import java.util.Scanner; public class ThreeTestScore { int size = 10; //create arrays for 10 student information String[] fname = new String[size]; String[] mname = new String[size]; String[] lname = new String[size]; int[] score1 = new int[size]; int[] score2 = new int[size]; int[] score3 = new int[size]; //read student informtion public void readStudentInfo(Scanner sc) { for (int i = 0; i < size; i++) { System.out.print("Enter First Name: "); String fname = sc.next(); System.out.print("Enter Middle Initial: "); String mname = sc.next(); System.out.print("Enter Last Name: "); String lname = sc.next(); int score1 = -1, score2 = -1, score3 = -1; do { System.out.print("Enter Score 1(between 0 and 100): "); score1 = sc.nextInt(); } while (score1 < 0 || score1 > 100); do { System.out.print("Enter Score 2(between 0 and 100): "); score2 = sc.nextInt(); } while (score2 < 0 || score2 > 100); do { System.out.print("Enter Score 3(between 0 and 100): "); score3 = sc.nextInt(); } while (score3 < 0 || score3 > 100); this.fname[i] = fname; this.mname[i] = mname; this.lname[i] = lname; this.score1[i] = score1; this.score2[i] = score2; this.score3[i] = score3; System.out.println(); } } public double getAverage(double score1, double score2, double score3) { return (score1 + score2 + score3) / 3; } public double getClassAverage(double value) { return value / size; } public void displayInfo() { System.out.println("========================================================================"); System.out.println("First Name\tMI\tLast Name\tTest 1\tTest 2\tTest 3\t Average"); System.out.println("----------\t----\t---------\t-----\t-----\t------\t--------\t"); double class_avg = 0; for (int i = 0; i < size; i++) { class_avg += getAverage(score1[i], score2[i], score3[i]); System.out.printf("%-10s\t%-2s\t%-10s\t%d\t%d\t%d\t%.2f\n", fname[i], mname[i], lname[i], score1[i], score2[i], score3[i], getAverage(score1[i], score2[i], score3[i])); } System.out.println(""); System.out.printf("class average: %.2f\n\n", getClassAverage(class_avg)); } public static void main(String[] args) { String option = "Yes"; Scanner sc = new Scanner(System.in); do { ThreeTestScore test = new ThreeTestScore(); test.readStudentInfo(sc); test.displayInfo(); System.out.print("Do you want to restart the program?(Y/N): "); option = sc.next().toLowerCase(); } while (!option.equals("n")); } } in Arrays and method
import java.util.Scanner;
public class ThreeTestScore {
int size = 10;
//create arrays for 10 student information
String[] fname = new String[size];
String[] mname = new String[size];
String[] lname = new String[size];
int[] score1 = new int[size];
int[] score2 = new int[size];
int[] score3 = new int[size];
//read student informtion
public void readStudentInfo(Scanner sc) {
for (int i = 0; i < size; i++) {
System.out.print("Enter First Name: ");
String fname = sc.next();
System.out.print("Enter Middle Initial: ");
String mname = sc.next();
System.out.print("Enter Last Name: ");
String lname = sc.next();
int score1 = -1, score2 = -1, score3 = -1;
do {
System.out.print("Enter Score 1(between 0 and 100): ");
score1 = sc.nextInt();
} while (score1 < 0 || score1 > 100);
do {
System.out.print("Enter Score 2(between 0 and 100): ");
score2 = sc.nextInt();
} while (score2 < 0 || score2 > 100);
do {
System.out.print("Enter Score 3(between 0 and 100): ");
score3 = sc.nextInt();
} while (score3 < 0 || score3 > 100);
this.fname[i] = fname;
this.mname[i] = mname;
this.lname[i] = lname;
this.score1[i] = score1;
this.score2[i] = score2;
this.score3[i] = score3;
System.out.println();
}
}
public double getAverage(double score1, double score2, double score3) {
return (score1 + score2 + score3) / 3;
}
public double getClassAverage(double value) {
return value / size;
}
public void displayInfo() {
System.out.println("========================================================================");
System.out.println("First Name\tMI\tLast Name\tTest 1\tTest 2\tTest 3\t Average");
System.out.println("----------\t----\t---------\t-----\t-----\t------\t--------\t");
double class_avg = 0;
for (int i = 0; i < size; i++) {
class_avg += getAverage(score1[i], score2[i], score3[i]);
System.out.printf("%-10s\t%-2s\t%-10s\t%d\t%d\t%d\t%.2f\n", fname[i], mname[i], lname[i], score1[i], score2[i], score3[i], getAverage(score1[i], score2[i], score3[i]));
}
System.out.println("");
System.out.printf("class average: %.2f\n\n", getClassAverage(class_avg));
}
public static void main(String[] args) {
String option = "Yes";
Scanner sc = new Scanner(System.in);
do {
ThreeTestScore test = new ThreeTestScore();
test.readStudentInfo(sc);
test.displayInfo();
System.out.print("Do you want to restart the program?(Y/N): ");
option = sc.next().toLowerCase();
} while (!option.equals("n"));
}
}
in Arrays and method

Step by step
Solved in 3 steps with 2 images

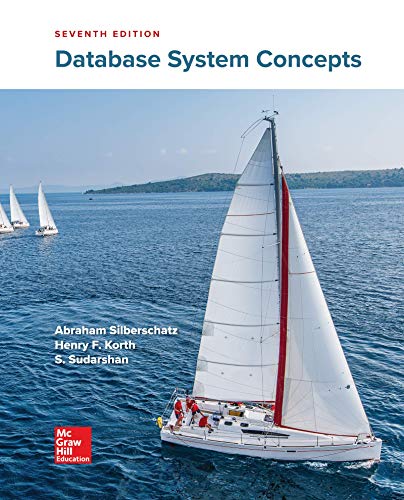
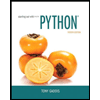
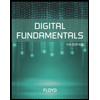
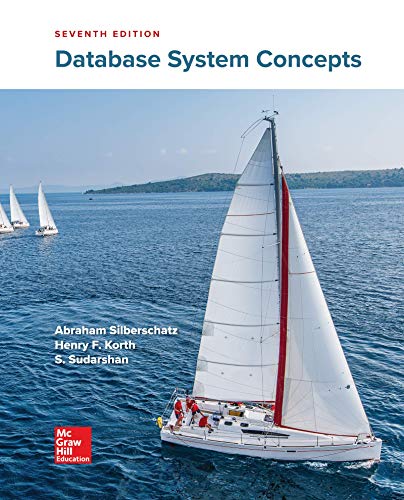
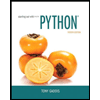
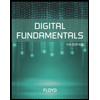
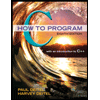
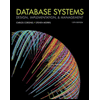
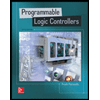