In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:
In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:
Chapter7: Characters, Strings, And The Stringbuilder
Section: Chapter Questions
Problem 7PE
Related questions
Question
In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below:

Transcribed Image Text:At the beginning, your program should draw an empty chess board and prompt the user to enter a move:
Welcome to the Chess Game!
a
b с d e
f
9
h
81
I I
18
71
T
17
61
I
16
51 T
15
41 I
14
31 T
13
21 I
|
12
11
I
|
I
11
a b с
de f g h
Enter a chess piece and its position or type X to exit:
A move is represented as a three-letter string where the first letter corresponds to a chess piece initial: K
stands for king, Q - queen, B - bishop, N - knight, and R - rook (a chariot). Pawns do not have initials, and
you will not be tested on their implementation. The next letter in the move is the position on the board
that corresponds to one of eight columns (called files). The columns (files) are labeled as a, b, c, d, e, f, g, h.
The third symbol is a digit that corresponds to one of eight rows (called ranks). The rows (ranks) are labeled
as 1, 2, 3, 4, 5, 6, 7, 8. For example, if the user enters Re6, your program should generate the following
output where R is the initial for a rook, and all possible moves of the rook are marked by x:
a
b
с
de f
g h
81
1
1
|
| x |
1
| 18
71
|
|
I
| x |
I I 17
61 x | x | x | x |R|x | x | x 16
51
T
Ix
15
41
14
31
I
X
| 1
13
21
|
| x |
I
I
12
11
|
Ix
| |
11
--+
a b с
d e f g
h
Enter a chess piece and its position or type X to exit:
If the user enters Kd5, your program should produce the following output where K is the initial for the
king, and all squares where the king can move are marked with x:
a
b
с
e
g h
81
| T
18
71
|
17
61 | |xx|x I
I
|
16
51 I IxIKIx
15
41
1 | x | x | x |
|
|
14
31
I 1
13
21
1
| 1
I I
12
11
I
11
a
b с
d e f g h
Enter a chess piece and its position or type X to exit:
|
I
|
I
![If the user does not enter a valid move that is included in all possible combinations of chess piece initials
(K, Q, B, N, R), files (a, b, c, d, e, f, g, h), and ranks (1, 2, 3, 4, 5, 6, 7, 8), then the program should reprint the
prompt:
Enter a chess piece and its position or type X to exit:
If the user enters X (or x), then the program should print 'Goodbye!' and terminate:
Goodbye!
NOTE: The user can use either upper or lowercase letters, for example X or x should result in the
termination of the program, Ke3, KE3, KE3, or ke3 means the same move.
Programming Approaches
In this assignment, you need to create a class Board and a class for each chess piece: King, Queen, Bishop,
Knight, and Rook. They all should be located in a module called chess.py. All chess piece classes should be
derived from the superclass Chess_Piece given to you.
Your class Board should have four methods init, empty, set, and draw. Three of the methods are already
implemented for you. You only need to implement the method draw() that should print the board layout
and label squares accordingly to the current chess piece positioned on the board. You can type or copy
and paste the following code into your file chess.py.
The given class Board has only one attribute that is also called board, and it is implemented as a dictionary.
The method empty() generates an empty board (a dictionary with keys corresponding to chess board
squares, and their values are white spaces (' '). The method set() updates the label of a given square by
assigning the dictionary key value to its new value.
class Board:
definit__(self):
self.board = {}
self.empty()
def empty(self):
for col in 'abcdefgh':
for row in '12345678':
self.board [col+row] =
#pos is a square label (al, a2,
h8)
def set(self, pos, piece):
if pos in self.board.keys():
self.board [pos] = piece
def draw(self):
The class Chess_Piece has four methods init, get_index, get_name, and moves. The constructor method
init() creates an object with attributes position and color and updates the board by placing the chess piece
on the board at a given position. The method get_name() should return the initial of the chess piece, and it
is not implemented in the superclass. However, you need to implement it in all subclasses, for example, the
method in a King class should return 'K', in a Rook class - 'R', etc. The method get_index() converts a
position (that is used as a key in the board dictionary) into a tuple of indexes where the first index is a
column number, and the second index is a row number). It is useful to use indexes (integers) instead of
strings to calculate possible moves of a chess piece because we can use addition and subtraction
operations on indexes. For example, to get a move of a knight located at e3, we can get its position as
indexes: 'e' has index 4, and '3' has index 2. Then, we can add 2 to the index of 'e' and 1 to the index of '3'
and get a new position (6, 3) that corresponds to g4.
