In this project, you should design a Date class and use it to create a calendar application that has many features. You are not limited to the features included in these instructions, feel free to add any other components you find useful.
In this project, you should design a Date class and use it to create a calendar application that has many features. You are not limited to the features included in these instructions, feel free to add any other components you find useful.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:In this project, you should design a Date class and use it to create a calendar application that has
many features. You are not limited to the features included in these instructions, feel free to add
any other components you find useful.
Note: A normal year has 365 days. A leap year has 366 days (the extra day is February 29). In this
project, you must take this fact into account. The rules that determine leap years are provided later
in these instructions.
Design the Date class, to be used in your application, containing:
A private data member month of type integer that holds the date's month.
A private data member day of type integer that holds the date's day.
A private data member year of type integer that holds the date’s year.
A default constructor that sets the date to January 1, 1753.
Accessors for each member variable.
Mutators for each member variable.
A member function that prints on the screen a date in the form mm / dd / yyyy.
A member function that returns the day name of a date (ex, Sunday, Monday ...). Note that
the day for January 1 of the year 1753 was a Monday.
• A member function that prints a date including the name of the day and the name of the
month as strings (ex: Tuesday, April 6, 2021).
The overloaded subtraction operator ( – ) that returns an integer that is the difference in
days between two Date objects.
The overloaded increment operator ( ++ ) that modifies the Date object so that it represents
the next day.
The overloaded decrement operator ( -- ) that modifies the Date object so that it represents
the previous day. The operator must not decrement dates prior to January 1, 1753.

Transcribed Image Text:Write a program that thoroughly tests your Date class. Consider using the following functions in
your program (you can use more functions). The implementations of these functions are left to
you; they can be members of the Date class, friends of it, or just regular stand-alone functions.
A function that tests whether a year is leap.
A function that calculates and returns a date by adding a fixed number of days to the current
date.
A function that calculates and returns a date by subtracting a fixed number of days from
the current date.
A function that returns the number of days passed in the current year.
A function that returns the number of days remaining in the current year.
A function that prints the calendar for the current month.
A function that prints the holidays of any year.
Rules for determining leap years:
Leap Years are any year that can be exactly divided by 4 (such as 2016, 2020, 2024, etc)
X Except if it can be exactly divided by 100, then it isn't (such as 2100, 2200, 2300, etc)
V Except if it can be exactly divided by 400, then it is (such as 2000, 2400, 2800, etc)
List of US federal holidays:
New Year's Day - January 1$st
Martin Luther King, Jr. Day - Third Monday of January
President's Day - Third Monday of February
Memorial Day - Last Monday of May
Independence Day - July 4th
Labor Day - First Monday of September
Columbus Day - Second Monday of October
Veterans Day - November 11th
Thanksgiving Day - Fourth Thursday of November
Christmas Day - December 25th
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
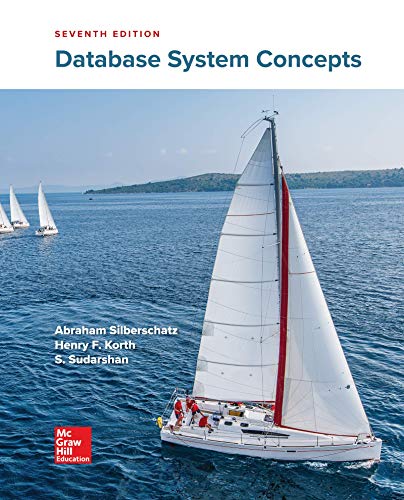
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
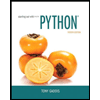
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
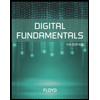
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
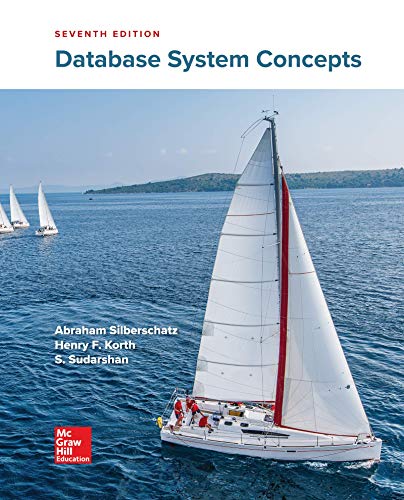
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
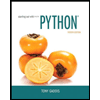
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
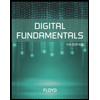
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
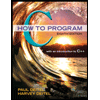
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
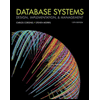
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
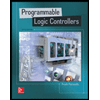
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education