Implement the queue interface in ourArrayList
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java
HW: Implement the queue interface in ourArrayList. Modify ourArrayList to implement wQueue interface.
Ill attach imaged of "ourarraylist"
code:
package com.mac286.ourArrayList;
public interface wQueue <T>{
public boolean empty();
public T remove();
public void insert(T e);
public int size();
public String toString();
}
![package com.mac286.ourArrayList;
/*Design a linked list with the following properties:
* 1- A reference to the first node (Head) and a reference to the last node (Tail)
* 2- keep track of the size of the list int size
* 3- Constructor to create an empty list
* 4- getter for size
* 5- isEmpty() returns true it the list is empty and false othewise.
* 6- method void add (T e) that adds e to the back of the list. Like a queue
* 7- method void add (T e, int ind) that adds element e at the specified index.
* 8- method T remove() that removes the head and returns it (like a queue)
* 9- method T remove (int ind) removes the element at index ind and returns it.
* 10- method T get (int ind) returns the element at index ind without removing it.
* 11- tostring that displays the content of the list from the head to the tail between brackets []
public class ourArrayList<T>{
private Node<T> Head = null;
private Node<T> Tail = null;
private int size = 0;
//default constructor
public ourArrayList() {
Head = Tail = null;
size = 0;
}
public int size() {
return size;
}
public boolean isEmpty() {
return (size == 0);
}
//implement the method add, that adds to the back of the list
public void add(T e) {
//HW TODO and TEST
//create a node and assign e to the data of that node.
Node<T> N = new Node<T> ( );//N.mData is null and N.next is null as well
N.setsData (e);
//chain the new node to the list
//check if the list is empty, then deal with the special case
if(this.isEmpty()) {
//head and tail refer to N
this.Head = this.Tail = N;
size++;
//return we are done.
return;
}
//The list is not empty
//if the list is not empty. Then update the link of the last element to refer to the
//new node then update Tail to refer to the new node.
Tail.setNext(N);
Tail = N;
//increase size.
size++;
}
//remove removes the first/head and returns it.
public T remove() {
//check if empty then return null
if(this.isEmpty()) {
return null;
}
//it is not empty
//save the returned item
T save = this.Head. getData();
//Head should refer to the next node
this.Head = this.Head.getNext();
if(this.Head == null) {
this.Tail = null;
}
size--;
return save;
}
//T get (int ind)
public T get(int ind) {
returns the element at index ind without removing it.
//if list is empty return null
////if index is smaller than 0 or larger than size-1 return null
if(this.isEmpty() || ind < 0 || ind > size - 1) {
return null;
}
//create a temp reference, starting at Head.
Node<T> temp = this.Head;
//loop through the list ind times and return the data at the node.
for (int i = 0; i < ind; i++) {](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F33eba9e5-eb7d-44a1-93e3-ed0ee897e17f%2Fa7727365-4209-4888-a19f-dc89d555ad32%2Fk0g836_processed.png&w=3840&q=75)
Transcribed Image Text:package com.mac286.ourArrayList;
/*Design a linked list with the following properties:
* 1- A reference to the first node (Head) and a reference to the last node (Tail)
* 2- keep track of the size of the list int size
* 3- Constructor to create an empty list
* 4- getter for size
* 5- isEmpty() returns true it the list is empty and false othewise.
* 6- method void add (T e) that adds e to the back of the list. Like a queue
* 7- method void add (T e, int ind) that adds element e at the specified index.
* 8- method T remove() that removes the head and returns it (like a queue)
* 9- method T remove (int ind) removes the element at index ind and returns it.
* 10- method T get (int ind) returns the element at index ind without removing it.
* 11- tostring that displays the content of the list from the head to the tail between brackets []
public class ourArrayList<T>{
private Node<T> Head = null;
private Node<T> Tail = null;
private int size = 0;
//default constructor
public ourArrayList() {
Head = Tail = null;
size = 0;
}
public int size() {
return size;
}
public boolean isEmpty() {
return (size == 0);
}
//implement the method add, that adds to the back of the list
public void add(T e) {
//HW TODO and TEST
//create a node and assign e to the data of that node.
Node<T> N = new Node<T> ( );//N.mData is null and N.next is null as well
N.setsData (e);
//chain the new node to the list
//check if the list is empty, then deal with the special case
if(this.isEmpty()) {
//head and tail refer to N
this.Head = this.Tail = N;
size++;
//return we are done.
return;
}
//The list is not empty
//if the list is not empty. Then update the link of the last element to refer to the
//new node then update Tail to refer to the new node.
Tail.setNext(N);
Tail = N;
//increase size.
size++;
}
//remove removes the first/head and returns it.
public T remove() {
//check if empty then return null
if(this.isEmpty()) {
return null;
}
//it is not empty
//save the returned item
T save = this.Head. getData();
//Head should refer to the next node
this.Head = this.Head.getNext();
if(this.Head == null) {
this.Tail = null;
}
size--;
return save;
}
//T get (int ind)
public T get(int ind) {
returns the element at index ind without removing it.
//if list is empty return null
////if index is smaller than 0 or larger than size-1 return null
if(this.isEmpty() || ind < 0 || ind > size - 1) {
return null;
}
//create a temp reference, starting at Head.
Node<T> temp = this.Head;
//loop through the list ind times and return the data at the node.
for (int i = 0; i < ind; i++) {
![for (int i = 0; i < ind; i++) {
//move to the next
temp = temp.getNext();
}
//temp should refer to the node you are looking for. return the data in that node
return temp.getData();
}
public void add(int ind, T e) {
//if ind is not valid return
if(ind < 0 || ind > size) {
return;
}
//if ind == size just call add(e), add it to the back
if(ind == size) {
//insert at the end just call add (e)
this.add(e);
return;
}
//create a new node with e as data
Node<T> N = new Node<T> (e);
//if ind == 0; //add it ot the head as a special case and return
if(ind == 0) {
N.setNext(this.Head);
Нead %3D N;
size++;
return;
}
//The index is somewhere in between.
