I'm interested in creating a digital personal assistant similar to Alexa and Siri, which can efficiently manage schedules. Specifically, I want to design a tool in Java that allows users to find events during a certain time period. This tool should also enable users to add and cancel events as their schedules change. To achieve this, I plan to use a SkipListMap class with specific operations (get, put, remove, subMap) and a DoublyLinkedList class. Additionally, I'll use the FakeRandomHeight class for debugging and testing. Could you provide guidance on how to approach this project, especially in terms of implementing the SkipListMap class and managing event data efficiently? Sample Input: AddEvent 101910 Breakfast AddEvent 101913 Lunch AddEvent 101918 Dinner GetEvent 101914 AddEvent 101911 Data Structures AddEvent 102007 Exercise PrintSkipList AddEvent 101915 Homework CancelEvent 102007 AddEvent 102013 Relax AddEvent 102011 Read a Book GetEvent 101915 AddEvent 101914 Call Mom PrintSkipList I would like the time to be in integer where it is in MMDDHH format and date is in MMDD format (MM is 01-12, DD is 01-31, and HH is 00-23). Sample Output: AddEvent 101910 Breakfast AddEvent 101913 Lunch AddEvent 101918 Dinner GetEvent 101914 none AddEvent 101911 Data Structures AddEvent 102007 Exercise PrintSkipList (S3) empty (S2) 101911:Data Structures (S1) 101911:Data Structures 101913:Lunch (S0) 101910:Breakfast 101911:Data Structures 101913:Lunch 101918:Dinner 102007:Exercise AddEvent 101915 Homework CancelEvent 102007 Exercise AddEvent 102013 Relax AddEvent 102011 Read a Book GetEvent 101915 Homework AddEvent 101914 Call Mom PrintSkipList (S4) empty (S3) 102011:Read a Book (S2) 101911:Data Structures 102011:Read a Book (S1) 101911:Data Structures 101915:Homework 101918:Lunch 102011:Read a Book (S0) 101910:Breakfast 101911:Data Structures 101913:Lunch 101914:Call Mom 101915:Homework 101918:Dinner 102011:Read a Book 102013:Relax
I'm interested in creating a digital personal assistant similar to Alexa and Siri, which can efficiently manage schedules. Specifically, I want to design a tool in Java that allows users to find events during a certain time period. This tool should also enable users to add and cancel events as their schedules change. To achieve this, I plan to use a SkipListMap class with specific operations (get, put, remove, subMap) and a DoublyLinkedList class. Additionally, I'll use the FakeRandomHeight class for debugging and testing.
Could you provide guidance on how to approach this project, especially in terms of implementing the SkipListMap class and managing event data efficiently?
Sample Input:
AddEvent 101913 Lunch
AddEvent 101918 Dinner
GetEvent 101914
AddEvent 101911 Data Structures
AddEvent 102007 Exercise
PrintSkipList
AddEvent 101915 Homework
CancelEvent 102007
AddEvent 102013 Relax
AddEvent 102011 Read a Book
GetEvent 101915
AddEvent 101914 Call Mom
PrintSkipList
00-23).
Sample Output:
AddEvent 101913 Lunch
AddEvent 101918 Dinner
GetEvent 101914 none
AddEvent 101911 Data Structures
AddEvent 102007 Exercise
PrintSkipList
(S3) empty
(S2) 101911:Data Structures
(S1) 101911:Data Structures 101913:Lunch
(S0) 101910:Breakfast 101911:Data Structures 101913:Lunch 101918:Dinner
102007:Exercise
AddEvent 101915 Homework
CancelEvent 102007 Exercise
AddEvent 102013 Relax
AddEvent 102011 Read a Book
GetEvent 101915 Homework
AddEvent 101914 Call Mom
PrintSkipList
(S4) empty
(S3) 102011:Read a Book
(S2) 101911:Data Structures 102011:Read a Book
(S1) 101911:Data Structures 101915:Homework 101918:Lunch 102011:Read a
Book
(S0) 101910:Breakfast 101911:Data Structures 101913:Lunch 101914:Call Mom
101915:Homework 101918:Dinner 102011:Read a Book 102013:Relax

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

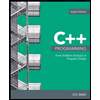
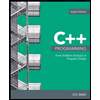