import socket def authenticate_user(tcp_socket): while True: response = tcp_socket.recv(1024).decode() print(response) username = input() tcp_socket.sendall(username.encode()) response = tcp_socket.recv(1024).decode() print(response) password = input() tcp_socket.sendall(password.encode()) auth_response = tcp_socket.recv(1024).decode() if "successful" in auth_response: print(auth_response) return True else: print(auth_response) def receive_items(tcp_socket): while True: response = tcp_socket.recv(1024).decode() if "Item List:" in response: print(response) while True: item = tcp_socket.recv(1024).decode() if not item.strip(): break print(item) break def select_items(tcp_socket): selected_items = [] while True: item_id = input("Select item by entering its ID (0 to finish): ") tcp_socket.sendall(item_id.encode()) if item_id == '0': break response = tcp_socket.recv(1024).decode() if "Invalid item ID" in response: print(response) else: selected_items.append(item_id) return selected_items def receive_receipt(tcp_socket): receipt = tcp_socket.recv(1024).decode() print(receipt) def send_credit_card_details(tcp_socket): credit_card_details = input("Enter encrypted credit card details: ") tcp_socket.sendall(credit_card_details.encode()) print("Credit card details sent.") def main(): # Setup TCP client tcp_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server1_address = ('localhost', 5000) # Server1's address try: tcp_socket.connect(server1_address) print("Connected to Server1.") # User Authentication if authenticate_user(tcp_socket): # Item Listing receive_items(tcp_socket) # Select Items selected_items = select_items(tcp_socket) # Receipt Generation receive_receipt(tcp_socket) # Credit Card Transaction send_credit_card_details(tcp_socket) finally: tcp_socket.close() if __name__ == "__main__": main() This is my client program and it does not let me enter Item number to select after shoing Items list. Example Scenario: Client connects to server1 using TCP Server1 prompts for credentials and validates Upon successful authentication, server1 provides item listing to the client. Client selects items. Server1 generates a receipt and returns it to the client. Client sends encrypted credit card details to server1. Server1 forwards the encrypted details to server2 using UDP. Server2 validates the credit card information and notifies server1. Server1 confirms payment to the client.
import socket
def authenticate_user(tcp_socket):
while True:
response = tcp_socket.recv(1024).decode()
print(response)
username = input()
tcp_socket.sendall(username.encode())
response = tcp_socket.recv(1024).decode()
print(response)
password = input()
tcp_socket.sendall(password.encode())
auth_response = tcp_socket.recv(1024).decode()
if "successful" in auth_response:
print(auth_response)
return True
else:
print(auth_response)
def receive_items(tcp_socket):
while True:
response = tcp_socket.recv(1024).decode()
if "Item List:" in response:
print(response)
while True:
item = tcp_socket.recv(1024).decode()
if not item.strip():
break
print(item)
break
def select_items(tcp_socket):
selected_items = []
while True:
item_id = input("Select item by entering its ID (0 to finish): ")
tcp_socket.sendall(item_id.encode())
if item_id == '0':
break
response = tcp_socket.recv(1024).decode()
if "Invalid item ID" in response:
print(response)
else:
selected_items.append(item_id)
return selected_items
def receive_receipt(tcp_socket):
receipt = tcp_socket.recv(1024).decode()
print(receipt)
def send_credit_card_details(tcp_socket):
credit_card_details = input("Enter encrypted credit card details: ")
tcp_socket.sendall(credit_card_details.encode())
print("Credit card details sent.")
def main():
# Setup TCP client
tcp_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server1_address = ('localhost', 5000) # Server1's address
try:
tcp_socket.connect(server1_address)
print("Connected to Server1.")
# User Authentication
if authenticate_user(tcp_socket):
# Item Listing
receive_items(tcp_socket)
# Select Items
selected_items = select_items(tcp_socket)
# Receipt Generation
receive_receipt(tcp_socket)
# Credit Card Transaction
send_credit_card_details(tcp_socket)
finally:
tcp_socket.close()
if __name__ == "__main__":
main()
This is my client
Example Scenario:
- Client connects to server1 using TCP
- Server1 prompts for credentials and validates
- Upon successful authentication, server1 provides item listing to the client.
- Client selects items.
- Server1 generates a receipt and returns it to the client.
- Client sends encrypted credit card details to server1.
- Server1 forwards the encrypted details to server2 using UDP.
- Server2 validates the credit card information and notifies server1.
- Server1 confirms payment to the client.
Unlock instant AI solutions
Tap the button
to generate a solution
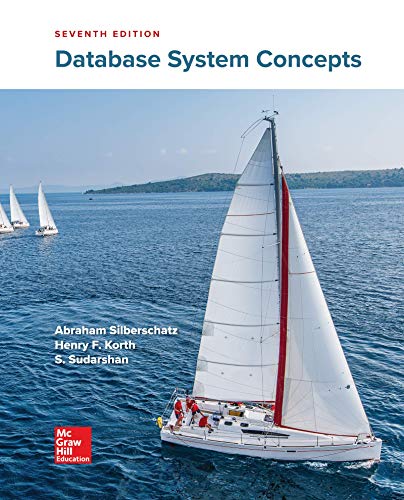
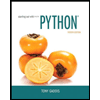
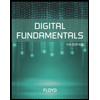
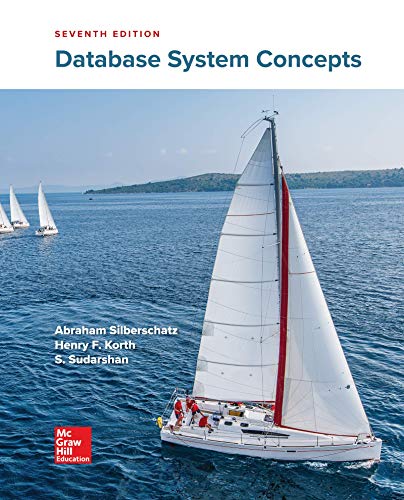
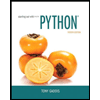
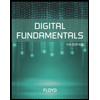
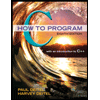
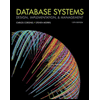
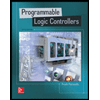