#ifndef H_StackType #define H_StackType #include #include using namespace std; template struct nodeType { Type info; nodeType *link; }; template class linkedStackType: public stackADT class linkedStackType { public: const linkedStackType& operator= (const linkedStackType&); bool isEmptyStack() const; bool isFullStack() const; void initializeStack(); //Function to initialize the stack to an empty state. void push(const Type& newItem); Type top() const; //Function to return the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: If the stack is empty, the program // terminates; otherwise, the top element of // the stack is returned. void pop(); linkedStackType(); //Postcondition: stackTop = NULL; linkedStackType(const linkedStackType& otherStack); r ~linkedStackType(); nodeType *stackTop; //pointer to the stack void copyStack(const linkedStackType& otherStack); //Function to make a copy of otherStack. //Postcondition: A copy of otherStack is created and // assigned to this stack. }; template linkedStackType::linkedStackType() { stackTop = NULL; } template bool linkedStackType::isEmptyStack() const { return(stackTop == NULL); } //end isEmptyStack template bool linkedStackType:: isFullStack() const { return false; } //end isFullStack template void linkedStackType:: initializeStack() { nodeType *temp; //pointer to delete the node while (stackTop != NULL) { temp = stackTop; //set temp to point to the //current node stackTop = stackTop->link; //advance stackTop to the //next node delete temp; //deallocate memory occupied by temp } } //end initializeStack template void linkedStackType::push(const Type& newElement) { nodeType *newNode; //pointer to create the new node newNode = new nodeType; //create the node newNode->info = newElement; //store newElement in the node newNode->link = stackTop; //insert newNode before stackTop stackTop = newNode; //set stackTop to point to the //top node } //end push template Type linkedStackType::top() const { assert(stackTop != NULL); //if stack is empty, //terminate the program return stackTop->info; //return the top element }/ template void linkedStackType::pop() { nodeType *temp; //pointer to deallocate memory if (stackTop != NULL) { temp = stackTop; //set temp to point to the top node stackTop = stackTop->link; //advance stackTop to the //next node delete temp; //delete the top node } else cout << "Cannot remove from an empty stack." << endl; } template void linkedStackType::copyStack (const linkedStackType& otherStack) { nodeType *newNode, *current, *last; if (stackTop != NULL) //if stack is nonempty, make it empty initializeStack(); if (otherStack.stackTop == NULL) stackTop = NULL; else { current = otherStack.stackTop; //set current to point //to the stack to be copied //copy the stackTop element of the stack stackTop = new nodeType; //create the node stackTop->info = current->info; //copy the info stackTop->link = NULL; //set the link field of the //node to NULL last = stackTop; //set last to point to the node current = current->link; //set current to point to //the next node //copy the remaining stack while (current != NULL) { newNode = new nodeType; newNode->info = current->info; newNode->link = NULL; last->link = newNode; last = newNode; current = current->link; } } } template linkedStackType::linkedStackType( const linkedStackType& otherStack) { stackTop = NULL; copyStack(otherStack); } template linkedStackType::~linkedStackType() { initializeStack(); }//end destructor template const linkedStackType& linkedStackType::operator=(const linkedStackType& otherStack) { if (this != &otherStack) //avoid self-copy copyStack(otherStack); return *this; }//end operator= #endif
#ifndef H_StackType
#define H_StackType
#include <iostream>
#include <cassert>
using namespace std;
template <class Type>
struct nodeType
{
Type info;
nodeType<Type> *link;
};
template <class Type>
class linkedStackType: public stackADT<Type>
class linkedStackType
{
public:
const linkedStackType<Type>& operator=
(const linkedStackType<Type>&);
bool isEmptyStack() const;
bool isFullStack() const;
void initializeStack();
//Function to initialize the stack to an empty state.
void push(const Type& newItem);
Type top() const;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element of
// the stack is returned.
void pop();
linkedStackType();
//Postcondition: stackTop = NULL;
linkedStackType(const linkedStackType<Type>& otherStack);
r
~linkedStackType();
nodeType<Type> *stackTop; //pointer to the stack
void copyStack(const linkedStackType<Type>& otherStack);
//Function to make a copy of otherStack.
//Postcondition: A copy of otherStack is created and
// assigned to this stack.
