I am having trouble determining time complexity when it comes to finding the runtime of a method or function. I need to know what the runtime for the following methods are. Any further explanation would also be helpful. All of these methods are dealing with a binary search tree in java. 1. public String getAllValues() - return a String that contains the elements of the tree in ascending order. All elements are separated by a space. 2. public int numberNodes() - return the total number of nodes in the tree. 3. public void insertList(int[] list) - insert all of the values from list into the tree. Note: My Method just goes through an array calling working insert() method on all the values in the list[] array. 4. public int removeLessThan(int value) - this method removes all integers strictly less than value from the tree. It also returns the number of nodes it removed. Note: In this method I first call a method to count nodes less than I call another method to recursively remove by truncating the left tree of any node less than value 5. public int numberOfLeaves() - return the number of leaves in the tree. 6. public int numberOfFullParents() - return the number of nodes that have 2 children. 7. public int howManyAtLevel(int level) - this method returns the num- ber of nodes at a particular level. 8. public int removeAllLeaves() - this method removes all leaves from the original tree. It also returns the number of leaves it removed. I only need the runtimes in terms of Big Theta. I DO NOT NEED THE METHODS as this is already done. I am assuming most of the runtimes are Big Theta of (n) but not completely sure. Thank you.
I am having trouble determining time complexity when it comes to finding the runtime of a method or function. I need to know what the runtime for the following methods are. Any further explanation would also be helpful.
All of these methods are dealing with a binary search tree in java.
1. public String getAllValues() - return a String that contains the elements
of the tree in ascending order. All elements are separated by a space.
2. public int numberNodes() - return the total number of nodes in the tree.
3. public void insertList(int[] list) - insert all of the values from list into
the tree.
Note: My Method just goes through an array calling working insert() method on all the values in the list[] array.
4. public int removeLessThan(int value) - this method removes all integers strictly less than value from the tree. It also returns the number of nodes it
removed.
Note: In this method I first call a method to count nodes less than I call another method to recursively remove by truncating the left tree of any node less than value
5. public int numberOfLeaves() - return the
number of leaves in the tree.
6. public int numberOfFullParents() - return the number of nodes that
have 2 children.
7. public int howManyAtLevel(int level) - this method returns the num-
ber of nodes at a particular level.
8. public int removeAllLeaves() - this method removes all leaves from the
original tree. It also returns the number of leaves it removed.
I only need the runtimes in terms of Big Theta. I DO NOT NEED THE METHODS as this is already done.
I am assuming most of the runtimes are Big Theta of (n) but not completely sure. Thank you.

Step by step
Solved in 3 steps

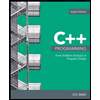
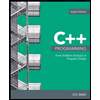