The method moves() is not implemented. It is unique for each class, and it should label the board with the
corresponding letters and 'x's. Your task is to find how to calculate indexes of possible moves. Hints: You
can traverse squares on the board and check if a square has indexes that correspond to a possible move. A
possible move for a rook is any square that has the same row or column index as the current position of
the rook. A possible move for the king is a square that is one distance away from the king's current
position. A possible move for a knight is a square that is two distances away in one direction and one
distance away in another direction.
class Chess_Piece:
definit__(self, board, pos, color='white'):
self.position = self.get_index(pos)
self.color = color
board.set(pos, self.get_name())
def get_index(self, pos):
return ('abcdefgh'.index(pos[0]), '12345678'.index(pos[1]))
def get_name(self):
pass
dof mover(colf board):](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdd3d3176-2cf4-4554-91a8-a12c09f2d35b%2F6884ab95-ede7-4f15-9f3d-0f298d7faa18%2Fxh45yvt_processed.png&w=3840&q=75)
Transcribed Image Text:If the user does not enter a valid move that is included in all possible combinations of chess piece initials
(K, Q, B, N, R), files (a, b, c, d, e, f, g, h), and ranks (1, 2, 3, 4, 5, 6, 7, 8), then the program should reprint the
prompt:
Enter a chess piece and its position or type X to exit:
If the user enters X (or x), then the program should print 'Goodbye!' and terminate:
Goodbye!
NOTE: The user can use either upper or lowercase letters, for example X or x should result in the
termination of the program, Ke3, KE3, KE3, or ke3 means the same move.
Programming Approaches
In this assignment, you need to create a class Board and a class for each chess piece: King, Queen, Bishop,
Knight, and Rook. They all should be located in a module called chess.py. All chess piece classes should be
derived from the superclass Chess_Piece given to you.
Your class Board should have four methods init, empty, set, and draw. Three of the methods are already
implemented for you. You only need to implement the method draw() that should print the board layout
and label squares accordingly to the current chess piece positioned on the board. You can type or copy
and paste the following code into your file chess.py.
The given class Board has only one attribute that is also called board, and it is implemented as a dictionary.
The method empty() generates an empty board (a dictionary with keys corresponding to chess board
squares, and their values are white spaces (' '). The method set() updates the label of a given square by
assigning the dictionary key value to its new value.
class Board:
definit__(self):
self.board = {}
self.empty()
def empty(self):
for col in 'abcdefgh':
for row in '12345678':
self.board [col+row] =
#pos is a square label (al, a2,
h8)
def set(self, pos, piece):
if pos in self.board.keys():
self.board [pos] = piece
def draw(self):
The class Chess_Piece has four methods init, get_index, get_name, and moves. The constructor method
init() creates an object with attributes position and color and updates the board by placing the chess piece
on the board at a given position. The method get_name() should return the initial of the chess piece, and it
is not implemented in the superclass. However, you need to implement it in all subclasses, for example, the
method in a King class should return 'K', in a Rook class - 'R', etc. The method get_index() converts a
position (that is used as a key in the board dictionary) into a tuple of indexes where the first index is a
column number, and the second index is a row number). It is useful to use indexes (integers) instead of
strings to calculate possible moves of a chess piece because we can use addition and subtraction
operations on indexes. For example, to get a move of a knight located at e3, we can get its position as
indexes: 'e' has index 4, and '3' has index 2. Then, we can add 2 to the index of 'e' and 1 to the index of '3'
and get a new position (6, 3) that corresponds to g4.
The method moves() is not implemented. It is unique for each class, and it should label the board with the
corresponding letters and 'x's. Your task is to find how to calculate indexes of possible moves. Hints: You
can traverse squares on the board and check if a square has indexes that correspond to a possible move. A
possible move for a rook is any square that has the same row or column index as the current position of
the rook. A possible move for the king is a square that is one distance away from the king's current
position. A possible move for a knight is a square that is two distances away in one direction and one
distance away in another direction.
class Chess_Piece:
definit__(self, board, pos, color='white'):
self.position = self.get_index(pos)
self.color = color
board.set(pos, self.get_name())
def get_index(self, pos):
return ('abcdefgh'.index(pos[0]), '12345678'.index(pos[1]))
def get_name(self):
pass
dof mover(colf board):
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
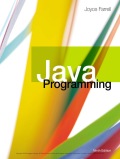
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
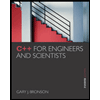
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
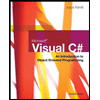
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
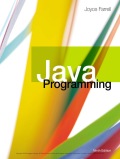
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
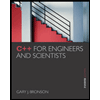
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
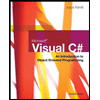
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
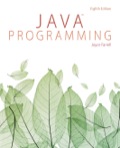
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT