Instructions In Chapter 4, we developed an algorithm for converting from binary to decimal. You can generalize this algorithm to work for a representation in any base. Instead of using a power of 2, you will write a program that converts any base between 2 and 16. Recall that for numbers 10-15 we use letters A - F. In convert.py, define a function named repToDecimal that expects two arguments, a string, and an integer. The second argument should be the base. For example, repToDecimal("10", 8) returns 8, whereas repToDecimal("10", 16) returns 16. convert.py + 1 # Put your code here 2 3 # A main for testing your program 4 def main(): 5 6 7 """Tests the function. """ print (repToDecimal('10', 10)) print (repToDecimal('10', 8)) print (repToDecimal('10', 2)) print (repToDecimal('10', 16)) 8 9 10 11 # The entry point for program execution 12 if __name__ == "__main__": 13 main()
Instructions In Chapter 4, we developed an algorithm for converting from binary to decimal. You can generalize this algorithm to work for a representation in any base. Instead of using a power of 2, you will write a program that converts any base between 2 and 16. Recall that for numbers 10-15 we use letters A - F. In convert.py, define a function named repToDecimal that expects two arguments, a string, and an integer. The second argument should be the base. For example, repToDecimal("10", 8) returns 8, whereas repToDecimal("10", 16) returns 16. convert.py + 1 # Put your code here 2 3 # A main for testing your program 4 def main(): 5 6 7 """Tests the function. """ print (repToDecimal('10', 10)) print (repToDecimal('10', 8)) print (repToDecimal('10', 2)) print (repToDecimal('10', 16)) 8 9 10 11 # The entry point for program execution 12 if __name__ == "__main__": 13 main()
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In Python.

Transcribed Image Text:Programming Exercise 5.5
Instructions
abl
In Chapter 4, we developed an algorithm for converting from binary to decimal. You
can generalize this algorithm to work for a representation in any base. Instead of
using a power of 2, you will write a program that converts any base between 2 and
16. Recall that for numbers 10 - 15 we use letters A - F.
In convert.py, define a function named repToDecimal that expects two arguments,
a string, and an integer. The second argument should be the base. For example,
repToDecimal("10", 8) returns 8, whereas repToDecimal("10", 16)
returns 16.
• The function should use a lookup table to find the value of any digit. Make sure
that this table (it is actually a dictionary) is initialized before the function is
defined.
• For its keys, use the 10 decimal digits (all strings) and the letters A... F (all
uppercase). The value stored with each key should be the integer that the digit
represents. (The letter A associates with the integer value 10, and so on.)
• The main loop of the function should convert each digit to uppercase, look up its
value in the table, and use this value in the computation.
A main function that tests the conversion function with numbers in several bases
has been provided.
An example of main and correct output is shown below:
def main():
10
8
2
16
print (repToDecimal('10', 10))
print (repToDecimal('10', 8))
print (repToDecimal('10', 2))
print (repToDecimal('10', 16))
convert.py
+
1 # Put your code here
2
3 # A main for testing your program
4 def main():
5
6
7
"""Tests the function."▪▪
print (repToDecimal('10', 10))
print (repToDecimal('10', 8))
print (repToDecimal('10', 2))
print (repToDecimal('10', 16))
8
9
10
11 # The entry point for program execution
12 if __name__ == "_
"__main__":
13
main()
14
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
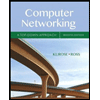
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
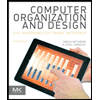
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
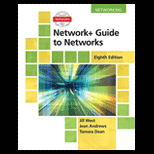
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
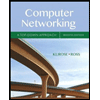
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
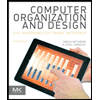
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
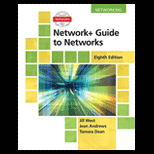
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
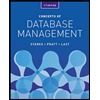
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
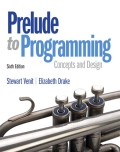
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
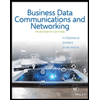
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY