How can i remove the extra spacing from my output while keeping the new line. This is my code: public class Money { private int dollar; private int cent; final static int MIN_CENT_VALUE = 0; final static int MAX_CENT_VALUE = 99; public Money() { this.dollar = 0; this.cent = 0; } public Money(int dollar, int cent) { this.dollar = dollar; if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) { this.cent = cent; } else { cent = 0; } } public int getDollar() { return this.dollar; } public void setDollar(int dol) { this.dollar = dol; } public int getCent() { return this.cent; } public void setCent(int c) { if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) { this.cent = c; } } publicMoneyadd(Money otherMoney) { Money m =newMoney(); m.dollar = this.dollar + otherMoney.dollar; m.cent = this.cent + otherMoney.cent; if(m.cent >=100) { m.dollar++; m.cent -= 100; } return m; } publicMoneysubtract(Money otherMoney) { Money m =newMoney(); m.dollar = this.dollar - otherMoney.dollar; m.cent = this.cent - otherMoney.cent; if(m.dollar <0|| m.cent <0) { returnnull; } return m; } publicbooleanequals(Money otherMoney) { returnthis== otherMoney; } publicintcompareTo(Money otherMoney) { Money m =newMoney(); if(this.dollar < otherMoney.dollar) { m = otherMoney.subtract(this); return-(m.dollar *100+ m.cent); } elseif(this.dollar > otherMoney.dollar) { m = this.subtract(otherMoney); return m.dollar *100+ m.cent; } else { return0; } } publicStringtoString() { if(this.cent <10) { return"$"+this.dollar +".0"+this.cent; } return"$"+this.dollar +"."+this.cent; } publicstaticMoneymax(Money[] m) { int max =(m[0].dollar*100)+ m[0].cent;//store the max as cents and then compare everything in cents int index =0; for(int i =0; i < m.length; i++) { if(max <(m[i].dollar*100)+ m[i].cent) { max = (m[i].dollar*100) + m[i].cent; index = i; } } return m[index]; } publicstaticvoidselectionSort(Money arr[]) { int n = arr.length; for(int i =0; i < n -1; i++) { int min = i; for(int j = i +1; j < n; j++) if(arr[j].dollar +((arr[j].cent)/100.0)< arr[min].dollar +((arr[min].cent)/100.0)) min = j; // Swap the found minimum element with the first element Money temp =newMoney(); temp = arr[min]; arr[min] = arr[i]; arr[i] = temp; } } public static void printList(Money[] m, int i) // This method takes an array of object as parameter { int counter=1; for(Money mm : m) { System.out.print(mm +" "); if(counter==i) System.out.print("\n"); counter++; } System.out.println(); } }
How can i remove the extra spacing from my output while keeping the new line. This is my code: public class Money { private int dollar; private int cent; final static int MIN_CENT_VALUE = 0; final static int MAX_CENT_VALUE = 99; public Money() { this.dollar = 0; this.cent = 0; } public Money(int dollar, int cent) { this.dollar = dollar; if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) { this.cent = cent; } else { cent = 0; } } public int getDollar() { return this.dollar; } public void setDollar(int dol) { this.dollar = dol; } public int getCent() { return this.cent; } public void setCent(int c) { if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) { this.cent = c; } } publicMoneyadd(Money otherMoney) { Money m =newMoney(); m.dollar = this.dollar + otherMoney.dollar; m.cent = this.cent + otherMoney.cent; if(m.cent >=100) { m.dollar++; m.cent -= 100; } return m; } publicMoneysubtract(Money otherMoney) { Money m =newMoney(); m.dollar = this.dollar - otherMoney.dollar; m.cent = this.cent - otherMoney.cent; if(m.dollar <0|| m.cent <0) { returnnull; } return m; } publicbooleanequals(Money otherMoney) { returnthis== otherMoney; } publicintcompareTo(Money otherMoney) { Money m =newMoney(); if(this.dollar < otherMoney.dollar) { m = otherMoney.subtract(this); return-(m.dollar *100+ m.cent); } elseif(this.dollar > otherMoney.dollar) { m = this.subtract(otherMoney); return m.dollar *100+ m.cent; } else { return0; } } publicStringtoString() { if(this.cent <10) { return"$"+this.dollar +".0"+this.cent; } return"$"+this.dollar +"."