In this assignment you must create a basic arena simulator. There are three main aspects to the assignment. The design and creation of three(3) Character classes that all extend a BaseCharacter class o WarriorClass o MageClass o PriestClass The design and creation of an ArenaManager class that is responsible for managing all of the Characters created. The specific responsibilities are: o To create and store characters. o To allow the viewing and retrieving of characters o To simulate arena challenges (where one character engages another in combat) The design of a basic menu to allow a user to perform and view all of the options of the system. Combat Flow: Combat is split into rounds and continues until either of the Combatant’s presentHP goes to 0 (or below). Each round of combat must be performed as follows: At the beginning of each round, a random character goes first (50 percent chance of either going first). We will call the winner of the initiative check “attacker” and the other character “counterattacker” for this description. 1. The attacker performs an attack using its getAttack() method. 2. After an attack is made, the target can dodge the attack . This is done by performing a roll check out of 100. If the roll is less than the target’s dodgeChance, the target has successfully dodged and takes no damage. If the target does not successfully dodge, it takes damage. 3. If the counterattacker has presentHP more than 0, steps 1 and 2 are performed by the counterattacker. 4. A summary of the round of combat must be displayed. At the end of combat, a clear indication of the winner must be displayed. In your main program, you must present a menu with functionality as shown below: 1) Add Contestant 2) View Specific Contestant 3) View All Contestants 4) Run Combat Challenge 5) Exit
In this assignment you must create a basic arena simulator. There are three main aspects to the assignment. The design and creation of three(3) Character classes that all extend a BaseCharacter class o WarriorClass o MageClass o PriestClass The design and creation of an ArenaManager class that is responsible for managing all of the Characters created. The specific responsibilities are: o To create and store characters. o To allow the viewing and retrieving of characters o To simulate arena challenges (where one character engages another in combat) The design of a basic menu to allow a user to perform and view all of the options of the system. Combat Flow: Combat is split into rounds and continues until either of the Combatant’s presentHP goes to 0 (or below). Each round of combat must be performed as follows: At the beginning of each round, a random character goes first (50 percent chance of either going first). We will call the winner of the initiative check “attacker” and the other character “counterattacker” for this description. 1. The attacker performs an attack using its getAttack() method. 2. After an attack is made, the target can dodge the attack . This is done by performing a roll check out of 100. If the roll is less than the target’s dodgeChance, the target has successfully dodged and takes no damage. If the target does not successfully dodge, it takes damage. 3. If the counterattacker has presentHP more than 0, steps 1 and 2 are performed by the counterattacker. 4. A summary of the round of combat must be displayed. At the end of combat, a clear indication of the winner must be displayed. In your main program, you must present a menu with functionality as shown below: 1) Add Contestant 2) View Specific Contestant 3) View All Contestants 4) Run Combat Challenge 5) Exit
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In this assignment you must create a basic arena simulator. There are three main aspects to the assignment. The design and creation of three(3) Character classes that all extend a BaseCharacter class o WarriorClass o MageClass o PriestClass The design and creation of an ArenaManager class that is responsible for managing all of the Characters created. The specific responsibilities are: o To create and store characters. o To allow the viewing and retrieving of characters o To simulate arena challenges (where one character engages another in combat) The design of a basic menu to allow a user to perform and view all of the options of the system. Combat Flow: Combat is split into rounds and continues until either of the Combatant’s presentHP goes to 0 (or below). Each round of combat must be performed as follows: At the beginning of each round, a random character goes first (50 percent chance of either going first). We will call the winner of the initiative check “attacker” and the other character “counterattacker” for this description. 1. The attacker performs an attack using its getAttack() method. 2. After an attack is made, the target can dodge the attack . This is done by performing a roll check out of 100. If the roll is less than the target’s dodgeChance, the target has successfully dodged and takes no damage. If the target does not successfully dodge, it takes damage. 3. If the counterattacker has presentHP more than 0, steps 1 and 2 are performed by the counterattacker. 4. A summary of the round of combat must be displayed. At the end of combat, a clear indication of the winner must be displayed. In your main program, you must present a menu with functionality as shown below: 1) Add Contestant 2) View Specific Contestant 3) View All Contestants 4) Run Combat Challenge 5) Exit

Transcribed Image Text:Additional Information about the behaviour of each polymorphic method.
BaseCharacter
Class
getAttack():AttackAttackinfo
100% chance of a primary attack
primaryAttack():AttackInfo
returns an AttackInfo object with: Attackinfo object with:
damage baseDamage
description = " strikes at"
secondaryAttack():Attackinfo
takeDamage(amt :int)
presentHp is reduced by amt
Warrior
Mage
90% chance of primary attack and 10% chance 70 % chance of primary attack
of secondary attack
and 30% chance of secondary
attack
damage = baseDamage + baseDamage*
(strength/20)
description = "strikes at"
Raises dodge value by agility/2 permanently
(dodge can be raised to maximum of 50) and
AttackInfo object with:
Attackinfo object with:
damage =
baseDamage+baseDamage*
(intelligence/20)
description = "throws a fireball
at"
damage = 2 (the actual number 2)
description = "gets more agile and leaps at"
All damage (amt) is reduced by the resistance
as a percentage (resistance %) before applying
Priest
60% chance of primary attack
and 40% chance of secondary
attack
Heals for( 5+ intelligence/20)
percent of max hitpoints and
Attackinfo object with:
damage set as 0
description = "heals
themselves and smiles at"

Transcribed Image Text:Summary of the state values (attributes)
Data
State
value/Attribute:
type
Bounds and
Description
notes
name
string
name of the character for example "Orc General" or "Vildred"
strength
int
strength of the character.
maximum 20
agility
int
agility of the character
maximum 20
intelligence
int
intelligence of the character
maximum 20
maxHp
int
maximum hit points the character can have
presentHp
int
present hit points of the character
dodgeChance
represents the percent chance of a character dodging an
attack
int
maximum 50
int
basic physical damage of the character
baseDamage
resistance
int
Resistance to damage (only applies to the Warrior class)
Maximum 50
Combat Flow:
Combat is split into rounds and continues until either of the Combatant's presentHP goes to 0 (or below).
Each round of combat must be performed as follows:
At the beginning of each round, a random character goes first (50 percent chance of either going first).
We will call the winner of the initiative check "attacker" and the other character "counterattacker" for this
description.
1. The attacker performs an attack using its getAttack() method.
2. After an attack is made, the target can dodge the attack. This is done by performing a roll check out of 100.
If the roll is less than the target's dodgeChance, the target has successfully dodged and takes no damage.
If the target does not successfully dodge, it takes damage.
3. If the counterattacker has presentHP more than 0, steps 1 and 2 are performed by the counterattacker.
4. A summary of the round of combat must be displayed.
At the end of combat, a clear indication of the winner must be displayed.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 7 steps with 3 images

Recommended textbooks for you
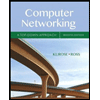
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
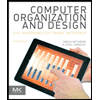
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
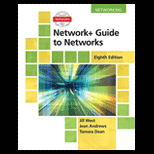
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
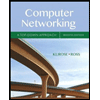
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
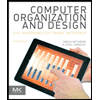
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
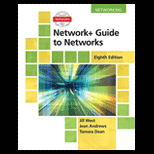
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
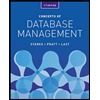
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
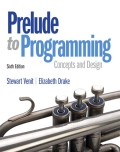
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
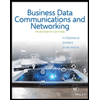
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY