In this assignment you will write a C++ program that can figure out a number chosen by a human user. The human user will think of a number between 1 and 100. The computer program will make guesses and the user will tell the program to guess higher or lower. A sample run of the program should look something like this, with the user inputs in orange: Think of a number between 1 and 100. Is it 50? (h/l/c): h Is it 75? (h/l/c): h Is it 88? (h/l/c): l Is it 81? (h/l/c): c Great! Do you want to play again? (y/n): y Think of a number between 1 and 100. Is it 50? (h/l/c): l Is it 25? (h/l/c): h Is it 37? (h/l/c): c Great! Do you want to play again? (y/n): n Notice that the user inputs the characters ‘h’, ‘l’, and ‘c’ for higher, lower, and correct, respectively. Every time the program makes a guess, it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50, so that's the computer's first guess. The user responds by saying 'h' for “higher” On the second guess the possible values are 51 to 100. The midpoint is 75. The user responds by saying 'h' for “higher” On the third guess the possible values are 76 to 100. The midpoint is 88. The user responds by saying 'l' for “lower” On the fourth guess the possible values are 76 to 87. The midpoint is 81. The user responds 'c' for “correct” Requirements The purpose of the assignment is to practice writing functions. Although it would be possible to write the entire program in the main() function, your solution should be heavily structured with functions. To receive credit, your main function must look like this: int main() { do { playOneGame(); } while (shouldPlayAgain()); return 0; } You must also have the following functions specified below: The playOneGame() function has a return type of void. It implements a complete guessing game on the range of 1 to 100. The shouldPlayAgain() function has a bool return type. It prompts the user to determine if the user wants to play again, reads in a character, returns true if the character is a ‘y’, and otherwise returns false. In addition, implement the helper functions getUserResponseToGuess(), and getMidpoint(). They should be called inside your playOneGame() function. getUserResponseToGuess. This function takes a parameter guess of type int. It prompts the user with the phrase “is it guess? (h/l/c): “ and returns a char. The char should be one of three possible values: ‘h’, ‘l’, or ‘c’. For example, if parameter guess is 75, the prompt would be "is it 75? (h/l/c):" It has the following signature: char getUserResponseToGuess(int guess) getMidpoint. This function takes two int parameters, and returns the midpoint of the two integers. It has the following signature: int getMidpoint(int low, int high) Suggestions Test your functions one by one as you write them. Once you're sure they're all working you can put them all together to make the whole game work. What to submit Submit a file with your program and sample output demonstrating program behavior with a couple games played, in one big .cpp file (not .rtf, .pdf, or .doc file). Optional but Recommended For extra practice, write a function named getRandomMidpoint. This function should accept two arguments just like getMidpoint. But it should return a random number in between the low and high arguments. You can swap this function with the getMidpoint function to change the nature of how the program makes its guesses. To get extra credit, make sure to show output of your program using both getMidpoint and getRandomMidpoint showing different behaviors. Don't forget to add a call to srand() at the beginning of your program in main() to make sure each run of the program generates new "random" numbers.
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
In this assignment you will write a C++
A sample run of the program should look something like this, with the user inputs in orange:
Think of a number between 1 and 100.
Is it 50? (h/l/c): h
Is it 75? (h/l/c): h
Is it 88? (h/l/c): l
Is it 81? (h/l/c): c
Great! Do you want to play again? (y/n): y
Think of a number between 1 and 100.
Is it 50? (h/l/c): l
Is it 25? (h/l/c): h
Is it 37? (h/l/c): c
Great! Do you want to play again? (y/n): n
Notice that the user inputs the characters ‘h’, ‘l’, and ‘c’ for higher, lower, and correct, respectively.
Every time the program makes a guess, it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81:
On the first guess, the possible values are 1 to 100. The midpoint is 50, so that's the computer's first guess.
The user responds by saying 'h' for “higher”
On the second guess the possible values are 51 to 100. The midpoint is 75.