//Go to node ind - 1 and insert your Node.
Node<T> temp = this. Head;
for (int i =0; i<ind-1; i++) {
//go to next node
temp = temp.getNext();
}
//Set the next opf new node to next of temp
N.setNext(temp.getNext());
//set next of temp to new node
temp.setNext(N);
size++;
}
//TODO HW : removes the element at index ind and returns it. Test it.
public T remove (int ind) {
if(this.isEmpty() || ind < 0 || ind >= size)
return null;
if(ind == 0)
return this.remove(0;
//The index is in between, go to the node before the one to remove
//create temporary reference temp
Node<T> temp = this.Head;
for (int i = 0; i < ind-1; i++)
temp = temp.getNext();
//temp refers to the node before the one to remove.
//save what to return
T save = temp.getNext().getData();
//create the connection to the one after
temp.setNext(temp.getNext().getNext());
//Tail may need to be updated in case we are removing the last one.
if(temp.getNext() == null)
Tail = temp;
size--;
return save;
public String toString() {
if(this.isEmpty())
return "[]";
String st = "[";
//create a temp reference to the first node.
Node<T> temp = this.Head;
while(temp.getNext() != null) {
//go through all nodes until the last and add the content to the string.
st += temp.toString() + ", ";
//go to the next node
temp = temp.getNext();
}
//temp should poinrt to the last element at this point
st += temp.toString() + "]";
return st;
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F33eba9e5-eb7d-44a1-93e3-ed0ee897e17f%2Fa7727365-4209-4888-a19f-dc89d555ad32%2Ffage9br_processed.png&w=3840&q=75)
Transcribed Image Text:for (int i = 0; i < ind; i++) {
//move to the next
temp = temp.getNext();
}
//temp should refer to the node you are looking for. return the data in that node
return temp.getData();
}
public void add(int ind, T e) {
//if ind is not valid return
if(ind < 0 || ind > size) {
return;
}
//if ind == size just call add(e), add it to the back
if(ind == size) {
//insert at the end just call add (e)
this.add(e);
return;
}
//create a new node with e as data
Node<T> N = new Node<T> (e);
//if ind == 0; //add it ot the head as a special case and return
if(ind == 0) {
N.setNext(this.Head);
Нead %3D N;
size++;
return;
}
//The index is somewhere in between.
//Go to node ind - 1 and insert your Node.
Node<T> temp = this. Head;
for (int i =0; i<ind-1; i++) {
//go to next node
temp = temp.getNext();
}
//Set the next opf new node to next of temp
N.setNext(temp.getNext());
//set next of temp to new node
temp.setNext(N);
size++;
}
//TODO HW : removes the element at index ind and returns it. Test it.
public T remove (int ind) {
if(this.isEmpty() || ind < 0 || ind >= size)
return null;
if(ind == 0)
return this.remove(0;
//The index is in between, go to the node before the one to remove
//create temporary reference temp
Node<T> temp = this.Head;
for (int i = 0; i < ind-1; i++)
temp = temp.getNext();
//temp refers to the node before the one to remove.
//save what to return
T save = temp.getNext().getData();
//create the connection to the one after
temp.setNext(temp.getNext().getNext());
//Tail may need to be updated in case we are removing the last one.
if(temp.getNext() == null)
Tail = temp;
size--;
return save;
public String toString() {
if(this.isEmpty())
return "[]";
String st = "[";
//create a temp reference to the first node.
Node<T> temp = this.Head;
while(temp.getNext() != null) {
//go through all nodes until the last and add the content to the string.
st += temp.toString() + ", ";
//go to the next node
temp = temp.getNext();
}
//temp should poinrt to the last element at this point
st += temp.toString() + "]";
return st;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
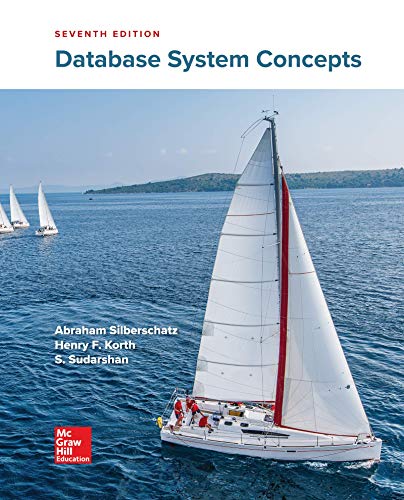
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
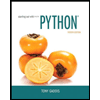
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
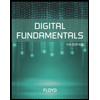
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
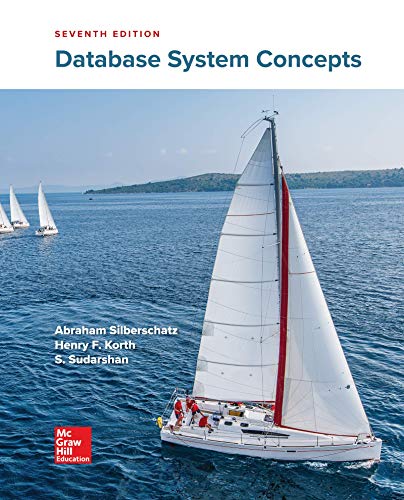
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
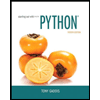
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
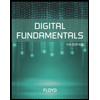
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
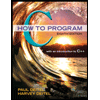
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
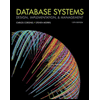
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
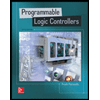
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education