+this.cent; } publicstaticMoneymax(Money[] m) { int max =(m[0].dollar*100)+ m[0].cent;//store the max as cents and then compare everything in cents int index =0; for(int i =0; i < m.length; i++) { if(max <(m[i].dollar*100)+ m[i].cent) { max = (m[i].dollar*100) + m[i].cent; index = i; } } return m[index]; } publicstaticvoidselectionSort(Money arr[]) { int n = arr.length; for(int i =0; i < n -1; i++) { int min = i; for(int j = i +1; j < n; j++) if(arr[j].dollar +((arr[j].cent)/100.0)< arr[min].dollar +((arr[min].cent)/100.0)) min = j; // Swap the found minimum element with the first element Money temp =newMoney(); temp = arr[min]; arr[min] = arr[i]; arr[i] = temp; } } public static void printList(Money[] m, int i) // This method takes an array of object as parameter { int counter=1; for(Money mm : m) { System.out.print(mm +" "); if(counter==i) System.out.print("\n"); counter++; } System.out.println(); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How can i remove the extra spacing from my output while keeping the new line. This is my code:
public class Money {
private int dollar;
private int cent;
final static int MIN_CENT_VALUE = 0;
final static int MAX_CENT_VALUE = 99;
public Money() {
this.dollar = 0;
this.cent = 0;
}
public Money(int dollar, int cent) {
this.dollar = dollar;
if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) {
this.cent = cent;
} else {
cent = 0;
}
}
public int getDollar() {
return this.dollar;
}
public void setDollar(int dol) {
this.dollar = dol;
}
public int getCent() {
return this.cent;
}
public void setCent(int c) {
if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) {
this.cent = c;
}
}
publicMoneyadd(Money otherMoney)
{
Money m =newMoney();
m.dollar = this.dollar + otherMoney.dollar;
m.cent = this.cent + otherMoney.cent;
if(m.cent >=100)
{
m.dollar++;
m.cent -= 100;
}
return m;
}
publicMoneysubtract(Money otherMoney)
{
Money m =newMoney();
m.dollar = this.dollar - otherMoney.dollar;
m.cent = this.cent - otherMoney.cent;
if(m.dollar <0|| m.cent <0)
{
returnnull;
}
return m;
}
publicbooleanequals(Money otherMoney)
{
returnthis== otherMoney;
}
publicintcompareTo(Money otherMoney)
{
Money m =newMoney();
if(this.dollar < otherMoney.dollar)
{
m = otherMoney.subtract(this);
return-(m.dollar *100+ m.cent);
}
elseif(this.dollar > otherMoney.dollar)
{
m = this.subtract(otherMoney);
return m.dollar *100+ m.cent;
}
else
{
return0;
}
}
publicStringtoString()
{
if(this.cent <10)
{
return"$"+this.dollar +".0"+this.cent;
}
return"$"+this.dollar +"."+this.cent;
}
publicstaticMoneymax(Money[] m)
{
int max =(m[0].dollar*100)+ m[0].cent;//store the max as cents and then compare everything in cents
int index =0;
for(int i =0; i < m.length; i++)
{
if(max <(m[i].dollar*100)+ m[i].cent)
{
max = (m[i].dollar*100) + m[i].cent;
index = i;
}
}
return m[index];
}
publicstaticvoidselectionSort(Money arr[])
{
int n = arr.length;
for(int i =0; i < n -1; i++)
{
int min = i;
for(int j = i +1; j < n; j++)
if(arr[j].dollar +((arr[j].cent)/100.0)< arr[min].dollar +((arr[min].cent)/100.0))
min = j;
// Swap the found minimum element with the first element
Money temp =newMoney();
temp = arr[min];