The user responds by saying 'h' for “higher”
On the third guess the possible values are 76 to 100. The midpoint is 88.
The user responds by saying 'l' for “lower”
On the fourth guess the possible values are 76 to 87. The midpoint is 81.
The user responds 'c' for “correct”
Requirements
The purpose of the assignment is to practice writing functions. Although it would be possible to write the entire program in the main() function, your solution should be heavily structured with functions. To receive credit, your main function must look like this:
int main()
{
do
{
playOneGame();
} while (shouldPlayAgain());
return 0;
}
You must also have the following functions specified below:
The playOneGame() function has a return type of void. It implements a complete guessing game on the range of 1 to 100.
The shouldPlayAgain() function has a bool return type. It prompts the user to determine if the user wants to play again, reads in a character, returns true if the character is a ‘y’, and otherwise returns false.
In addition, implement the helper functions getUserResponseToGuess(), and getMidpoint(). They should be called inside your playOneGame() function.
getUserResponseToGuess. This function takes a parameter guess of type int. It prompts the user with the phrase “is it guess? (h/l/c): “ and returns a char. The char should be one of three possible values: ‘h’, ‘l’, or ‘c’. For example, if parameter guess is 75, the prompt would be "is it 75? (h/l/c):" It has the following signature:
char getUserResponseToGuess(int guess)
getMidpoint. This function takes two int parameters, and returns the midpoint of the two integers. It has the following signature:
int getMidpoint(int low, int high)
Suggestions
Test your functions one by one as you write them. Once you're sure they're all working you can put them all together to make the whole game work.
What to submit
Submit a file with your program and sample output demonstrating program behavior with a couple games played, in one big .cpp file (not .rtf, .pdf, or .doc file).
Optional but Recommended
For extra practice, write a function named getRandomMidpoint. This function should accept two arguments just like getMidpoint. But it should return a random number in between the low and high arguments. You can swap this function with the getMidpoint function to change the nature of how the program makes its guesses. To get extra credit, make sure to show output of your program using both getMidpoint and getRandomMidpoint showing different behaviors. Don't forget to add a call to srand() at the beginning of your program in main() to make sure each run of the program generates new "random" numbers.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

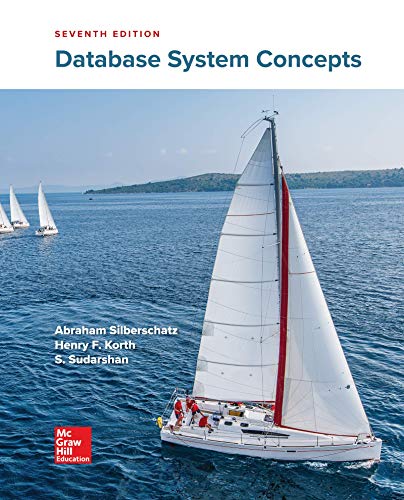
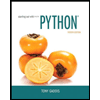
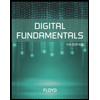
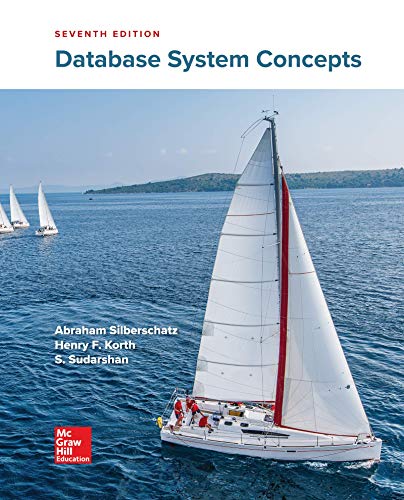
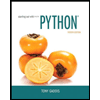
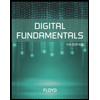
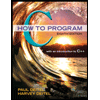
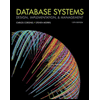
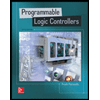