Implement codes such that it will pass 3 tests: 1. WalkingBoardBasicTestpackage walking.game.tests;import org.junit.platform.suite.api.SelectClasses;import org.junit.platform.suite.api.Suite; import walking.game.WalkingBoardTest; @SelectClasses({WalkingBoardBasicTestSuite.StructuralTests.class, WalkingBoardBasicTestSuite.FunctionalTests.class})@Suite public class WalkingBoardBasicTestSuite {@SelectClasses({DirectionStructureTest.class, WalkingBoardStructureTest.class})@Suite public static class StructuralTests {} @SelectClasses({WalkingBoardTest.class})@Suite public static class FunctionalTests {}}2. WalkingBoardStructure:package walking.game.tests; import static check.CheckThat.*;import static check.CheckThat.Condition.*; import org.junit.jupiter.api.BeforeAll;import org.junit.jupiter.api.Test;import org.junit.jupiter.api.condition.DisabledIf; import check.CheckThat; public class WalkingBoardStructureTest {@BeforeAllpublicstaticvoidinit() {CheckThat.theClass("walking.game.WalkingBoard").thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL);} @Test@DisabledIf(notApplicable)publicvoidfieldTiles() {it.hasField("tiles: array of array of int").thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE).thatHas(GETTER).thatHasNo(SETTER);} @Test@DisabledIf(notApplicable)publicvoidfieldX() {it.hasField("x: int").thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE).thatHasNo(GETTER, SETTER);} @Test@DisabledIf(notApplicable)publicvoidfieldY() {it.hasField("y: int").thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE).thatHasNo(GETTER, SETTER);} @Test@DisabledIf(notApplicable)publicvoidfieldBASE_TILE_SCORE() {it.hasField("BASE_TILE_SCORE: int").thatIs(USABLE_WITHOUT_INSTANCE, NOT_MODIFIABLE, VISIBLE_TO_ALL).thatHasNo(GETTER, SETTER).withInitialValue(3);} @Test@DisabledIf(notApplicable)publicvoidconstructor01() {it.hasConstructor(withArgs("size: int")).thatIs(VISIBLE_TO_ALL);} @Test@DisabledIf(notApplicable)publicvoidconstructor02() {it.hasConstructor(withArgs("tiles: array of array of int")).thatIs(VISIBLE_TO_ALL);} @Test@DisabledIf(notApplicable)publicvoidmethodGetPosition() {it.hasMethod("getPosition", withNoParams()).thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL).thatReturns("array of int");} @Test@DisabledIf(notApplicable)publicvoidmethodIsValidPosition() {it.hasMethod("isValidPosition", withParams("x: int", "y: int")).thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL).thatReturns("boolean");} @Test@DisabledIf(notApplicable)publicvoidmethodGetTile() {it.hasMethod("getTile", withParams("x: int", "y: int")).thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL).thatReturns("int");} @Test@DisabledIf(notApplicable)publicvoidmethodGetX() {it.hasMethod("getXStep", withParams("direction: walking.game.util.Direction")).thatIs(FULLY_IMPLEMENTED, USABLE_WITHOUT_INSTANCE, VISIBLE_TO_ALL).thatReturns("int");} @Test@DisabledIf(notApplicable)publicvoidmethodGetY() {it.hasMethod("getYStep", withParams("direction: walking.game.util.Direction")).thatIs(FULLY_IMPLEMENTED, USABLE_WITHOUT_INSTANCE, VISIBLE_TO_ALL).thatReturns("int");} @Test@DisabledIf(notApplicable)publicvoidmethodMoveAndSet() {it.hasMethod("moveAndSet", withParams("TODOname: walking.game.util.Direction", "TODOname: int")).thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL).thatReturns("int");}}
Implement codes such that it will pass 3 tests:
1. WalkingBoardBasicTest
package walking.game.tests;
import org.junit.platform.suite.api.SelectClasses;
import org.junit.platform.suite.api.Suite;
import walking.game.WalkingBoardTest;
@SelectClasses({
WalkingBoardBasicTestSuite.StructuralTests.class
, WalkingBoardBasicTestSuite.FunctionalTests.class
})
@Suite public class WalkingBoardBasicTestSuite {
@SelectClasses({
DirectionStructureTest.class
, WalkingBoardStructureTest.class
})
@Suite public static class StructuralTests {}
@SelectClasses({
WalkingBoardTest.class
})
@Suite public static class FunctionalTests {}
}
2. WalkingBoardStructure:
package walking.game.tests;
import static check.CheckThat.*;
import static check.CheckThat.Condition.*;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.condition.DisabledIf;
import check.CheckThat;
public class WalkingBoardStructureTest {
@BeforeAll
publicstaticvoidinit() {
CheckThat.theClass("walking.game.WalkingBoard")
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldTiles() {
it.hasField("tiles: array of array of int")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE)
.thatHas(GETTER)
.thatHasNo(SETTER);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldX() {
it.hasField("x: int")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE)
.thatHasNo(GETTER, SETTER);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldY() {
it.hasField("y: int")
.thatIs(INSTANCE_LEVEL, MODIFIABLE, VISIBLE_TO_NONE)
.thatHasNo(GETTER, SETTER);
}
@Test
@DisabledIf(notApplicable)
publicvoidfieldBASE_TILE_SCORE() {
it.hasField("BASE_TILE_SCORE: int")
.thatIs(USABLE_WITHOUT_INSTANCE, NOT_MODIFIABLE, VISIBLE_TO_ALL)
.thatHasNo(GETTER, SETTER)
.withInitialValue(3);
}
@Test
@DisabledIf(notApplicable)
publicvoidconstructor01() {
it.hasConstructor(withArgs("size: int"))
.thatIs(VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidconstructor02() {
it.hasConstructor(withArgs("tiles: array of array of int"))
.thatIs(VISIBLE_TO_ALL);
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodGetPosition() {
it.hasMethod("getPosition", withNoParams())
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturns("array of int");
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodIsValidPosition() {
it.hasMethod("isValidPosition", withParams("x: int", "y: int"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturns("boolean");
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodGetTile() {
it.hasMethod("getTile", withParams("x: int", "y: int"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturns("int");
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodGetX() {
it.hasMethod("getXStep", withParams("direction: walking.game.util.Direction"))
.thatIs(FULLY_IMPLEMENTED, USABLE_WITHOUT_INSTANCE, VISIBLE_TO_ALL)
.thatReturns("int");
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodGetY() {
it.hasMethod("getYStep", withParams("direction: walking.game.util.Direction"))
.thatIs(FULLY_IMPLEMENTED, USABLE_WITHOUT_INSTANCE, VISIBLE_TO_ALL)
.thatReturns("int");
}
@Test
@DisabledIf(notApplicable)
publicvoidmethodMoveAndSet() {
it.hasMethod("moveAndSet", withParams("TODOname: walking.game.util.Direction", "TODOname: int"))
.thatIs(FULLY_IMPLEMENTED, INSTANCE_LEVEL, VISIBLE_TO_ALL)
.thatReturns("int");
}
}

Step by step
Solved in 2 steps

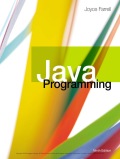
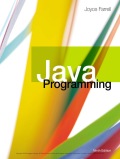