import java.util.*; public class Watersort { Character top = null; // create constants for colors static Character red= 'r'; static Character blue = 'b'; static Character yellow= 'g'; // Bottles declaratio public static void showAll( StackAsMyArrayList bottles[]) { for (int i = 0; i<=4; i++) { System.out.println("Bottle "+ i+ ": " + bottles[i]); } } public static void main(String args[]) { //create the bottle StackAsMyArrayList bottleONE = new StackAsMyArrayList(); System.out.println("\nbottleONE:"+ bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); bottleONE.push(red); bottleONE.push(red); bottleONE.push(red); System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); bottleONE.push(blue); System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); bottleONE.pop(); bottleONE.pop(); System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); // Bottles declaration StackAsMyArrayList bottles[] = new StackAsMyArrayList[5]; //You can do this with a for also bottles[0] = new StackAsMyArrayList(); bottles[1] = new StackAsMyArrayList(); bottles[2] = new StackAsMyArrayList(); bottles[3] = new StackAsMyArrayList(); bottles[4] = new StackAsMyArrayList(); // Fill 3 bottles with specific colors // need not be uniform colours - just easy to do it with a for Random r = new Random(); char c[] = {red,blue,yellow}; for (int i = 0; i<12; i++) { while(true){ int temp=r.nextInt(5); if(bottles[temp].size() <3){ bottles[temp].push(c[r.nextInt(3)]); break; } } } showAll(bottles); } } From the program add method public static boolean solved (StackAsMyArrayList bottle[ ])which will check if the game is solved
import java.util.*;
public class Watersort {
Character top = null;
// create constants for colors
static Character red= 'r';
static Character blue = 'b';
static Character yellow= 'g';
// Bottles declaratio
public static void showAll( StackAsMyArrayList bottles[])
{
for (int i = 0; i<=4; i++)
{
System.out.println("Bottle "+ i+ ": " + bottles[i]);
}
}
public static void main(String args[])
{
//create the bottle
StackAsMyArrayList bottleONE = new StackAsMyArrayList<Character>();
System.out.println("\nbottleONE:"+ bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
bottleONE.push(red);
bottleONE.push(red);
bottleONE.push(red);
System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
bottleONE.push(blue);
System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
bottleONE.pop();
bottleONE.pop();
System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
// Bottles declaration
StackAsMyArrayList bottles[] = new StackAsMyArrayList[5];
//You can do this with a for also
bottles[0] = new StackAsMyArrayList<Character>();
bottles[1] = new StackAsMyArrayList<Character>();
bottles[2] = new StackAsMyArrayList<Character>();
bottles[3] = new StackAsMyArrayList<Character>();
bottles[4] = new StackAsMyArrayList<Character>();
// Fill 3 bottles with specific colors
// need not be uniform colours - just easy to do it with a for
Random r = new Random();
char c[] = {red,blue,yellow};
for (int i = 0; i<12; i++)
{
while(true){
int temp=r.nextInt(5);
if(bottles[temp].size() <3){
bottles[temp].push(c[r.nextInt(3)]);
break;
}
}
}
showAll(bottles);
}
}
From the program add method public static boolean solved (StackAsMyArrayList bottle[ ])which will check if the game is solved

Step by step
Solved in 2 steps

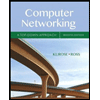
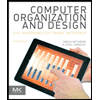
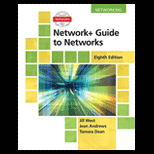
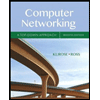
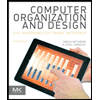
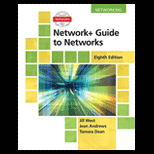
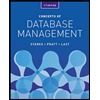
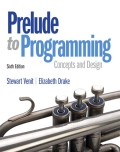
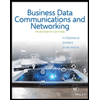