arr[min] = arr[i];
arr[i] = temp;
}
}
public static void printList(Money[] m, int i) // This method takes an array of object as parameter
{
int counter=1;
for(Money mm : m)
{
System.out.print(mm +" ");
if(counter==i)
System.out.print("\n");
counter++;
}
System.out.println();
}
}
![C I have X
←
Monne x C My cocx |
learn.zybooks.com/zybook/CSUNCOMP110ZartoshtyFall2022/chapter/11/section/17
COMP X
Monney Lab.pdf
=zyBooks My library > COMP 110: Introduction to Algorithms and Programming home > 11.17: Money
Your output
Answe X zy Section X b Answe x
Expected output
5 previous submissions
[+] Extra Credit Digi....pdf
+ $10.95 is $16.40
$10.95 is null
$5.45 is $5.50
5
s method result: false
b Succes x
45+$10.95 is $16.40
45 $10.95 is null
.95 $5.45 is $5.50
als method result: false
pareTo method result: -550
orted list
zy zyBool X
C Post a X
reTo method result: -550
ted list
$4.90 $17.56 $8.88 $22.14 $14.99 $22.08 $16.80 $17.53 $14.38
$16.08 $6.49 $15.70 $9.50
Ow The gr X
9
ax is $24.09
d list
$4.90 $6.49 $8.88 $9.50 $14.38 $14.99 $15.70 $16.08 $16.80
3 $17.56 $22.08 $22.14 $24.09
comp hw .jpeg
EzyBooks catalog ? Help/FAQ
30 $4.90 $17.56 $8.88 $22.14 $14.99 $22.08 $16.80 $17.53 $14.38
.09 $16.08 $6.49 $15.70 $9.50
Max is $24.09
ted list
30 $4.90 $6.49 $8.88 $9.50 $14.38 $14.99 $15.70 $16.08 $16.80
53 $17.56 $22.08 $22.14 $24.09
digital X
Digital X
R
Rayan Jathan
Show All
X](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4b13bf20-6c75-4968-9db9-7aa89f4710e6%2F895352be-8216-4961-b1bb-391b8181de0b%2Fvd6mnme_processed.png&w=3840&q=75)
Transcribed Image Text:C I have X
←
Monne x C My cocx |
learn.zybooks.com/zybook/CSUNCOMP110ZartoshtyFall2022/chapter/11/section/17
COMP X
Monney Lab.pdf
=zyBooks My library > COMP 110: Introduction to Algorithms and Programming home > 11.17: Money
Your output
Answe X zy Section X b Answe x
Expected output
5 previous submissions
[+] Extra Credit Digi....pdf
+ $10.95 is $16.40
$10.95 is null
$5.45 is $5.50
5
s method result: false
b Succes x
45+$10.95 is $16.40
45 $10.95 is null
.95 $5.45 is $5.50
als method result: false
pareTo method result: -550
orted list
zy zyBool X
C Post a X
reTo method result: -550
ted list
$4.90 $17.56 $8.88 $22.14 $14.99 $22.08 $16.80 $17.53 $14.38
$16.08 $6.49 $15.70 $9.50
Ow The gr X
9
ax is $24.09
d list
$4.90 $6.49 $8.88 $9.50 $14.38 $14.99 $15.70 $16.08 $16.80
3 $17.56 $22.08 $22.14 $24.09
comp hw .jpeg
EzyBooks catalog ? Help/FAQ
30 $4.90 $17.56 $8.88 $22.14 $14.99 $22.08 $16.80 $17.53 $14.38
.09 $16.08 $6.49 $15.70 $9.50
Max is $24.09
ted list
30 $4.90 $6.49 $8.88 $9.50 $14.38 $14.99 $15.70 $16.08 $16.80
53 $17.56 $22.08 $22.14 $24.09
digital X
Digital X
R
Rayan Jathan
Show All
X
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
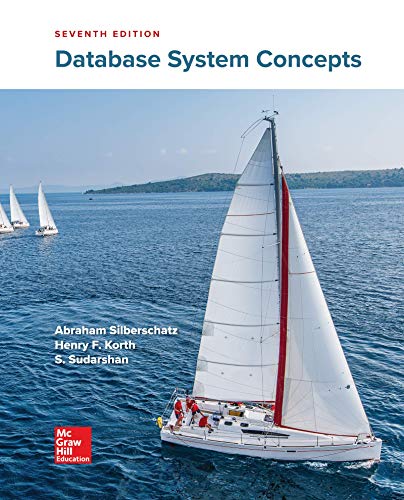
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
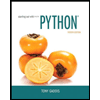
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
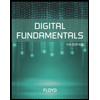
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
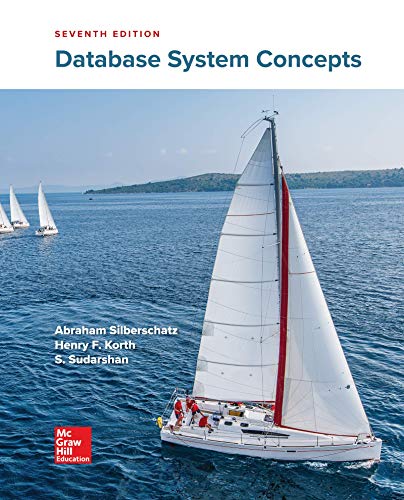
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
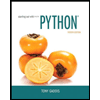
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
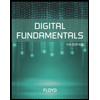
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
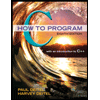
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
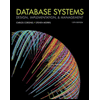
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
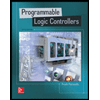
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education