I got part of this code right but the other part I dont this is the question - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Now, write a program that declares an array of five Purchase objects and prompt a user for their values. As each Purchase object is created, continuously prompt until the user enters an invoice number between 1000 and 8000 inclusive and a non-negative sale amount. Prompt the user for values for each object and then display all the values. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return saleAmount; } public int getInvoiceNumber() { return invoiceNumber; } public void display() { System.out.println("Invoice #" + invoiceNumber + " Amount of sale: $" + saleAmount + " Tax: $" + tax); } } import java.util.*; public class PurchaseArray { public static void main(String[] args) { // Write your code here //array of five Purchase objects Purchase[] purchases = new Purchase[5]; int num; double amount; Scanner input = new Scanner(System.in); int x; final int LOW = 1000, HIGH = 8000; //loop over Purchase length for(x = 0; x < purchases.length; ++x) { purchases[x] = new Purchase(); System.out.println("------Purchase " + (x+1) + "-------"); System.out.print("Enter invoice number >> "); num = input.nextInt(); while(num < LOW || num > HIGH) { System.out.println("Invoice number must be between " + LOW + " and " + HIGH); System.out.print("Enter invoice number >> "); num = input.nextInt(); } System.out.print("Enter sale amount >> "); amount = input.nextDouble(); //call methods for each purchase to set invoice number and sales amount purchases[x].setInvoiceNumber(num); purchases[x].setSaleAmount(amount); } System.out.println(); //print result for(x = 0; x < purchases.length; ++x) { purchases[x].display(); } } } This is the check information that I am missing - Test CaseIncomplete PurchaseArrayTest 2 Input 5689 12.5 4578 25.89 1436 25.89 21 2165 -57 57.78 8541 6396 4578.20 Output------Purchase 1------- 5689 Enter invoice number >> Enter sale amount >> 12.5 ------Purchase 2------- Enter invoice number >> 4578 Enter sale amount >> 25.89 ------Purchase 3------- Enter invoice number >> 1436 Enter sale amount >> 25.89 ------ Purchase 4------- Enter invoice number >> 21 Invoice number must be between 1000 and 8000 Enter invoice number >> 2165 Enter sale amount >> -57 ------Purchase 5------- Enter invoice number >> 57.78 Exception in thread "main" java.util.InputMismatchException at java.util.Scanner.throwFor(Scanner.java:864) at java.util.Scanner.next(Scanner.java:1485) at java.util.Scanner.nextInt(Scanner.java:2117) at java.util.Scanner.nextInt(Scanner.java:2076) at PurchaseArray.main(PurchaseArray.java:18) 8541 6396 4578.20 Results(all in red) #5689 $12.5 $0.625 #4578 $25.89 $1.29 #1436 $25.89 $1.29 #2165 $57.78 $2.88 #6396 $4578.2 $228.91
I got part of this code right but the other part I dont this is the question - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Now, write a program that declares an array of five Purchase objects and prompt a user for their values. As each Purchase object is created, continuously prompt until the user enters an invoice number between 1000 and 8000 inclusive and a non-negative sale amount. Prompt the user for values for each object and then display all the values. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return saleAmount; } public int getInvoiceNumber() { return invoiceNumber; } public void display() { System.out.println("Invoice #" + invoiceNumber + " Amount of sale: $" + saleAmount + " Tax: $" + tax); } } import java.util.*; public class PurchaseArray { public static void main(String[] args) { // Write your code here //array of five Purchase objects Purchase[] purchases = new Purchase[5]; int num; double amount; Scanner input = new Scanner(System.in); int x; final int LOW = 1000, HIGH = 8000; //loop over Purchase length for(x = 0; x < purchases.length; ++x) { purchases[x] = new Purchase(); System.out.println("------Purchase " + (x+1) + "-------"); System.out.print("Enter invoice number >> "); num = input.nextInt(); while(num < LOW || num > HIGH) { System.out.println("Invoice number must be between " + LOW + " and " + HIGH); System.out.print("Enter invoice number >> "); num = input.nextInt(); } System.out.print("Enter sale amount >> "); amount = input.nextDouble(); //call methods for each purchase to set invoice number and sales amount purchases[x].setInvoiceNumber(num); purchases[x].setSaleAmount(amount); } System.out.println(); //print result for(x = 0; x < purchases.length; ++x) { purchases[x].display(); } } } This is the check information that I am missing - Test CaseIncomplete PurchaseArrayTest 2 Input 5689 12.5 4578 25.89 1436 25.89 21 2165 -57 57.78 8541 6396 4578.20 Output------Purchase 1------- 5689 Enter invoice number >> Enter sale amount >> 12.5 ------Purchase 2------- Enter invoice number >> 4578 Enter sale amount >> 25.89 ------Purchase 3------- Enter invoice number >> 1436 Enter sale amount >> 25.89 ------ Purchase 4------- Enter invoice number >> 21 Invoice number must be between 1000 and 8000 Enter invoice number >> 2165 Enter sale amount >> -57 ------Purchase 5------- Enter invoice number >> 57.78 Exception in thread "main" java.util.InputMismatchException at java.util.Scanner.throwFor(Scanner.java:864) at java.util.Scanner.next(Scanner.java:1485) at java.util.Scanner.nextInt(Scanner.java:2117) at java.util.Scanner.nextInt(Scanner.java:2076) at PurchaseArray.main(PurchaseArray.java:18) 8541 6396 4578.20 Results(all in red) #5689 $12.5 $0.625 #4578 $25.89 $1.29 #1436 $25.89 $1.29 #2165 $57.78 $2.88 #6396 $4578.2 $228.91
Chapter8: Arrays
Section: Chapter Questions
Problem 11PE
Related questions
Question
I got part of this code right but the other part I dont this is the question -
In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods.