};
template <class Type>
linkedStackType<Type>::linkedStackType()
{
stackTop = NULL;
}
template <class Type>
bool linkedStackType<Type>::isEmptyStack() const
{
return(stackTop == NULL);
} //end isEmptyStack
template <class Type>
bool linkedStackType<Type>:: isFullStack() const
{
return false;
} //end isFullStack
template <class Type>
void linkedStackType<Type>:: initializeStack()
{
nodeType<Type> *temp; //pointer to delete the node
while (stackTop != NULL)
{
temp = stackTop; //set temp to point to the
//current node
stackTop = stackTop->link; //advance stackTop to the
//next node
delete temp; //deallocate memory occupied by temp
}
} //end initializeStack
template <class Type>
void linkedStackType<Type>::push(const Type& newElement)
{
nodeType<Type> *newNode; //pointer to create the new node
newNode = new nodeType<Type>; //create the node
newNode->info = newElement; //store newElement in the node
newNode->link = stackTop; //insert newNode before stackTop
stackTop = newNode; //set stackTop to point to the
//top node
} //end push
template <class Type>
Type linkedStackType<Type>::top() const
{
assert(stackTop != NULL); //if stack is empty,
//terminate the program
return stackTop->info; //return the top element
}/
template <class Type>
void linkedStackType<Type>::pop()
{
nodeType<Type> *temp; //pointer to deallocate memory
if (stackTop != NULL)
{
temp = stackTop; //set temp to point to the top node
stackTop = stackTop->link; //advance stackTop to the
//next node
delete temp; //delete the top node
}
else
cout << "Cannot remove from an empty stack." << endl;
}
template <class Type>
void linkedStackType<Type>::copyStack
(const linkedStackType<Type>& otherStack)
{
nodeType<Type> *newNode, *current, *last;
if (stackTop != NULL) //if stack is nonempty, make it empty
initializeStack();
if (otherStack.stackTop == NULL)
stackTop = NULL;
else
{
current = otherStack.stackTop; //set current to point
//to the stack to be copied
//copy the stackTop element of the stack
stackTop = new nodeType<Type>; //create the node
stackTop->info = current->info; //copy the info
stackTop->link = NULL; //set the link field of the
//node to NULL
last = stackTop; //set last to point to the node
current = current->link; //set current to point to
//the next node
//copy the remaining stack
while (current != NULL)
{
newNode = new nodeType<Type>;
newNode->info = current->info;
newNode->link = NULL;
last->link = newNode;
last = newNode;
current = current->link;
}
}
}
template <class Type>
linkedStackType<Type>::linkedStackType(
const linkedStackType<Type>& otherStack)
{
stackTop = NULL;
copyStack(otherStack);
}
template <class Type>
linkedStackType<Type>::~linkedStackType()
{
initializeStack();
}//end destructor
template <class Type>
const linkedStackType<Type>& linkedStackType<Type>::operator=(const linkedStackType<Type>& otherStack)
{
if (this != &otherStack) //avoid self-copy
copyStack(otherStack);
return *this;
}//end operator=
#endif


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

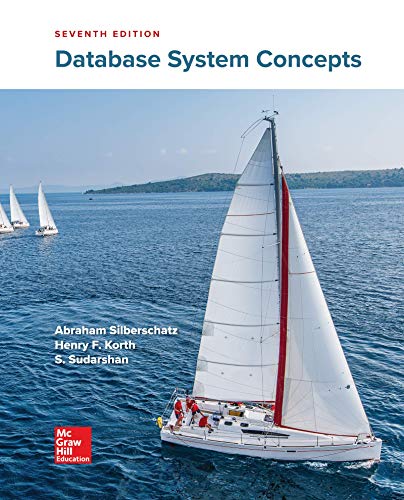
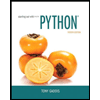
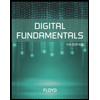
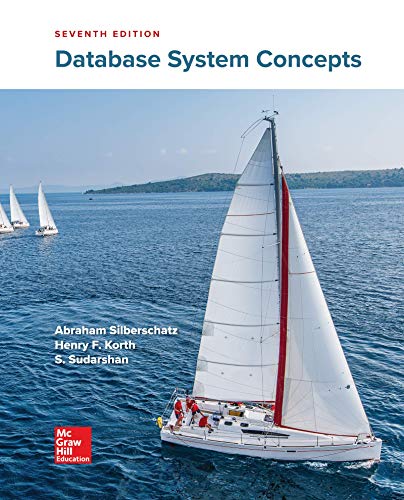
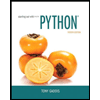
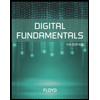
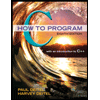
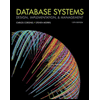
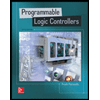