRuns;
this.distances = new double[numRuns];
this.times = new double[numRuns];
}
}
static void quickSort(Runner[] arr, int low, int high)
{
if (low < high) { int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
static int partition(Runner[] arr, int low, int high)
{
String pivot = arr[high].name; int i = (low - 1);
for (int j = low; j < high; j++) { if (arr[j].name.compareToIgnoreCase(pivot) < 0)
{
i++;
Runner temp = arr[i]; arr[i] = arr[j]; arr[j] = temp;
}
} Runner temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the number of runners: ");
int numRunners = scanner.nextInt();
scanner.nextLine();
Runner[] runners = new Runner[numRunners];
for (int i = 0; i < numRunners; i++) {
System.out.println("\nRunner " + (i + 1));
System.out.print("Enter the name of the runner: ");
String name = scanner.nextLine();
System.out.print("Enter the number of runs: ");
int numRuns = scanner.nextInt();
scanner.nextLine();
runners[i] = new Runner(name, numRuns);
for (int j = 0; j < numRuns; j++)
{
System.out.println("\nRun " + (j + 1));
System.out.print("Enter the distance in miles (e.g., 3.1): ");
runners[i].distances[j] = scanner.nextDouble();
System.out.print("Enter the time in minutes (e.g., 23.9): ");
runners[i].times[j] = scanner.nextDouble();
}
}
quickSort(runners, 0, numRunners - 1);
System.out.println("\nRunner Details:");
for (Runner runner : runners)
{
System.out.println(runner.name + " - Average Pace: " + calculateAveragePace(runner) + " minutes/mile");
}
double totalPace = 0;
int totalRuns = 0; for (Runner runner : runners) { for (int j = 0; j < runner.numRuns; j++) { totalPace += calculatePace(runner.distances[j], runner.times[j]); totalRuns++;
}
}
double averagePaceAllRuns = totalPace / totalRuns;
System.out.println("\nAverage Pace of All Runs: " + averagePaceAllRuns + " minutes/mile");
System.out.println("\nTotal Number of Runners: " + numRunners);
System.out.println("Total Number of Runs: " + totalRuns);
scanner.close();
}
private static double calculatePace(double distance, double time)
{
return time / distance;
}
private static double calculateAveragePace(Runner runner)
{
double totalPace = 0;
for (int i = 0; i < runner.numRuns; i++)
{
totalPace += calculatePace(runner.distances[i], runner.times[i]);
}
return totalPace / runner.numRuns;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
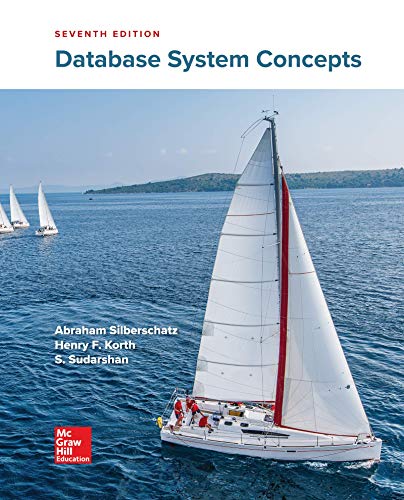
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
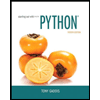
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
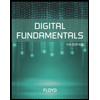
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
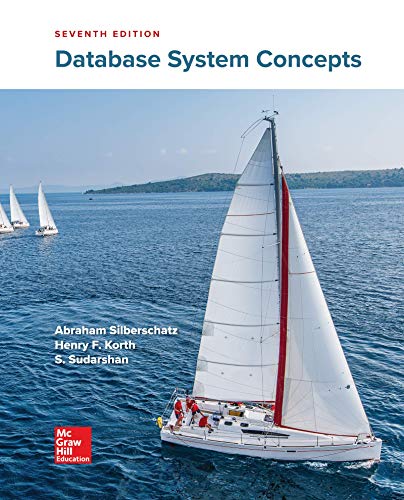
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
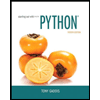
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
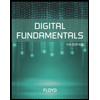
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
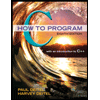
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
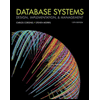
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
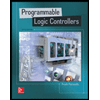
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education