Now, write a program that declares an array of five Purchase objects and prompt a user for their values. As each Purchase object is created, continuously prompt until the user enters an invoice number between 1000 and 8000 inclusive and a non-negative sale amount. Prompt the user for values for each object and then display all the values.
This is the code I have -
public class Purchase
{
private int invoiceNumber;
private double saleAmount;
private double tax;
private static final double RATE = 0.05;
public void setInvoiceNumber(int num)
{
invoiceNumber = num;
}
public void setSaleAmount(double amt)
{
saleAmount = amt;
tax = saleAmount * RATE;
}
public double getSaleAmount()
{
return saleAmount;
}
public int getInvoiceNumber()
{
return invoiceNumber;
}
public void display()
{
System.out.println("Invoice #" + invoiceNumber +
" Amount of sale: $" + saleAmount + " Tax: $" + tax);
}
}
import java.util.*;
public class PurchaseArray {
public static void main(String[] args) {
// Write your code here
//array of five Purchase objects
Purchase[] purchases = new Purchase[5];
int num;
double amount;
Scanner input = new Scanner(System.in);
int x;
final int LOW = 1000, HIGH = 8000;
//loop over Purchase length
for(x = 0; x < purchases.length; ++x)
{
purchases[x] = new Purchase();
System.out.println("------Purchase " + (x+1) + "-------");
System.out.print("Enter invoice number >> ");
num = input.nextInt();
while(num < LOW || num > HIGH)
{
System.out.println("Invoice number must be between " + LOW + " and " + HIGH);
System.out.print("Enter invoice number >> ");
num = input.nextInt();
}
System.out.print("Enter sale amount >> ");
amount = input.nextDouble();
//call methods for each purchase to set invoice number and sales amount
purchases[x].setInvoiceNumber(num);
purchases[x].setSaleAmount(amount);
}
System.out.println();
//print result
for(x = 0; x < purchases.length; ++x)
{
purchases[x].display();
}
}
}
This is the check information that I am missing -
Test CaseIncomplete
PurchaseArrayTest 2
Input
5689 12.5 4578 25.89 1436 25.89 21 2165 -57 57.78 8541 6396 4578.20
Output------Purchase 1------- 5689 Enter invoice number >> Enter sale amount >> 12.5
------Purchase 2------- Enter invoice number >> 4578 Enter sale amount >> 25.89
------Purchase 3------- Enter invoice number >> 1436 Enter sale amount >> 25.89 ------
Purchase 4------- Enter invoice number >> 21 Invoice number must be between 1000 and 8000 Enter invoice number >> 2165 Enter sale amount >> -57
------Purchase 5------- Enter invoice number >> 57.78 Exception in thread "main" java.util.InputMismatchException at java.util.Scanner.throwFor(Scanner.java:864) at java.util.Scanner.next(Scanner.java:1485) at java.util.Scanner.nextInt(Scanner.java:2117) at java.util.Scanner.nextInt(Scanner.java:2076) at PurchaseArray.main(PurchaseArray.java:18)
8541
6396
4578.20
Results(all in red)
#5689
$12.5
$0.625
#4578
$25.89
$1.29
#1436
$25.89
$1.29
#2165
$57.78
$2.88
#6396
$4578.2
$228.91
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
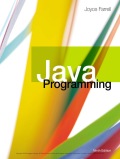
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
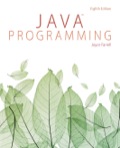
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
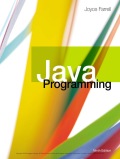
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
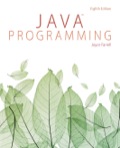
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
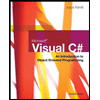
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,