In this question, we are going to write a Racket function wordMaxIndex that calculates the maximum index of each word that appears in an input word list. For example, if the input is ’(time is long but life is short) Then one output can be ((time 0) (long 2) (but 3) (life 4) (is 5) (short 6)) The index of the first word in a word list is 0; the index for the second word is 1; etc. Therefore, in the previous example, the maximum index for word “time” is 0; the maximum index for word “is” is 5, since “is” appears twice, at index 1 and 5. Note that you are not asked to sort the words in the output. Therefore, the output is correct as long as the indexes are correct. Do the following steps to implement the word-index program. In our discussion, we call a word with an index a word-index pair, for example, (short 6) and (is 5) are word-index pairs. We call a list of word- index pairs a word-index list. (a) Write a function initialWIList that takes a list of words and creates a word-index list. The resulting word-index list should have the right index for every word in the list. For instance, (initialWIList ’(time is long but life is short)) should generate the following output: ((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6)) Hint: develop initialWIList on top of a helper function initialWIListHelper, which takes an index k and a word list l as input. It assumes that the first word in l has index k and generates the right word-index list. For instance, (initialWIListHelper 4 ’(life is short)) should generate the following output: ((life 4) (is 5) (short 6)) (b) Write a function mergeWI. It takes two inputs. The first is a word-index pair and the second is a word-index list. This function generates a new word-index list. If the input word- index pair has a corresponding pair in the word-index list with the same word, then only the pair with the bigger index should be kept; otherwise, the output word-index list should have the input word-index list with the word-index pair at the end of the list. For instance, (mergeWI ’(is 1) ’((time 0) (is 5))) should generate ((time 0) (is 5)) As another example (mergeWI ’(life 4) ’((time 0) (is 5))) should generate ((time 0) (is 5) (life 4)) (c) (2 points) Write a function mergeByWord, which takes a word- index list and produces a new word-index list; the output word- index list should have one word-index pair for each word that appears in the input list and the index should be the maximum index of all indexes for that word in the input list. For instance, if the input list is ((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6)) then the output should be ((time 0) (long 2) (but 3) (life 4) (is 5) (short 6)) Write mergeByWord based on the reduce function we discussed in class and mergeWI. The reduce function is not built-in in Racket; you can type it in yourself: (define (reduce f l v) (if (null? l) v (f (car l) (reduce f (cdr l) v)))) (d) Finally, write a wordMaxIndex function that takes in
In this question, we are going to write a Racket function wordMaxIndex that calculates the maximum index of each word that appears in an input word list. For example, if the input is ’(time is long but life is short) Then one output can be ((time 0) (long 2) (but 3) (life 4) (is 5) (short 6)) The index of the first word in a word list is 0; the index for the second word is 1; etc. Therefore, in the previous example, the maximum index for word “time” is 0; the maximum index for word “is” is 5, since “is” appears twice, at index 1 and 5. Note that you are not asked to sort the words in the output. Therefore, the output is correct as long as the indexes are correct. Do the following steps to implement the word-index program. In our discussion, we call a word with an index a word-index pair, for example, (short 6) and (is 5) are word-index pairs. We call a list of word- index pairs a word-index list. (a) Write a function initialWIList that takes a list of words and creates a word-index list. The resulting word-index list should have the right index for every word in the list. For instance, (initialWIList ’(time is long but life is short)) should generate the following output: ((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6)) Hint: develop initialWIList on top of a helper function initialWIListHelper, which takes an index k and a word list l as input. It assumes that the first word in l has index k and generates the right word-index list. For instance, (initialWIListHelper 4 ’(life is short)) should generate the following output: ((life 4) (is 5) (short 6)) (b) Write a function mergeWI. It takes two inputs. The first is a word-index pair and the second is a word-index list. This function generates a new word-index list. If the input word- index pair has a corresponding pair in the word-index list with the same word, then only the pair with the bigger index should be kept; otherwise, the output word-index list should have the input word-index list with the word-index pair at the end of the list. For instance, (mergeWI ’(is 1) ’((time 0) (is 5))) should generate ((time 0) (is 5)) As another example (mergeWI ’(life 4) ’((time 0) (is 5))) should generate ((time 0) (is 5) (life 4)) (c) (2 points) Write a function mergeByWord, which takes a word- index list and produces a new word-index list; the output word- index list should have one word-index pair for each word that appears in the input list and the index should be the maximum index of all indexes for that word in the input list. For instance, if the input list is ((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6)) then the output should be ((time 0) (long 2) (but 3) (life 4) (is 5) (short 6)) Write mergeByWord based on the reduce function we discussed in class and mergeWI. The reduce function is not built-in in Racket; you can type it in yourself: (define (reduce f l v) (if (null? l) v (f (car l) (reduce f (cdr l) v)))) (d) Finally, write a wordMaxIndex function that takes in
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 12E: (Program) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
In this question, we are going to write a Racket function
wordMaxIndex that calculates the maximum index of each word that
appears in an input word list. For example, if the input is
’(time is long but life is short)
Then one output can be
((time 0) (long 2) (but 3) (life 4) (is 5) (short 6))
The index of the first word in a word list is 0; the index for the second
word is 1; etc. Therefore, in the previous example, the maximum index
for word “time” is 0; the maximum index for word “is” is 5, since “is”
appears twice, at index 1 and 5. Note that you are not asked to sort
the words in the output. Therefore, the output is correct as long as
the indexes are correct.
Do the following steps to implement the word-index program. In our
discussion, we call a word with an index a word-index pair, for example,
(short 6) and (is 5) are word-index pairs. We call a list of word-
index pairs a word-index list.
(a) Write a function initialWIList that takes a list of
words and creates a word-index list. The resulting word-index
list should have the right index for every word in the list. For
instance,
wordMaxIndex that calculates the maximum index of each word that
appears in an input word list. For example, if the input is
’(time is long but life is short)
Then one output can be
((time 0) (long 2) (but 3) (life 4) (is 5) (short 6))
The index of the first word in a word list is 0; the index for the second
word is 1; etc. Therefore, in the previous example, the maximum index
for word “time” is 0; the maximum index for word “is” is 5, since “is”
appears twice, at index 1 and 5. Note that you are not asked to sort
the words in the output. Therefore, the output is correct as long as
the indexes are correct.
Do the following steps to implement the word-index program. In our
discussion, we call a word with an index a word-index pair, for example,
(short 6) and (is 5) are word-index pairs. We call a list of word-
index pairs a word-index list.
(a) Write a function initialWIList that takes a list of
words and creates a word-index list. The resulting word-index
list should have the right index for every word in the list. For
instance,
(initialWIList ’(time is long but life is short))
should generate the following output:
((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6))
Hint: develop initialWIList on top of a helper function initialWIListHelper,
which takes an index k and a word list l as input. It assumes that
the first word in l has index k and generates the right word-index
list. For instance,
(initialWIListHelper 4 ’(life is short))
should generate the following output:
((life 4) (is 5) (short 6))
(b) Write a function mergeWI. It takes two inputs. The
first is a word-index pair and the second is a word-index list.
This function generates a new word-index list. If the input word-
index pair has a corresponding pair in the word-index list with
the same word, then only the pair with the bigger index should
be kept; otherwise, the output word-index list should have the
input word-index list with the word-index pair at the end of the
list. For instance,
(mergeWI ’(is 1) ’((time 0) (is 5)))
should generate
((time 0) (is 5))
As another example
(mergeWI ’(life 4) ’((time 0) (is 5)))
should generate
((time 0) (is 5) (life 4))
should generate the following output:
((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6))
Hint: develop initialWIList on top of a helper function initialWIListHelper,
which takes an index k and a word list l as input. It assumes that
the first word in l has index k and generates the right word-index
list. For instance,
(initialWIListHelper 4 ’(life is short))
should generate the following output:
((life 4) (is 5) (short 6))
(b) Write a function mergeWI. It takes two inputs. The
first is a word-index pair and the second is a word-index list.
This function generates a new word-index list. If the input word-
index pair has a corresponding pair in the word-index list with
the same word, then only the pair with the bigger index should
be kept; otherwise, the output word-index list should have the
input word-index list with the word-index pair at the end of the
list. For instance,
(mergeWI ’(is 1) ’((time 0) (is 5)))
should generate
((time 0) (is 5))
As another example
(mergeWI ’(life 4) ’((time 0) (is 5)))
should generate
((time 0) (is 5) (life 4))
(c) (2 points) Write a function mergeByWord, which takes a word-
index list and produces a new word-index list; the output word-
index list should have one word-index pair for each word that
appears in the input list and the index should be the maximum
index of all indexes for that word in the input list. For instance,
if the input list is
((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6))
then the output should be
((time 0) (long 2) (but 3) (life 4) (is 5) (short 6))
Write mergeByWord based on the reduce function we discussed in
class and mergeWI. The reduce function is not built-in in Racket;
you can type it in yourself:
(define (reduce f l v)
(if (null? l) v
(f (car l) (reduce f (cdr l) v))))
(d) Finally, write a wordMaxIndex function that takes in
a list of words and outputs the right word-index list; that is, for
each word in the input, the output word-index list should contain
a pair for the word with the maximum index. Write this function
based on initialWIList and mergeByWord.
index list and produces a new word-index list; the output word-
index list should have one word-index pair for each word that
appears in the input list and the index should be the maximum
index of all indexes for that word in the input list. For instance,
if the input list is
((time 0) (is 1) (long 2) (but 3) (life 4) (is 5) (short 6))
then the output should be
((time 0) (long 2) (but 3) (life 4) (is 5) (short 6))
Write mergeByWord based on the reduce function we discussed in
class and mergeWI. The reduce function is not built-in in Racket;
you can type it in yourself:
(define (reduce f l v)
(if (null? l) v
(f (car l) (reduce f (cdr l) v))))
(d) Finally, write a wordMaxIndex function that takes in
a list of words and outputs the right word-index list; that is, for
each word in the input, the output word-index list should contain
a pair for the word with the maximum index. Write this function
based on initialWIList and mergeByWord.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
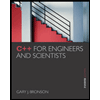
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
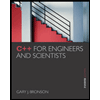
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr